Introduction
Within the programming realm, environment friendly knowledge interchange is a recurring necessity. JSON (JavaScript Object Notation) stands out as a well-liked format for knowledge interchange as a consequence of its human-readable nature and machine-friendly parsing capabilities. This complete information delves into various methodologies for changing a Python dictionary to JSON. Step-by-step examples are supplied to facilitate a radical understanding of the method.
Why Convert Python Dictionary to JSON?
Python dictionaries, a sturdy knowledge construction for key-value pairs, excel in knowledge manipulation. Nonetheless, JSON usually takes priority for knowledge sharing or storage as a consequence of its widespread help throughout numerous programming languages and platforms. The conversion ensures compatibility and clean integration with various methods.
Additionally Learn: Working with Lists & Dictionaries in Python
Strategies to Convert Python Dictionary to JSON
A number of approaches can be found in Python for changing a dictionary to JSON. Let’s discover every methodology intimately:
Utilizing the json Module
The built-in json module simplifies JSON knowledge dealing with in Python. It encompasses features and lessons for encoding and decoding JSON knowledge, facilitating seamless conversion between Python dictionaries and JSON.
Utilizing the json.dumps() Perform
The json.dumps() perform conveniently transforms a Python dictionary right into a JSON-formatted string. It permits customization, akin to specifying indentation and sorting, tailoring the conversion course of to particular wants.
Utilizing the json.dump() Perform
Just like json.dumps(), json.dump() converts a Python dictionary to a JSON string. Nonetheless, as a substitute of returning the string, it writes the JSON knowledge on to a file-like object, streamlining the method of saving JSON knowledge for later use.
Utilizing the json.JSONEncoder Class
The json.JSONEncoder class empowers customers to customise the JSON encoding course of. Subclassing this class and overriding its strategies present enhanced management over the conversion, particularly helpful for dealing with advanced knowledge varieties.
Utilizing Third-Occasion Libraries
Past the native json module, numerous third-party libraries like simplejson, ujson, and rapidjson prolong performance. These libraries provide superior options, improved efficiency, and enhanced error dealing with for working with JSON knowledge.
Dive into our free Python course to grasp changing Python dictionaries to JSON. Discover JSON Conversion Now!
Changing Python Dictionary to JSON: Step-by-Step Information
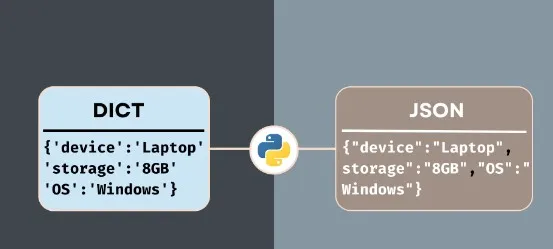
Now, let’s embark on an in depth step-by-step information to grasp the method totally:
Importing the json Module
import json
Making a Python Dictionary
knowledge = {
"title": "John Doe",
"age": 25,
"metropolis": "New York"
}
Step 3: Changing the Dictionary to JSON
json_data = json.dumps(knowledge)
Writing the JSON to a File
with open("knowledge.json", "w") as file:
json.dump(knowledge, file)
Step 5: Dealing with Errors and Exceptions
strive:
json_data = json.dumps(knowledge)
besides json.JSONDecodeError as e:
print("Error decoding JSON:", str(e))
Examples of Changing Python Dictionary to JSON
Let’s discover sensible examples as an instance the conversion course of in several situations:
Primary Conversion
knowledge = {
"title": "John Doe",
"age": 25,
"metropolis": "New York"
}
json_data = json.dumps(knowledge)
print(json_data)
Output:
{“title”: “John Doe”, “age”: 25, “metropolis”: “New York”}
Dealing with Nested Dictionaries
knowledge = {
"title": "John Doe",
"age": 25,
"deal with": {
"road": "123 Most important St",
"metropolis": "New York",
"state": "NY"
}
}
json_data = json.dumps(knowledge)
print(json_data)
Output:
{“title”: “John Doe”, “age”: 25, “deal with”: {“road”: “123 Most important St”, “metropolis”: “New York”, “state”: “NY”}}
Dealing with Lists and Tuples in Dictionaries
knowledge = {
"title": "John Doe",
"age": 25,
"hobbies": ["reading", "coding", "gaming"],
"scores": (90, 85, 95)
}
json_data = json.dumps(knowledge)
print(json_data)
Output:
{“title”: “John Doe”, “age”: 25, “hobbies”: [“reading”, “coding”, “gaming”], “scores”: [90, 85, 95]}
Dealing with Particular Information Sorts (e.g., datetime)
import datetime
knowledge = {
"title": "John Doe",
"age": 25,
"dob": datetime.date(1995, 5, 15)
}
# Customized JSON encoder for datetime objects
class DateTimeEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, datetime.date):
return obj.isoformat()
return tremendous().default(obj)
json_data = json.dumps(knowledge, cls=DateTimeEncoder)
print(json_data)
Output:
{“title”: “John Doe”, “age”: 25, “dob”: “1995-05-15”}
Greatest Practices for Changing Python Dictionary to JSON
To make sure a seamless and optimized conversion course of, adhere to the next greatest practices:
- Validate knowledge earlier than conversion to ensure it aligns with anticipated codecs. Deal with errors or inconsistencies preemptively.
- Protect Unicode characters by utilizing the ensure_ascii=False parameter in json.dumps(). This ensures correct encoding and decoding.
- Customise the JSON output’s format by using the indent parameter in json.dumps(). Regulate the variety of areas for indentation to reinforce readability.
- For big dictionaries or frequent conversions, think about leveraging third-party libraries like simplejson, ujson, or rapidjson for improved efficiency.
Frequent Points and Troubleshooting
Whereas changing Python dictionaries to JSON, be conscious of widespread points:
- JSON Serialization Errors: Tackle unsupported knowledge varieties or round references in dictionaries to stop JSON serialization errors. Guarantee all knowledge varieties are JSON serializable.
- Invalid JSON Output: Examine for syntax errors or lacking commas in dictionaries that will result in invalid JSON output. Make the most of on-line JSON validators to determine and rectify points.
- Dealing with Round References: Round references between objects in dictionaries could trigger infinite loops throughout JSON serialization. Handle round references by utilizing the json.JSONEncoder class and overriding its default() methodology.
Conclusion
This information covers various strategies for changing a Python dictionary to JSON. It emphasizes the importance of JSON for knowledge interchange, offering a radical understanding of conversion processes and greatest practices. Frequent points and troubleshooting methods are additionally addressed, making certain confidence in leveraging JSON for knowledge interchange in Python initiatives.
Incessantly Requested Questions
A. Changing a Python dictionary to JSON is crucial for efficient knowledge interchange. JSON, being a extensively supported format throughout numerous programming languages and platforms, ensures compatibility and seamless integration with various methods.
A. Errors will be dealt with utilizing attempt to besides blocks. For example, catching json.JSONDecodeError lets you handle errors through the decoding course of.
A. Sure, the method helps nested dictionaries. The json module seamlessly handles advanced knowledge buildings, making certain correct illustration in JSON format.
A. Sure, the method helps nested dictionaries. The json module seamlessly handles advanced knowledge buildings, making certain correct illustration in JSON format.