Introduction
Python, a flexible and highly effective programming language, comes with its distinctive challenges, and one which builders typically encounter is the AttributeError. On this complete information, we’ll delve into the varied kinds of attribute errors in Python and discover the most effective practices for dealing with them. Whether or not you’re a novice or a seasoned Python developer, this information is your companion to mastering the talent of managing attribute errors successfully.
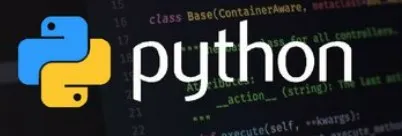
Forms of Attribute Errors
Forms of Attribute Errors: When working with Python, it’s possible you’ll encounter various attribute errors, every demanding a nuanced strategy. Understanding these errors is pivotal for crafting sturdy and error-resistant code. Let’s discover some prevalent kinds of attribute errors and find out how to deal with them.
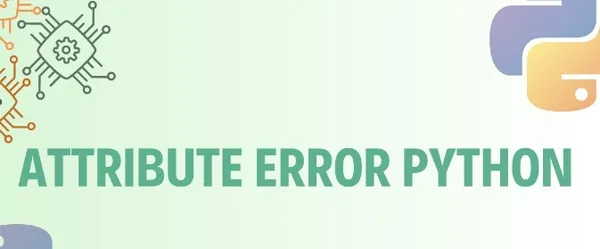
1. Accessing Nonexistent Attributes
Trying to entry an attribute that doesn’t exist for the article’s sort can result in AttributeErrors.
As an illustration:
marks_list = [90, 100, 95]
marks_list.append(92) # Appropriate utilization of append() as an alternative of add()
print(marks_list)
Output:
—————————————————————————
AttributeError Traceback (most up-to-date name final)
<ipython-input-20-9e43b1d2d17c> in <cell line: 2>()
1 marks_list = [90,100,95]
—-> 2 marks_list.add(92)
AttributeError: ‘checklist’ object has no attribute ‘add’
Rationalization:- Lists don’t have an add attribute so as to add a component; they’ve an append() technique.
Dealing with Above Error by utilizing append() technique
marks_list = [90,100,95]
marks_list.add(92)
print(marks_list)
Output:
[90, 100, 95, 92]
2. Typos or Misspelled Attributes
Incorrectly spelling the attribute title is a typical mistake that leads to AttributeErrors.
Instance:
my_str = "Hello, Himanshu."
print(my_str.low())
Output:
—————————————————————————
AttributeError Traceback (most up-to-date name final)
<ipython-input-29-47d1e641106e> in <cell line: 2>()
1 my_str=”Hello, Himanshu.”
—-> 2 print(my_str.low())
AttributeError: ‘str’ object has no attribute ‘low’
Rationalization:
The proper attribute is decrease().
Dealing with Above Error by utilizing decrease() technique
my_str="Hello, Himanshu."
print(my_str.decrease())
Output:
hello, himanshu.
3. Incorrect Object Kind
Anticipating an object to have attributes belonging to a distinct sort may end up in AttributeErrors.
For instance:
num = 42
num.higher()
Output:
—————————————————————————
AttributeError Traceback (most up-to-date name final)
<ipython-input-30-6782a28ddc25> in <cell line: 2>()
1 num = 42
—-> 2 num.higher()
AttributeError: ‘int’ object has no attribute ‘higher’
Rationalization:
The higher() technique is for strings, not numbers.
Dealing with Above Error by utilizing higher() technique with string
num = "My Marks : 42"
num.higher()
Output:
‘MY MARKS : 42’
Additionally Learn: Prime 31 Python Initiatives | Newbie to Superior (Up to date 2024)
Dealing with Attribute Errors in Object-Oriented Programming
Object-oriented programming (OOP) in Python introduces further nuances in dealing with attribute errors. Listed here are greatest practices for managing attribute errors in an OOP paradigm:
Pre-Test Existence:
Use hasattr(object, attribute_name) to confirm existence earlier than accessing.
if hasattr(object, attribute_name):
worth = object.attribute
Exception Dealing with:
Enclose attribute entry in try-except blocks to catch AttributeErrors gracefully:
strive:
worth = object.attribute
besides AttributeError:
# A compass for gracefully dealing with the error, like setting a default worth or logging a warning
Protected Attribute Entry:
Use getattr(object, attribute_name, default_value) for protected entry and defaults:
title = getattr(particular person, 'title', 'Unknown')
Additionally Learn: 7 Methods to Take away Duplicates from a Listing in Python
Greatest Practices for Dealing with Attribute Errors
Attribute errors will be difficult, however adopting greatest practices can result in extra dependable and maintainable code. Listed here are some pointers:
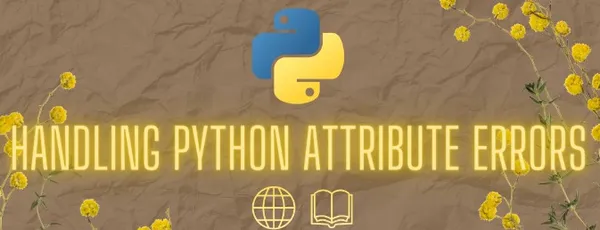
Prevention:
- Leverage sort hints and IDE autocompletion for early detection of potential errors.
- Doc anticipated attributes and strategies clearly to keep away from misinterpretations.
- Analyze and refactor code to reduce pointless attribute entry makes an attempt.
Pre-checking:
- Use hasattr(object, attribute_name) to verify the existence of an attribute earlier than trying to entry it. This observe eliminates the necessity for redundant error checks, enhancing code readability.
Exception dealing with:
- try-except Blocks: Safeguard attribute entry by enclosing it in try-except blocks. This ensures swish error dealing with. Provide clear error messages and handle the state of affairs appropriately, corresponding to setting a default worth or logging a warning.
- Particular Exceptions: Improve precision by catching particular AttributeError subtypes. This enables for extra nuanced and focused error dealing with.
Protected Entry:
- Use getattr(object, attribute_name, default_value) to securely entry attributes, offering a fallback worth if the attribute is absent. This strategy prevents dependence on doubtlessly incorrect assumptions concerning attribute existence.
Customized Attribute Dealing with:
- The __getattr__() and __getattribute__() strategies empower you to specify customized actions when an attribute is just not situated. This proves invaluable for providing various implementations or adeptly managing unexpected attributes.
Further Concerns
- Dynamic Attributes: Train warning when coping with dynamically generated attributes. Implement checks or safeguards to keep away from accessing non-existent ones.
- Duck Typing: Prioritize flexibility, however verify that objects adhere to the anticipated interface to sidestep runtime errors throughout technique calls.
- Testing: Rigorously check your code with various object sorts and situations to unveil any potential attribute-related points.
- Debugging: Make use of debugging instruments to hint the execution move and determine the origin of AttributeErrors.
Conclusion
In conclusion, mastering the artwork of dealing with attribute errors is essential for changing into a proficient Python developer. By understanding the several types of attribute errors and adopting efficient methods to deal with them, you possibly can craft extra sturdy and error-free code. Whether or not you’re a novice or an skilled developer, the ideas and methods mentioned on this information will empower you to change into a extra assured Python programmer.
Be part of our free Python course and effortlessly improve your programming prowess by mastering important sorting methods. Begin immediately for a journey of talent growth!
Steadily Requested Questions
A. AttributeError in Python happens when there may be an try and entry or modify an attribute that an object doesn’t possess or when there’s a mismatch in attribute utilization.
A. Forestall the error by checking if the attribute exists utilizing hasattr(object, attribute_name). Alternatively, make use of a try-except block to gracefully deal with the error and supply fallback mechanisms.
A. Frequent attribute errors embrace accessing nonexistent attributes, typos or misspelled attributes, and anticipating attributes from an incorrect object sort. Options contain pre-checking attribute existence, using try-except blocks, and making certain alignment with the right object sorts.
A. In OOP, attribute errors will be managed by pre-checking attribute existence with hasattr, utilizing try-except blocks for swish error dealing with, and using protected attribute entry with getattr(object, attribute_name, default_value).