Introduction
Variables are a necessary a part of any programming language, together with Python. They permit us to retailer and manipulate information inside our packages. In Python, variables can have totally different scopes, which decide their accessibility and visibility inside the program. On this article, we’ll discover the ideas of world and native variables in Python, perceive their variations, and talk about greatest practices for utilizing them successfully.
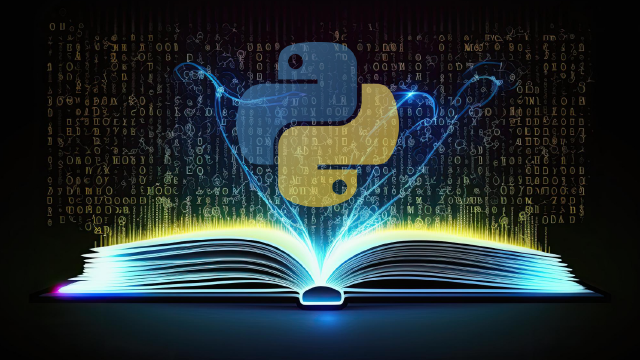
What are Variables in Python?
Variables in Python are used to retailer information values that may be accessed and manipulated all through this system. They act as containers for holding various kinds of information, corresponding to numbers, strings, or objects. In Python, variables are dynamically typed, which means that their sort can change throughout runtime.
Understanding Scope in Python
Scope refers back to the area of a program the place a variable is outlined and might be accessed. In Python, there are three sorts of variable scopes: international, native, and nonlocal.
World Variables
World variables are outlined exterior any operate or class and might be accessed from wherever inside the program. They’ve a worldwide scope, which means they’re seen to all capabilities and courses. World variables are helpful whenever you need to share information between totally different elements of your program.
Native Variables
Native variables are outlined inside a operate or a block of code and might solely be accessed inside that particular operate or block. They’ve a neighborhood scope, which means they’re solely seen inside the operate or block the place they’re outlined. Native variables are non permanent and are destroyed as soon as the operate or block of code is executed.
Nonlocal Variables
Nonlocal variables are utilized in nested capabilities, the place a operate is outlined inside one other operate. They’re neither international nor native variables. Nonlocal variables might be accessed and modified by the internal operate, in addition to the outer operate that encloses it.
Examples and Use Instances of World and Native Variables in Python
Let’s discover some examples and use circumstances to grasp the sensible purposes of world and native variables in Python.
World Variables in Python
depend = 0
def increment():
international depend
depend += 1
increment()
print(depend)
Output
1
On this instance, we’ve a worldwide variable `depend` that’s incremented contained in the `increment()` operate utilizing the `international` key phrase. The up to date worth of `depend` is then printed, which is 1.
Native Variables in Python
def calculate_sum(a, b):
consequence = a + b
return consequence
sum = calculate_sum(5, 10)
print(sum)
Output
15
On this instance, we’ve a neighborhood variable `consequence` that’s outlined inside the `calculate_sum()` operate. The operate takes two arguments, `a` and `b`, and calculates their sum. The result’s saved within the `consequence` variable, which is then returned and assigned to the worldwide variable `sum`. Lastly, the worth of `sum` is printed, which is 15.
Nonlocal Variables in Python
def outer_function():
x = 10
def inner_function():
nonlocal x
x += 5
print(x)
# Output: 15
inner_function()
outer_function()
On this instance, we’ve a nonlocal variable `x` that’s outlined within the `outer_function()`. The `inner_function()` is a nested operate that accesses and modifies the worth of `x` utilizing the `nonlocal` key phrase. The up to date worth of `x` is then printed, which is 15.
Variations Between World and Native Variables in Python
There are a number of variations between international and native variables in Python, together with their definition and declaration, entry and visibility, lifetime and reminiscence allocation, and modifiability and immutability.
Definition and Declaration
World variables are declared exterior any operate or class, whereas native variables are declared inside a operate or block of code. World variables might be accessed from wherever in this system, whereas native variables are solely accessible inside the operate or block the place they’re outlined.
Entry and Visibility
World variables have a wider scope and might be accessed from any a part of this system. Native variables, then again, have a restricted scope and might solely be accessed inside the operate or block the place they’re outlined.
Lifetime and Reminiscence Allocation
World variables have an extended lifetime as they’re created when this system begins and exist till this system terminates. They’re saved in a separate reminiscence location. Native variables, then again, have a shorter lifetime and are created when the operate or block of code is executed. They’re saved within the stack reminiscence.
Modifiability and Immutability
World variables might be modified all through this system, and their values might be modified a number of instances. Native variables, nonetheless, are non permanent and might solely be modified inside the operate or block the place they’re outlined.
Greatest Practices for Utilizing World and Native Variables in Python
To make sure clear and maintainable code, you will need to comply with some greatest practices when utilizing international and native variables in Python.
Avoiding Variable Title Conflicts
When utilizing international and native variables, it’s essential to decide on variable names which are distinctive and don’t battle with one another. This helps in avoiding surprising habits and makes the code extra readable.
Utilizing World Variables Sparingly:
World variables ought to be used sparingly as they’ll make the code tougher to grasp and debug. It’s endorsed to encapsulate code in capabilities and use native variables each time attainable.
Encapsulating Code in Capabilities
Encapsulating code in capabilities promotes code reusability and modularity. It permits for higher group of variables and reduces the possibilities of naming conflicts.
Utilizing Nonlocal Variables in Nested Capabilities
When working with nested capabilities, nonlocal variables can be utilized to entry and modify variables from the outer operate. This gives a method to share information between nested capabilities with out utilizing international variables.
Superior Matters and Strategies
Along with international and native variables in Python, there are some superior subjects and strategies associated to variable scope in Python.
World Variables in Modules
World variables might be outlined inside modules and accessed from totally different elements of this system. This permits for sharing information between a number of information and promotes code reusability.
World Variables in Lessons
In object-oriented programming, international variables might be outlined inside courses and accessed by totally different strategies of the category. This gives a method to retailer and share information throughout totally different situations of the category.
Dynamic Variable Scoping
Python helps dynamic variable scoping, which implies that the scope of a variable can change throughout runtime. This permits for extra flexibility in accessing and modifying variables based mostly on this system’s logic.
Variable Scope in Nested Capabilities
Nested capabilities in Python have entry to variables from the outer operate’s scope. This permits for information sharing and encapsulation of code inside nested capabilities.
Conclusion
Understanding the ideas of world and native variables in Python is essential for writing environment friendly and maintainable code. By following the most effective practices and avoiding widespread errors, you’ll be able to successfully use international and native variables to retailer and manipulate information inside your packages. Bear in mind to decide on variable names properly, encapsulate code in capabilities, and use international variables sparingly. With a stable understanding of variable scope in Python, you’ll be able to write cleaner and extra organized code.
Able to deepen your Python expertise and embark on an thrilling journey into the realms of Synthetic Intelligence and Machine Studying? Enroll in our Licensed AI & ML BlackBelt Plus Program and take your Python proficiency to the following stage. Achieve hands-on expertise, unlock superior ideas, and place your self as an AI and ML professional. Don’t miss this chance to turn out to be an authorized Python BlackBelt—join now and elevate your programming prowess! Be taught extra about Python by enrolling within the Licensed AI & ML BlackBelt Plus Program immediately.