Introduction
Indentation performs a vital function in Python programming. It’s a distinctive function of the language that units it aside from different programming languages. In Python, indentation is used to outline the construction and hierarchy of the code. It helps in visually organizing the code and making it extra readable. This text will discover the idea of indentation in Python, its significance, widespread errors, finest practices, and examples.
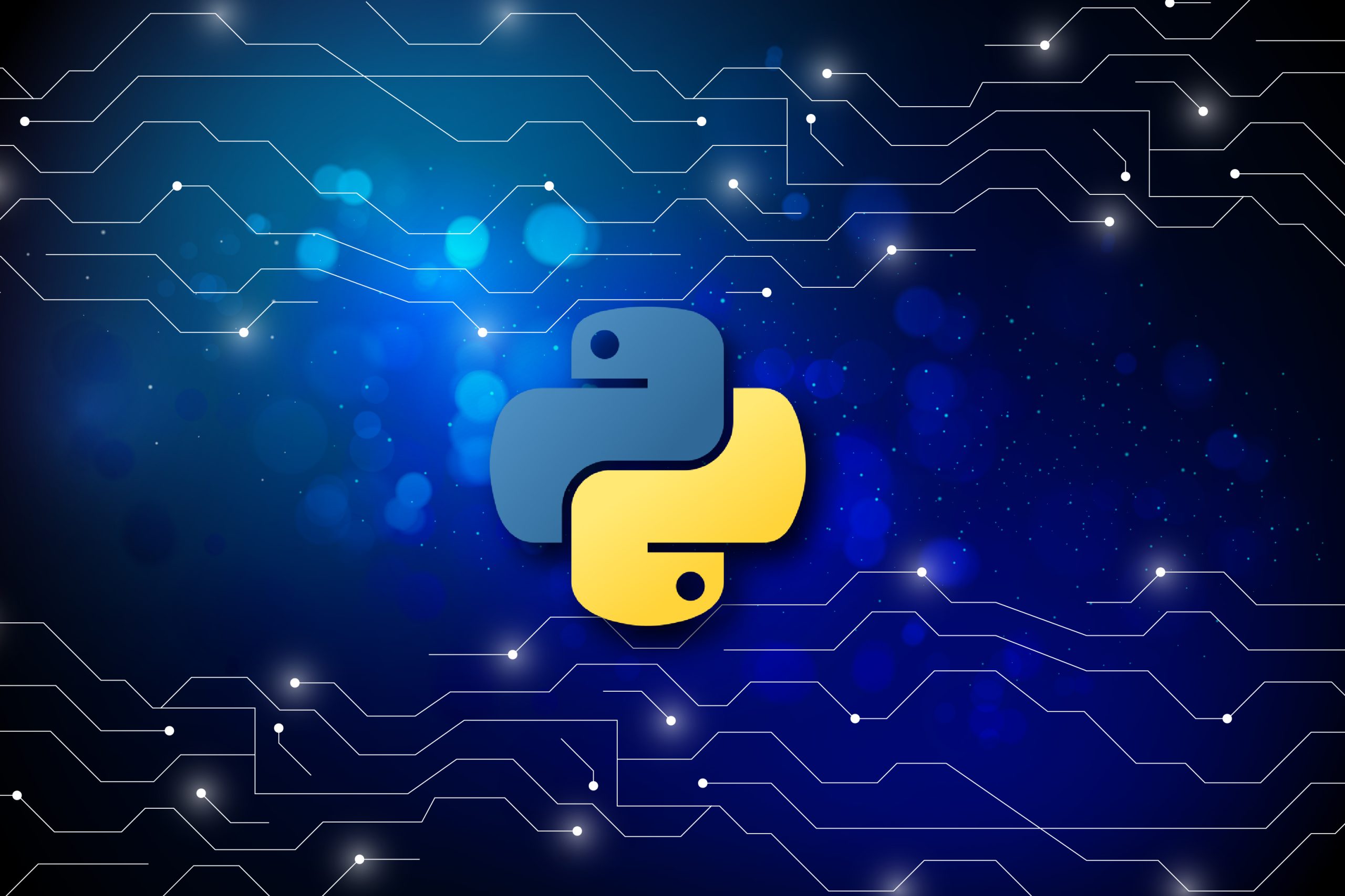
What’s Indentation in Python?
Indentation refers back to the areas or tabs which are used at the start of a line of code to point its stage of nesting inside a block. In Python, indentation isn’t just a matter of favor or desire, however it’s a syntactical requirement. In contrast to different programming languages that use braces or key phrases to outline blocks of code, Python makes use of indentation.
Elevate your expertise totally free! Enlist in our Introduction to Python Course and open doorways to a world of coding mastery.
Why is Indentation Essential in Python?
Indentation is necessary in Python for a number of causes. Firstly, it helps in defining the construction and hierarchy of the code. By visually representing the nesting of code blocks, indentation makes the code extra readable and comprehensible. It permits programmers to rapidly determine the start and finish of code blocks.
Secondly, indentation is essential for the right execution of the code. In Python, indentation is used to find out the scope of statements inside a block. Incorrect indentation can result in syntax errors or logical errors within the code. Subsequently, it’s important to comply with the indentation guidelines to make sure the code runs easily.
Additionally Learn: Tips on how to Convert Python Dictionary to Pandas DataFrame ?
Indentation Methods in Python
- Fundamental Indentation Guidelines: In Python, every line of code inside a block should be indented by the identical variety of areas or tabs. The usual conference is to make use of 4 areas for indentation. It is strongly recommended to keep away from utilizing tabs for indentation to stop mixing tabs and areas.
- Indentation Ranges and Nesting: Python makes use of indentation to point the extent of nesting inside a block. Every nested block ought to be indented additional than its mum or dad block. This helps in visually representing the hierarchy of code blocks.
- Indentation Width and Consistency: The width of indentation, i.e., the variety of areas or tabs used for indentation, is a matter of private desire. Nonetheless, it is very important be constant all through the codebase. PEP 8, the official type information for Python, recommends utilizing 4 areas for indentation.
- Mixing Tabs and Areas in Indentation: Mixing tabs and areas in indentation is taken into account unhealthy apply in Python. It might result in syntax errors and make the code tough to learn and preserve. It is strongly recommended to configure the textual content editor or IDE to make use of areas as an alternative of tabs for indentation.
Frequent Indentation Patterns and Examples
Indentation in Management Constructions (if-else, for, whereas)
Management buildings in Python, similar to if-else statements, for loops, and whereas loops, require correct indentation for proper execution. Right here’s an instance:
if situation:
# Indented block of code
statement1
statement2
else:
# Indented block of code
statement3
statement4
Indentation in Operate Definitions and Courses
Operate definitions and sophistication definitions additionally require correct indentation. Right here’s an instance:
def my_function():
# Indented block of code
statement1
statement2
class MyClass:
# Indented block of code
statement3
statement4
Indentation in Exception Dealing with (try-except)
Exception dealing with in Python makes use of indentation to outline the code block throughout the try to besides statements. Right here’s an instance:
attempt:
# Indented block of code
statement1
statement2
besides Exception:
# Indented block of code
statement3
statement4
Indentation in Record Comprehension and Generator Expressions
Record comprehension and generator expressions additionally require correct indentation. Right here’s an instance:
my_list = [x for x in range(10) if x % 2 == 0]
# Indented block of code
statement1
statement2
Additionally Learn: Understanding classmethod() in Python
Frequent Indentation Errors and Their Impression on Code Execution
Incorrect indentation can have a major influence on the execution of Python code. Listed here are some widespread indentation errors and their penalties:
- Lacking Indentation: Forgetting to indent code blocks may end up in a “IndentationError: anticipated an indented block” error. This error happens when Python expects an indented block after an announcement that requires one, similar to if statements or loops.
- Incorrect Indentation Ranges: Utilizing inconsistent or incorrect indentation ranges can result in syntax errors or logical errors within the code. It might trigger the code to behave unexpectedly or produce incorrect outcomes.
- Mixing Tabs and Areas in Indentation: Python is delicate to the usage of tabs and areas for indentation. Mixing tabs and areas may end up in an “IndentationError: inconsistent use of tabs and areas in indentation” error. It is strongly recommended to make use of both tabs or areas persistently all through the code.
- Inconsistent Indentation Fashion: Inconsistent indentation type, similar to utilizing completely different numbers of areas for indentation, could make the code tough to learn and preserve. You will need to comply with a constant indentation type to enhance code readability.
Indentation Greatest Practices for Readability and Maintainability
- Constant and Clear Indentation Ranges: Consistency is essential in relation to indentation. Use the identical variety of areas or tabs for indentation all through the codebase. Clear indentation ranges assist in understanding the construction of the code.
- Correct Use of Whitespace and Clean Strains: Correct use of whitespace and clean strains can improve code readability. Use clean strains to separate logical sections of code. Add whitespace round operators and after commas to enhance code readability.
- Breaking Lengthy Strains and Wrapping Indentation: When a line of code exceeds the really useful line size (often 79 or 80 characters), it’s advisable to interrupt it into a number of strains. Make sure that the indentation is constant throughout the damaged strains.
Conclusion
Indentation is a elementary side of Python programming. It helps in defining the construction and hierarchy of the code, making it extra readable and comprehensible. By following the most effective practices and avoiding widespread errors, you possibly can make sure that your code is correctly indented and executes easily. Bear in mind to make use of constant indentation, select the appropriate indentation type, and leverage instruments for automated code formatting.
Able to code? Start your Python journey with our Free Course.
Enroll now to find the great thing about programming!