Introduction
Picture resizing is a vital process in laptop imaginative and prescient that includes altering the size of a picture whereas sustaining its side ratio. It’s basic in varied functions, together with net improvement, laptop imaginative and prescient duties, and machine studying fashions. On this article, we are going to discover completely different image-resizing methods utilizing OpenCV, a well-liked library for laptop imaginative and prescient duties in Python.
Picture resizing performs a significant position in laptop imaginative and prescient functions. It permits us to regulate the scale of photographs to fulfill particular necessities, equivalent to becoming photographs into an online web page format, making ready photographs for machine studying fashions, or resizing photographs for laptop imaginative and prescient duties like object detection and recognition.
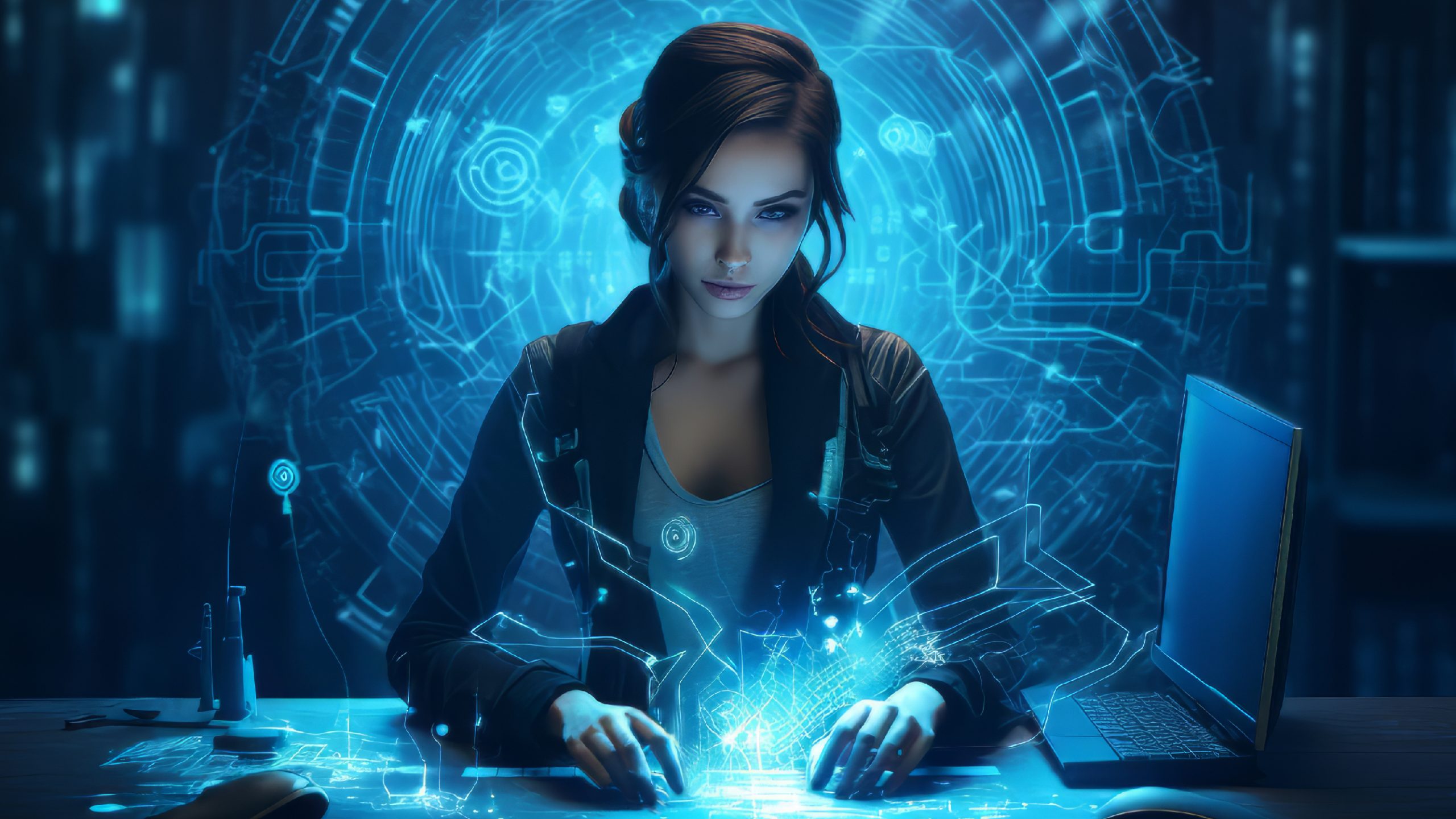
How-To: Picture Resizing in Python utilizing OpenCV
Comply with this step-by-step information to resize photographs effortlessly utilizing OpenCV in Python:
- Putting in OpenCV and Required Libraries.
Start by putting in the OpenCV library and any further required libraries. OpenCV might be put in utilizing bundle managers like pip.
- Loading and Displaying an Picture
Import the OpenCV library in your Python script and use the cv2.imread() perform to load a picture from a file. Show the loaded picture utilizing cv2.imshow(), and don’t overlook to incorporate cv2.waitKey(0) to make sure the picture window stays open till you press a key.
- Resizing an Picture
Make the most of the cv2.resize() perform to resize the loaded picture. Specify the specified dimensions (width and peak) as parameters.
- Saving the Resized Picture
After resizing the picture, put it aside to a brand new file utilizing the cv2.imwrite() perform. Present the filename and the resized picture as parameters.
Within the subsequent part, we’ll talk about this extra totally.
Picture Resizing Course of in Python utilizing OpenCV
Now, let’s dive into the sensible implementation of picture resizing utilizing OpenCV in Python. Comply with the steps under:
Putting in OpenCV and Required Libraries
Earlier than we start, be sure you have OpenCV put in in your system. You may set up it utilizing pip:
Code:
pip set up opencv-python
Moreover, it’s possible you’ll want to put in different libraries, equivalent to NumPy, for picture manipulation duties.
Loading and Displaying an Picture
To resize a picture, we first have to load it into our Python script. OpenCV supplies the `imread` perform to learn a picture from a file. We will then show the picture utilizing the `imshow` perform.
Code
import cv2
# Load the picture
picture = cv2.imread('picture.jpg')
# Show the picture
cv2.imshow('Authentic Picture', picture)
cv2.waitKey(0)
cv2.destroyAllWindows()
Resizing an Picture
To resize the picture, we will use OpenCV’s `resize` perform. We should specify the unique picture, goal dimensions, and interpolation methodology as enter parameters.
Code
# Resize the picture
resized_image = cv2.resize(picture, (new_width, new_height), interpolation=cv2.INTER_LINEAR)
Saving the Resized Picture
After resizing the picture, we will put it aside to a file utilizing the `imwrite` perform.
Code
# Save the resized picture
cv2.imwrite('resized_image.jpg', resized_image)
Picture Resizing Methods in OpenCV
OpenCV supplies a number of methods for resizing photographs, every serving completely different functions. Let’s discover a number of the generally used methods:
Resizing by Scaling
Resizing a picture by scaling includes multiplying the width and peak of the picture by a scaling issue. This system permits us to extend or lower the scale of a picture whereas sustaining its side ratio. OpenCV supplies the `resize` perform, which takes the unique picture and the specified dimensions as enter parameters.
Resizing with Side Ratio Preservation
Preserving the side ratio of a picture is essential to keep away from distortion. OpenCV supplies a handy methodology to resize photographs whereas preserving their side ratio. By specifying the specified width or peak, OpenCV mechanically adjusts the opposite dimension to take care of the side ratio.
Resizing with Customized Dimensions
In some circumstances, we might have to resize a picture to particular customized dimensions. OpenCV permits us to resize photographs to any desired width and peak by specifying the goal dimensions explicitly.
Resizing utilizing Interpolation Strategies
Interpolation strategies are used to estimate pixel values when resizing a picture. OpenCV supplies varied interpolation strategies, equivalent to nearest-neighbor, bilinear, and bicubic interpolation. These strategies assist in preserving picture high quality and decreasing artifacts throughout the resizing course of.
Frequent Challenges and Concerns in Picture Resizing
Whereas resizing photographs, we should contemplate sure challenges and preserve picture high quality. Let’s talk about some widespread challenges:
- Sustaining Picture High quality and Side Ratio: Sustaining picture high quality and side ratio is essential to keep away from distortion and artifacts. We will guarantee high-quality resized photographs utilizing applicable interpolation strategies and preserving the side ratio.
- Dealing with Completely different Picture Codecs: Photos might be in varied codecs, equivalent to JPEG, PNG, or BMP. Dealing with completely different picture codecs accurately throughout resizing is crucial to keep away from compatibility points.
- Coping with Reminiscence Constraints: Resizing massive photographs can eat important reminiscence. It’s important to optimize the resizing course of to deal with reminiscence constraints effectively, particularly when working with restricted assets.
Superior Picture Resizing Methods in OpenCV
OpenCV presents superior picture resizing methods that transcend easy scaling. Let’s discover a few of these methods:
Content material-Conscious Picture Resizing
Content material-aware picture resizing is a classy approach that goals to resize photographs whereas preserving necessary content material and constructions, adapting the resizing course of to the picture’s content material.
- Preservation of Content material: Not like conventional resizing strategies that will distort or crop necessary parts, content-aware resizing intelligently identifies and preserves areas of excessive significance within the picture.
- Seam Carving Algorithm: One of many fashionable approaches to content-aware resizing is the Seam Carving algorithm, which was briefly talked about within the article. Seam carving identifies and removes or provides seams (paths of pixels) with low significance, permitting for non-uniform resizing.
- Adaptive Resizing: Content material-aware resizing adapts the resizing operation based mostly on the picture’s content material. It could cut back the scale of much less necessary areas whereas sustaining the integrity of great objects or constructions.
- Purposes: Content material-aware resizing is especially helpful in eventualities the place preserving the content material and construction of the picture is essential. It finds functions in images, graphic design, and net improvement the place sustaining the visible integrity of photographs throughout resizing is necessary.
- Artifact Discount: Much like seam carving, content-aware resizing helps cut back artifacts that will happen in conventional resizing strategies, making certain a extra visually pleasing end result.
- Consumer Steerage: Some content-aware resizing instruments enable customers to information the resizing course of by specifying areas of the picture that ought to be preserved or eliminated. This interactive method supplies extra management over the ultimate end result.
- Limitations: Whereas content-aware resizing is highly effective, it might face challenges in sure photographs or advanced scenes. The effectiveness will depend on the algorithm used and the flexibility to precisely establish the significance of various picture areas.
Seam Carving for Picture Resizing
Seam carving is a complicated image-resizing approach that goes past conventional scaling. Not like conventional strategies that uniformly resize a picture, seam carving goals to intelligently resize photographs by eradicating or including seams, that are paths of pixels with low vitality.
- Power Map: The vitality of a pixel represents its significance within the picture. An vitality map is created by computing the gradient of the picture, highlighting areas with excessive distinction and necessary options.
- Dynamic Programming: Seam carving makes use of dynamic programming to seek out the optimum seam (path) to take away or duplicate within the picture. The seam with the bottom gathered vitality is taken into account, making certain that necessary options are preserved.
- Non-Uniform Resizing: Seam carving permits for non-uniform resizing, that means that completely different quantities can resize completely different picture elements. This permits the preservation of necessary particulars whereas resizing much less important areas.
- Artifact Discount: Seam carving helps cut back artifacts that will happen in conventional resizing strategies, particularly in photographs with advanced constructions or objects.
Tremendous-Decision Picture Resizing
Tremendous-resolution is a way that goals to reinforce the decision of a picture, producing high-frequency particulars that will not be current within the unique picture.
- Upsampling: Tremendous-resolution includes rising the spatial decision of a picture by upsampling, the place finer particulars are generated between current pixels.
- Studying-Based mostly Approaches: Fashionable super-resolution methods typically contain deep studying approaches. Convolutional Neural Networks (CNNs) are skilled to study the mapping between low-resolution and high-resolution picture pairs.
- Single Picture Tremendous-Decision (SISR): Some methods deal with enhancing the decision of a single picture with out counting on further high-resolution counterparts. These strategies use realized priors to generate believable high-resolution particulars.
- Purposes: Tremendous-resolution is especially helpful in functions the place high-quality photographs are required, equivalent to medical imaging, satellite tv for pc imagery, and surveillance.
- Commerce-offs: Whereas super-resolution can improve picture particulars, it’s important to notice that it can’t recuperate info that isn’t current within the unique low-resolution picture. The outcomes rely upon the standard of the coaching knowledge and the chosen super-resolution mannequin.
A number of libraries and instruments can be found for picture resizing. Let’s evaluate OpenCV with some fashionable options:
OpenCV vs PIL/Pillow
OpenCV and PIL/Pillow are extensively used libraries for image-processing duties. Whereas OpenCV focuses extra on laptop imaginative and prescient duties, PIL/Pillow supplies a broader vary of picture manipulation capabilities. The selection between the 2 will depend on the particular necessities of your mission.
Code
# PIL/Pillow for Picture Resizing
from PIL import Picture
# Load a picture from file
image_path="path/to/your/picture.jpg"
original_image_pillow = Picture.open(image_path)
# Set the specified width and peak
target_width = 300
target_height = 200
# Resize the picture utilizing PIL/Pillow
resized_image_pillow = original_image_pillow.resize((target_width, target_height))
# Show the unique and resized photographs utilizing PIL/Pillow
original_image_pillow.present(title="Authentic Picture")
resized_image_pillow.present(title="Resized Picture (Pillow)")
OpenCV vs scikit-image
Scikit-image is one other fashionable library for image-processing duties in Python. It supplies a complete set of picture resizing, filtering, and manipulation capabilities. OpenCV, alternatively, is extra specialised for laptop imaginative and prescient duties. The selection between the 2 will depend on the particular wants of your mission.
Code
# scikit-image for Picture Resizing
from skimage import io, rework
# Load a picture from file
image_path="path/to/your/picture.jpg"
original_image_skimage = io.imread(image_path)
# Set the specified width and peak
target_width = 300
target_height = 200
# Resize the picture utilizing scikit-image
resized_image_skimage = rework.resize(original_image_skimage, (target_height, target_width))
# Show the unique and resized photographs utilizing scikit-image
io.imshow(original_image_skimage)
io.title('Authentic Picture')
io.present()
io.imshow(resized_image_skimage)
io.title('Resized Picture (scikit-image)')
io.present()
OpenCV vs. ImageMagick
ImageMagick is a robust command-line device for picture manipulation. It supplies a variety of capabilities for resizing, cropping, and reworking photographs. Then again, OpenCV is a Python library that gives related performance and extra laptop imaginative and prescient capabilities. The selection between the 2 will depend on your most popular programming language and the complexity of your mission.
Code
# ImageMagick for Picture Resizing (utilizing subprocess)
import subprocess
# Set the paths
input_image_path="path/to/your/picture.jpg"
output_image_path="path/to/your/resized_image_magick.jpg"
# Set the specified width and peak
target_width = 300
target_height = 200
# Use ImageMagick command-line device for resizing
resize_command = f"convert {input_image_path} -resize {target_width}x{target_height} {output_image_path}"
subprocess.run(resize_command, shell=True)
# Word: Make sure that ImageMagick is put in in your system for the subprocess to work.
Picture Resizing for Particular Use Circumstances
Picture resizing serves completely different functions in varied functions. Let’s discover some particular use circumstances:
- Picture Resizing for Internet Purposes: In net improvement, picture resizing is crucial to optimize the loading time of net pages. By resizing photographs to the suitable dimensions, we will cut back the file dimension and enhance the general efficiency of net functions.
- Picture Resizing for Laptop Imaginative and prescient Duties: In laptop imaginative and prescient duties like object detection and recognition, resizing photographs to a selected dimension is usually vital. By resizing photographs to a constant dimension, we will be sure that the enter to our laptop imaginative and prescient fashions stays constant and correct.
- Picture Resizing for Machine Studying Fashions: Machine studying fashions typically require resizing photographs to a selected dimension earlier than coaching or inference. By resizing photographs to a constant dimension, we will guarantee compatibility with our machine-learning fashions and enhance their efficiency.
Conclusion
Picture resizing is a basic operation in laptop imaginative and prescient that permits us to regulate the size of photographs whereas sustaining their side ratio. OpenCV supplies varied methods and capabilities for picture resizing in Python. Following the steps outlined on this article, you possibly can resize photographs effectively for various functions. Contemplate the challenges and select the suitable methods based mostly in your particular necessities.
Elevate your expertise, achieve hands-on expertise, and change into a grasp in synthetic intelligence and machine studying. Be a part of now to entry unique coaching, mentorship, and certification. Rework your profession and embrace the way forward for expertise. Enroll within the Licensed AI & ML BlackBelt Plus Program right now and form the way forward for innovation!