Introduction
Python is a flexible programming language that provides numerous instruments and options to make coding extra environment friendly and arranged. One such characteristic is the classmethod() operate, which permits us to outline strategies which might be sure to the category somewhat than an occasion of the category. On this article, we’ll discover the idea of classmethod() in Python, its advantages, syntax, utilization, variations from staticmethod(), implementation examples, greatest practices, and customary errors to keep away from.
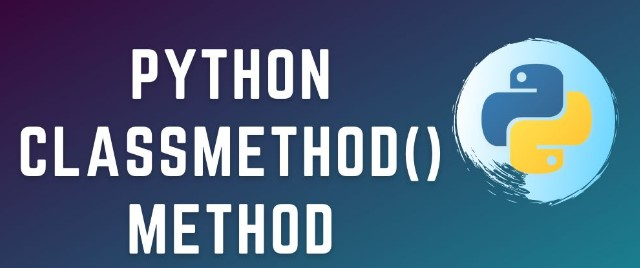
Study Introduction to Python Programming. Click on right here.
What’s a classmethod() in Python?
A classmethod() is a built-in operate in Python that’s used to outline a technique that’s sure to the category and never the occasion of the category. It’s denoted by the @classmethod decorator and may be accessed immediately from the category itself, with out the necessity for creating an occasion of the category.
Utilizing classmethod() affords a number of advantages in Python programming. Firstly, it permits us to outline strategies that may be accessed immediately from the category, making the code extra readable and arranged. Secondly, classmethod() gives a method to modify class attributes, which may be helpful in sure situations. Lastly, classmethod() permits us to implement inheritance extra effectively, as we’ll discover later on this information.
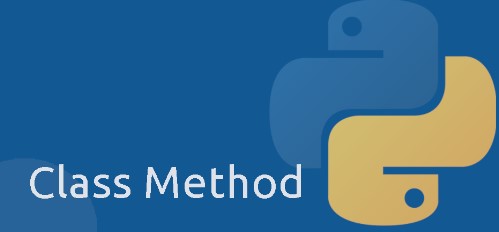
Syntax and Utilization of classmethod()
The syntax for outlining a classmethod() is as follows:
class MyClass:
@classmethod
def my_method(cls, arg1, arg2, ...):
# methodology implementation
Within the above syntax, `my_method` is the identify of the category methodology, and `arg1`, `arg2`, … are the arguments that the tactic can settle for. The `cls` parameter is robotically handed to the tactic and refers back to the class itself.
To make use of a classmethod(), we will immediately name it from the category, with out the necessity for creating an occasion of the category. For instance:
MyClass.my_method(arg1, arg2, ...)
Variations between classmethod() and staticmethod()
Though each classmethod() and staticmethod() are used to outline strategies which might be sure to the category somewhat than an occasion, there are some key variations between them.
- The primary distinction lies in the best way they deal with the primary parameter. In classmethod(), the primary parameter is robotically handed and refers back to the class itself (often named `cls`), whereas in staticmethod(), no parameters are robotically handed.
- One other distinction is that classmethod() can entry and modify class attributes, whereas staticmethod() can not. This makes classmethod() extra appropriate for situations the place we have to work with class-level information.
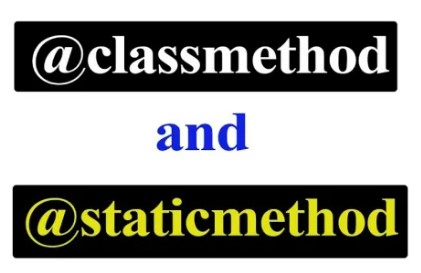
Examples of classmethod() Implementation
Let’s discover some examples to know how classmethod() may be carried out in Python.
Creating Class Strategies
class Circle:
pi = 3.14159
@classmethod
def calculate_area(cls, radius):
return cls.pi * radius * radius
# Calling the category methodology
space = Circle.calculate_area(5)
print("Space of the circle:", space)
Within the above instance, we outline a category methodology `calculate_area()` within the `Circle` class. This methodology calculates the realm of a circle utilizing the category attribute `pi` and the given radius. We will immediately name the category methodology utilizing the category identify, with out creating an occasion of the category.
Accessing Class Attributes and Strategies
class Rectangle:
size = 0
width = 0
def __init__(self, size, width):
self.size = size
self.width = width
@classmethod
def create_square(cls, aspect):
return cls(aspect, aspect)
# Making a sq. utilizing the category methodology
sq. = Rectangle.create_square(5)
print("Sq. size:", sq..size)
print("Sq. width:", sq..width)
On this instance, we outline a category methodology `create_square()` within the `Rectangle` class. This methodology creates a sq. by initializing the size and width with the identical worth. We will entry and modify the category attributes `size` and `width` utilizing the category methodology.
Modifying Class Attributes
class Counter:
depend = 0
def __init__(self):
Counter.depend += 1
@classmethod
def get_count(cls):
return cls.depend
# Creating situations of the category
c1 = Counter()
c2 = Counter()
c3 = Counter()
# Accessing the category attribute utilizing the category methodology
print("Rely:", Counter.get_count())
On this instance, we outline a category methodology `get_count()` within the `Counter` class. This methodology returns the worth of the category attribute `depend`, which retains monitor of the variety of situations created. We will entry the category attribute utilizing the category methodology, with out the necessity for creating an occasion.
Inheritance and classmethod()
class Animal:
legs = 4
@classmethod
def get_legs(cls):
return cls.legs
class Canine(Animal):
breed = "Labrador"
@classmethod
def get_breed(cls):
return cls.breed
# Accessing class attributes and strategies via inheritance
print("Variety of legs:", Canine.get_legs())
print("Breed:", Canine.get_breed())
On this instance, we outline a category methodology `get_legs()` within the `Animal` class, which returns the variety of legs. The `Canine` class inherits from the `Animal` class and defines its personal class methodology `get_breed()`, which returns the breed of the canine. We will entry each the category attributes and strategies via inheritance.
Future Consideration: Utilizing __class__
For readers searching for a deeper understanding, a substitute for the cls parameter is the usage of the __class__ attribute. Whereas cls is conventionally used and really helpful, understanding __class__ can present insights into the inside workings of Python.
The __class__ attribute refers back to the class of an occasion. When used inside a category methodology, it represents the category itself. Whereas this method is extra superior and never generally utilized in on a regular basis situations, exploring it will possibly deepen your grasp of Python’s mechanisms.
class Instance:
information = "Instance class"
@classmethod
def display_class_name(cls):
print("Class identify:", cls.__name__)
print("Utilizing __class__ attribute:", cls.__class__.__name__)
# Calling the category methodology
Instance.display_class_name()
On this instance, cls.__name__ and cls.__class__.__name__ each yield the category identify. Whereas cls.__name__ immediately accesses the category identify, cls.__class__.__name__ accesses the category identify through the __class__ attribute.
Remember that utilizing __class__ immediately is much less standard and could also be pointless in typical use instances. Nevertheless, it may be a helpful exploration for these fascinated by delving deeper into the Python language.
When to Use classmethod() in Python
classmethod() is helpful in numerous situations, corresponding to:
- When we have to outline strategies which might be sure to the category and never the occasion.
- Once we need to entry or modify class attributes.
- When implementing inheritance and must work with class-level information.
By utilizing classmethod(), we will make our code extra organized, readable, and environment friendly.
Widespread Errors and Pitfalls with classmethod()
Whereas utilizing classmethod(), there are some widespread errors and pitfalls to keep away from:
- Forgetting to make use of the @classmethod decorator earlier than defining the tactic.
- Not passing the `cls` parameter within the methodology definition.
- Unintentionally utilizing staticmethod() as an alternative of classmethod() or vice versa.
- Modifying mutable class attributes immediately with out utilizing the category methodology.
By being conscious of those errors, we will guarantee the correct utilization of classmethod() in our code.
Conclusion
On this article, we explored the idea of classmethod() in Python. We discovered about its advantages, syntax, utilization, variations from staticmethod(), and numerous examples of its implementation. We additionally mentioned when to make use of classmethod(), and customary errors to keep away from. By understanding and using classmethod() successfully, we will improve our Python programming abilities and create extra organized and environment friendly code.
You can even refer to those articles to know extra:
Regularly Requested Questions
A1: The primary goal of utilizing classmethod() in Python is to outline strategies which might be sure to the category somewhat than an occasion of the category. It permits direct entry from the category itself with out the necessity to create an occasion. classmethod() is especially helpful when working with class-level information, accessing or modifying class attributes, and implementing inheritance effectively.
A2: The important thing distinction between classmethod() and staticmethod() lies in how they deal with the primary parameter. In classmethod(), the primary parameter is robotically handed and refers back to the class itself (often named cls
), whereas staticmethod() doesn’t robotically cross any parameters. One other distinction is that classmethod() can entry and modify class attributes, making it appropriate for situations the place class-level information manipulation is required.
A3: No, classmethod() just isn’t usually used for creating situations of a category. Its main goal is to outline strategies that function on the category itself somewhat than situations. For creating situations, the usual constructor methodology __init__
is extra applicable.
A4: classmethod() is useful in inheritance because it permits youngster lessons to entry and make the most of class-level strategies and attributes outlined within the guardian class. This facilitates a extra environment friendly and arranged implementation of inheritance, enabling youngster lessons to inherit and prolong performance from the guardian class via class strategies.