Introduction
Python gives a variety of options and functionalities. Its clear and readable syntax, in depth normal library, and vibrant neighborhood of builders contribute to Python’s reputation and widespread adoption in numerous industries. One of many basic ideas in Python is scope, which determines the accessibility and visibility of variables inside a program. Understanding scope is essential for writing environment friendly and bug-free code. On this complete information, we are going to discover the totally different facets of scope in Python and the way it impacts the habits of variables.
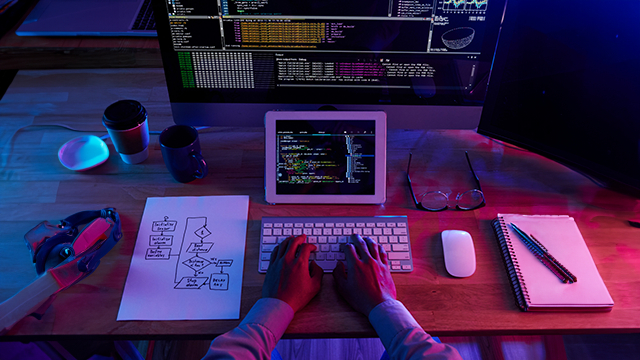
What’s Scope in Python?
Scope refers back to the area or context through which a variable is outlined and will be accessed. It defines the visibility and lifelong of a variable. In Python, there are three sorts of scope: world scope, native scope, and nonlocal scope.
You possibly can discover Python right here: Python
Significance of Understanding Scope in Python
Understanding scope is important for writing clear and maintainable code. It helps stop naming conflicts, improves code readability, and enhances reusability. By understanding the several types of scope in Python, builders can write extra environment friendly and bug-free applications.
World Scope
The worldwide scope refers to a program’s outermost scope. Variables outlined within the world scope are accessible from anyplace throughout the program and have a worldwide lifetime, that means they exist so long as this system is working.
Native Scope
Native scope refers back to the innermost scope of a program, resembling a operate or a block of code. Variables outlined within the native scope are solely accessible inside that particular scope. These variables have a neighborhood lifetime, that means they’re created when the scope is entered and destroyed when the scope is exited.
Nonlocal Scope
Nonlocal scope is a scope that’s neither world nor native. It’s used after we need to modify a variable in an outer (however non-global) scope from an internal scope. Nonlocal variables are accessible inside nested capabilities or internal scopes.
World, Native, and Nonlocal Variables in Python
World Variables
The World variables are outlined outdoors any operate or block of code. They’re accessible from anyplace throughout the program. To entry a worldwide variable inside a operate, we have to use the `world` key phrase.
Code
x = 10
def my_function():
world x
print(x)
my_function()
Output
10
Native Variables
Native variables are outlined inside a operate or block of code. They’re solely accessible inside that particular scope. Native variables can have the identical title as world variables, however they’re fully separate entities.
Code
x = 10
def my_function():
x = 20
print(x)
my_function()
print(x)
Output
20
10
Nonlocal Variables
Nonlocal variables are used to change variables in an outer (however non-global) scope from an internal scope. They’re accessible inside nested capabilities or internal scopes.
Code
def outer_function():
x = 10
def inner_function():
nonlocal x
x = 20
inner_function()
print(x)
outer_function()
Output
20
Additionally learn: Introduction to Python Programming (Newbie’s Information)
Scoping Guidelines in Python
LEGB Rule
Python follows the LEGB rule to find out the scope of a variable. LEGB stands for Native, Enclosing, World, and Constructed-in. When a variable is referenced, Python searches for it within the following order: native scope, enclosing scope, world scope, and built-in scope.
Code
x = 10
def my_function():
x = 20
def inner_function():
x = 30
print(x)
inner_function()
print(x)
my_function()
Output
30
20
Enclosing Scope
Enclosing scope refers back to the scope of the outer operate when there are nested capabilities. Interior capabilities can entry variables from the enclosing scope.
Code
def outer_function():
x = 10
def inner_function():
print(x)
inner_function()
outer_function()
Output
10
Nested Scopes
Nested scopes happen when there are a number of ranges of nested capabilities. Every nested operate has its personal scope, they usually can entry variables from outer scopes.
Code
def outer_function():
x = 10
def inner_function():
y = 20
def nested_function():
print(x + y)
nested_function()
inner_function()
outer_function()
Output
30
Scope and Features in Python
Operate Scope
In Python, capabilities create their very own native scope. Variables outlined inside a operate are solely accessible inside that operate.
Code
def my_function():
x = 10
print(x)
my_function()
print(x)
Output
10
Error: NameError: title ‘x’ shouldn’t be outlined
Operate Arguments and Scope
Operate arguments are additionally native variables throughout the operate’s scope. They’re created when the operate is named and destroyed when the operate completes its execution.
Code
def my_function(x):
print(x)
my_function(10)
print(x)
Output
10
Error: NameError: title ‘x’ shouldn’t be outlined
Returning Values from Features
Features can return values that may be assigned to variables within the calling scope. The returned values are accessible outdoors the operate.
Code
def add_numbers(a, b):
return a + b
outcome = add_numbers(10, 20)
print(outcome)
Output
30
Scope and Loops in Python
Loop Scope
In Python, variables outlined inside a loop have a neighborhood scope and are solely accessible inside that loop.
Code
for i in vary(5):
x = i * 2
print(x)
print(x)
Output
8
Scope Inside Loops In comparison with C/C++
In contrast to C/C++, Python doesn’t create a brand new scope for every iteration of a loop. Variables outlined inside a loop are accessible outdoors the loop.
Code
for i in vary(5):
x = i * 2
print(x)
Output
8
Scope and Modules in Python
Module Scope
Module scope refers back to the scope of variables outlined inside a module. These variables are accessible from any a part of the module.
Code
# module.py
x = 10
def my_function():
print(x)
# foremost.py
import module
print(module.x)
module.my_function()
Output
10
10
Importing and Utilizing Variables from Different Modules
Variables outlined in a single module will be imported and utilized in one other module utilizing the `import` assertion.
Code
# module1.py
x = 10
# module2.py
import module1
print(module1.x)
Output
10
Scope and Lessons in Python
Class Scope
Class scope refers back to the scope of variables outlined inside a category. These variables are accessible throughout the class and its cases.
Code
class MyClass:
x = 10
def my_method(self):
print(self.x)
my_object = MyClass()
print(my_object.x)
my_object.my_method()
Output:
10
Occasion Variables and Class Variables
Occasion variables are outlined inside strategies of a category and are distinctive to every occasion of the category. Class variables are outlined outdoors any methodology and are shared amongst all cases of the category.
Code
class MyClass:
class_variable = 10
def __init__(self, instance_variable):
self.instance_variable = instance_variable
object1 = MyClass(20)
object2 = MyClass(30)
print(object1.class_variable)
print(object2.class_variable)
print(object1.instance_variable)
print(object2.instance_variable)
Output
10
10
20
30
Scope and Exception Dealing with in Python
Scope of Exception Variables
Exception variables are native variables throughout the scope of an exception handler. They’re created when an exception is raised and destroyed when the exception is dealt with.
Code
attempt:
x = 10 / 0
besides ZeroDivisionError as e:
print(e)
print('a')
print(e)
Output
division by zero
a
Error: NameError: title ‘e’ shouldn’t be outlined
Dealing with Exceptions in Totally different Scopes
Exceptions will be dealt with at totally different ranges of scope, resembling inside a operate or on the world degree. The scope through which an exception is dealt with impacts the visibility of exception variables.
Code
def my_function():
attempt:
x = 10 / 0
besides ZeroDivisionError as e:
print(e)
my_function()
print(e)
Output
division by zero
Error: NameError: title ‘e’ shouldn’t be outlined
Conclusion
Understanding scope is essential for writing environment friendly and bug-free Python code. Builders can write clear and maintainable code by greedy the ideas of worldwide scope, native scope, and nonlocal scope. Scoping guidelines, operate scope, loop scope, module scope, class scope, and exception dealing with all play a major function in figuring out the visibility and accessibility of variables inside a program. By following the rules outlined on this complete information, builders can leverage the ability of scope in Python to write down strong and dependable purposes.
Elevate your abilities, advance your profession, and keep forward within the dynamic world of expertise with the Licensed AI & ML BlackBelt PlusProgram. Restricted spots can be found. Enroll now to safe your home and embark on a transformative journey into the way forward for synthetic intelligence and machine studying. Don’t miss out on this unique alternative! Click on right here to affix the elite ranks of Licensed AI & ML BlackBelts!