Introduction
Iterating over a dictionary in Python is a basic talent that permits you to effectively entry and manipulate key-value pairs inside this versatile information construction. By iterating by way of a dictionary, you possibly can carry out numerous operations, comparable to extracting values, updating data, or making use of particular logic to every key-value pair. This course of is essential for dealing with and processing information effectively in Python applications, offering a dynamic technique to work together with the contents of a dictionary. Within the following dialogue, we are going to discover totally different strategies and methods for iterating over dictionaries.
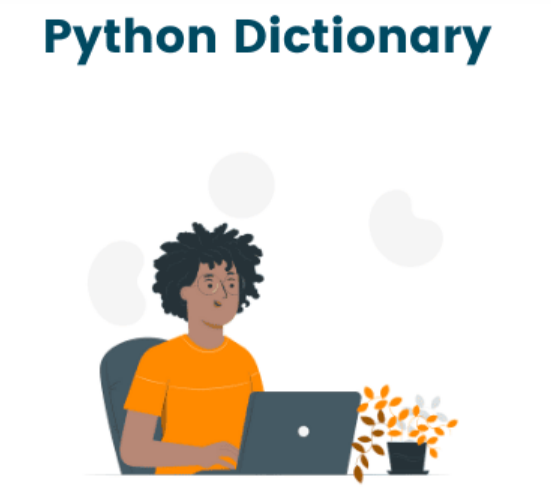
How you can Iterate Over a Dictionary in Python
Iterating by way of a dictionary means going by way of every key-value pair one after the other. It’s a vital activity if you wish to use a dictionary correctly in Python.
To do that, there are a number of generally used strategies for dictionary iteration:
- Use keys() to iterate by way of keys.
- Use .values() to undergo all values.
- Use objects() to iterate by way of key-value pairs.
- Make use of a for loop to loop by way of the dictionary.
- Entry keys utilizing map() and dict.get.
- Entry keys in Python utilizing zip().
- Entry keys by way of the unpacking of a dictionary.
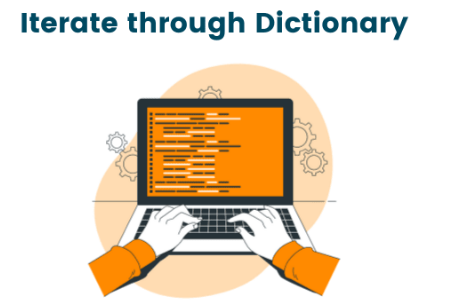
These strategies present numerous methods to navigate and work with the contents of a dictionary, providing you with flexibility in dealing with information in your Python applications.
Need to stage up your python abilities? Enroll in our Free Python Course!
Use keys() to iterate by way of keys
Utilizing the keys() methodology in Python permits you to iterate by way of the keys of a dictionary, offering a simple technique to entry and manipulate the dictionary’s construction. This methodology returns a view object that shows a listing of all of the keys within the dictionary.
Right here’s a easy instance demonstrating find out how to use keys() for dictionary iteration:
# Outline a pattern dictionary
fruit_prices = {'apple': 1.0, 'banana': 0.75, 'orange': 1.5, 'grape': 2.0}
# Iterate by way of keys utilizing keys()
for fruit in fruit_prices.keys():
print(f"The worth of {fruit} is {fruit_prices[fruit]} {dollars}.")
On this instance, we now have a dictionary fruit_prices with fruit names as keys and their corresponding costs as values. The keys() methodology is utilized within the for loop to iterate by way of every key (fruit) within the dictionary. Contained in the loop, we entry the corresponding worth utilizing the important thing and print the fruit together with its worth.
By utilizing keys(), you effectively traverse the keys of the dictionary, making it a handy methodology for duties that particularly contain working with the keys themselves.
Use .values() to undergo all values
Using the values() methodology in Python supplies a simple strategy to iterate by way of all of the values of a dictionary. This methodology returns a view object containing all of the values current within the dictionary, permitting you to entry and manipulate the information with out explicitly coping with the keys.
Let’s discover a sensible instance of find out how to use values() for dictionary iteration:
# Outline a pattern dictionary
fruit_prices = {'apple': 1.0, 'banana': 0.75, 'orange': 1.5, 'grape': 2.0}
# Iterate by way of values utilizing values()
for worth in fruit_prices.values():
print(f"The worth of a fruit is {worth} {dollars}.")
On this instance, the dictionary fruit_prices associates fruit names with their respective costs. The values() methodology is employed within the for loop to iterate by way of every worth within the dictionary. Contained in the loop, we print a press release that comes with the present worth.
By using values(), you possibly can effectively traverse by way of all of the values of the dictionary, making it notably helpful when your activity includes working with the values straight moderately than the related keys. This methodology enhances the pliability of dictionary manipulation in Python.
Use objects() to iterate by way of key-value pairs
The objects() methodology in Python is a robust software for iterating by way of key-value pairs in a dictionary. It supplies a handy technique to entry each the keys and their corresponding values concurrently, permitting for efficient manipulation of your entire dictionary construction.
Let’s delve right into a sensible instance to showcase find out how to use objects() for dictionary iteration:
# Outline a pattern dictionary
fruit_prices = {'apple': 1.0, 'banana': 0.75, 'orange': 1.5, 'grape': 2.0}
# Iterate by way of key-value pairs utilizing objects()
for fruit, worth in fruit_prices.objects():
print(f"The worth of {fruit} is {worth} {dollars}.")
On this instance, the dictionary fruit_prices incorporates fruit names as keys and their corresponding costs as values. The objects() methodology is employed within the for loop to iterate by way of every key-value pair. Contained in the loop, the variables fruit and worth are used to characterize the present key and its related worth, respectively. This permits us to print a press release that features each the fruit identify and its worth.
By utilizing objects(), you achieve a concise and environment friendly technique to navigate by way of your entire dictionary, extracting each keys and values as wanted. This methodology is especially helpful when your activity requires simultaneous entry to each parts of every key-value pair.
Make use of a for loop to loop by way of the dictionary
Utilizing a for loop in Python is a flexible and basic approach for iterating by way of a dictionary. This strategy supplies a simple technique of accessing every key within the dictionary, enabling efficient manipulation of the information saved inside.
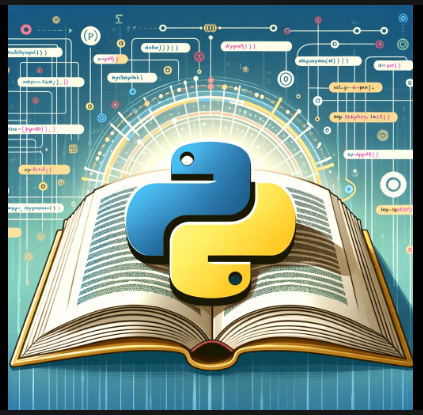
Let’s illustrate the utilization of a for loop to loop by way of a dictionary with a sensible instance:
# Outline a pattern dictionary
fruit_prices = {'apple': 1.0, 'banana': 0.75, 'orange': 1.5, 'grape': 2.0}
# Loop by way of the dictionary utilizing a for loop
for fruit in fruit_prices:
print(f"The worth of {fruit} is {fruit_prices[fruit]} {dollars}.")
On this instance, the dictionary fruit_prices incorporates fruit names as keys and their corresponding costs as values. The for loop is employed to iterate by way of every key within the dictionary. Contained in the loop, the variable fruit represents the present key, permitting us to entry the corresponding worth utilizing fruit_prices[fruit]. We then print a press release that features each the fruit identify and its worth.
Using a for loop on this method simplifies the method of iterating by way of a dictionary, offering a clear and environment friendly technique to entry and manipulate the information it holds. This system is especially helpful when you find yourself primarily desirous about working with the keys of the dictionary.
Entry keys utilizing map() and dict.get
In Python, you possibly can entry the keys of a dictionary utilizing the map() perform together with dict.get(). This mix supplies a concise and environment friendly technique to apply a perform to every key within the dictionary, producing a brand new iterable containing the outcomes.
Let’s illustrate this strategy with a sensible instance:
# Outline a pattern dictionary
fruit_prices = {'apple': 1.0, 'banana': 0.75, 'orange': 1.5, 'grape': 2.0}
# Use map() and dict.get() to entry keys
keys_result = map(fruit_prices.get, fruit_prices)
# Convert the consequence to a listing for visualization
keys_list = record(keys_result)
# Print the keys obtained utilizing map() and dict.get()
print("Keys obtained utilizing map() and dict.get():", keys_list)
On this instance, the map() perform is utilized to the fruit_prices.get methodology, which retrieves the worth for every key within the dictionary. The result’s then transformed into a listing for higher visualization. This record, keys_list, incorporates the keys of the fruit_prices dictionary.
Utilizing map() along with dict.get() is a robust strategy when it’s essential carry out an operation on every key of the dictionary and acquire the leads to a brand new iterable. It enhances the pliability of working with dictionaries in a concise and expressive method.
Entry keys in Python utilizing zip()
In Python, the zip() perform affords an environment friendly methodology to entry keys from a dictionary. By utilizing zip() along with the dictionary’s keys, you possibly can create pairs of keys and their corresponding values. This strategy supplies a handy technique to iterate by way of the keys with out straight accessing the dictionary.
Let’s dive right into a sensible instance to show find out how to entry keys utilizing zip():
# Outline a pattern dictionary
fruit_prices = {'apple': 1.0, 'banana': 0.75, 'orange': 1.5, 'grape': 2.0}
# Entry keys utilizing zip()
keys_result = record(zip(fruit_prices.keys()))
# Print the keys obtained utilizing zip()
print("Keys obtained utilizing zip():", keys_result)
On this instance, the zip() perform applies to fruit_prices.keys(), creating pairs of keys and their corresponding values. By changing the consequence into a listing, the keys_result variable incorporates the keys of the fruit_prices dictionary.
Utilizing zip() supplies a clear and concise technique to entry keys, and it’s particularly useful once you wish to work with key-value pairs as tuples. This methodology enhances the pliability of iterating by way of dictionary keys in a fashion that’s each readable and expressive.
Entry keys by way of the unpacking of a dictionary
In Python, you possibly can entry keys from a dictionary by way of the method of unpacking. This includes extracting the keys straight from the dictionary and dealing with them individually. Unpacking permits for a simple and environment friendly technique to entry and iterate by way of the keys with out explicitly calling any particular methodology.
Let’s discover a sensible instance for instance find out how to entry keys by way of the unpacking of a dictionary:
# Outline a pattern dictionary
fruit_prices = {'apple': 1.0, 'banana': 0.75, 'orange': 1.5, 'grape': 2.0}
# Entry keys by way of dictionary unpacking
keys_result = [*fruit_prices]
# Print the keys obtained by way of unpacking
print("Keys obtained by way of unpacking:", keys_result)
On this instance, the syntax [*fruit_prices] unpacks the dictionary, extracting all its keys. The ensuing keys_result incorporates a listing of the keys from the fruit_prices dictionary.
Unpacking supplies a concise and readable technique to entry keys, and it’s notably helpful once you desire a easy record of keys with out the necessity for added strategies or capabilities. This strategy enhances the readability and expressiveness of your code when working with dictionaries in Python.
Conclusion
In conclusion, figuring out find out how to undergo a dictionary in Python is essential for working with information. We’ve explored alternative ways, like utilizing keys(), values(), and objects(), or using methods comparable to for loops, map(), dict.get(), zip(), and unpacking.
These strategies present various methods to deal with dictionaries, permitting you to select the one that matches your wants finest. Studying find out how to iterate over dictionaries is a key talent that makes working with information in Python a lot simpler, whether or not you’re new to programming or already skilled. It’s like having a robust software to navigate and manipulate data successfully in your Python applications.
You possibly can learn extra articles associated to Python Dictionaries:
Ceaselessly Requested Questions
A: You need to use a for loop to iterate over the keys of a dictionary.
Instance:
my_dict = {‘a’: 1, ‘b’: 2}
for key in my_dict:
print(key)
A: The keys()
methodology can be utilized to get an iterable of keys for a dictionary.
Instance:
my_dict = {‘a’: 1, ‘b’: 2}
for key in my_dict.keys():
print(key)
A: You need to use a for loop to iterate over the values of a dictionary.
Instance:
my_dict = {‘a’: 1, ‘b’: 2}
for worth in my_dict.values():
print(worth)
A: Sure, the values()
methodology can be utilized to get an iterable of values for a dictionary.
Instance:
my_dict = {‘a’: 1, ‘b’: 2}
for worth in my_dict.values():
print(worth)
A: You need to use a for loop to iterate over the objects of a dictionary, which returns key-value pairs. Instance:
my_dict = {‘a’: 1, ‘b’: 2}
for key, worth in my_dict.objects():
print(f’Key: {key}, Worth: {worth}’)