Introduction
Python programming opens the door to a world of limitless potentialities, and one elementary job that always stands earlier than us is extracting distinctive values from an inventory. Getting distinctive values from an inventory is a standard job in Python programming. Similar to every line of code has its distinctive function, so do the weather in an inventory, and discerning the singular gems from the muddle of duplicates turns into a vital talent.
Distinctive values check with components in an inventory that happen solely as soon as, with out duplicates. On this article, you’ll study A-Z about varied strategies for acquiring distinctive values from an inventory and focus on their significance in numerous situations.
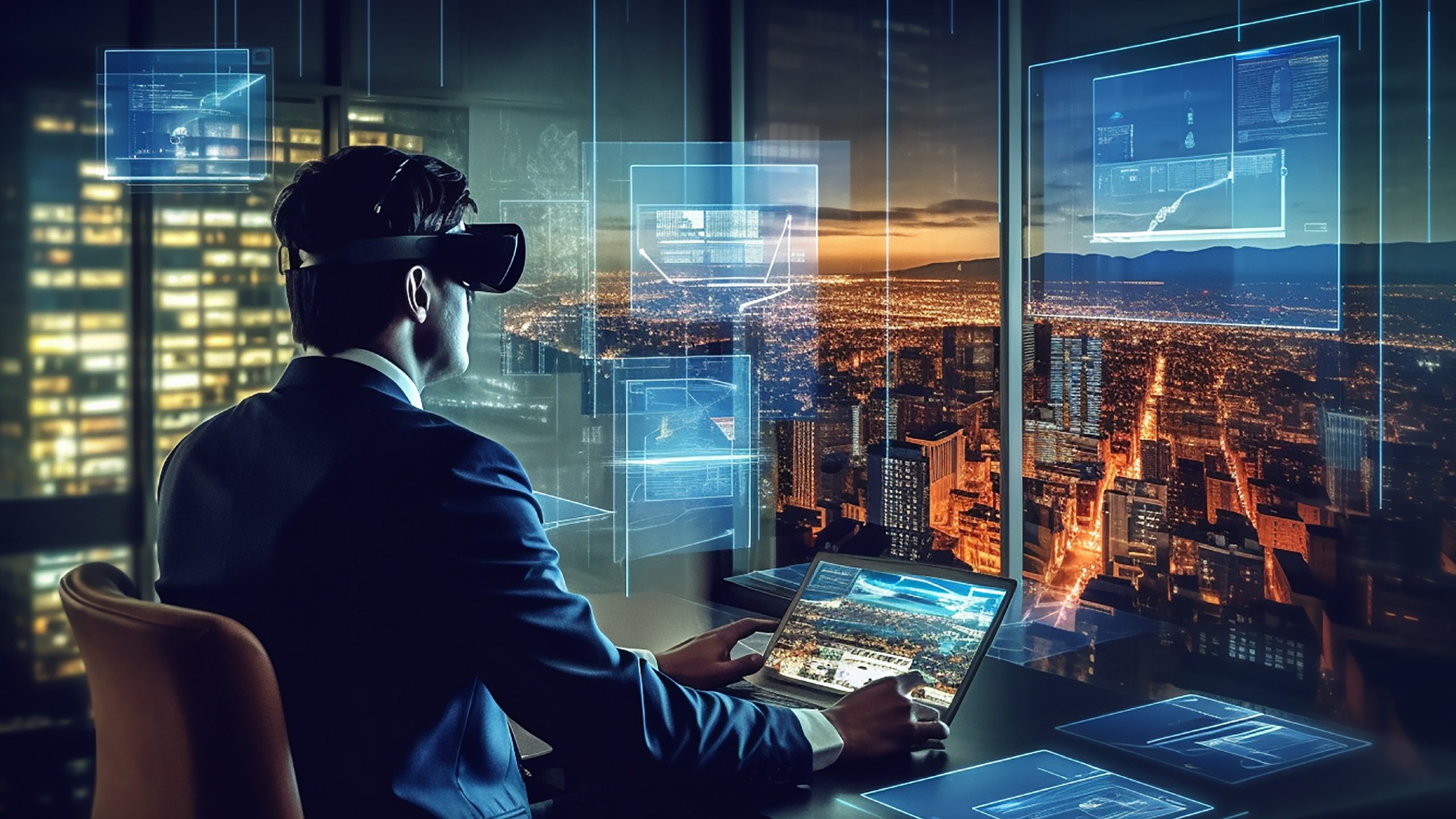
Why is Getting Distinctive Values Vital?
Acquiring distinctive values from an inventory is essential in lots of programming duties. It permits us to eradicate duplicate entries, simplify knowledge evaluation, and enhance the effectivity of our code. Whether or not working with giant datasets, performing statistical evaluation, or manipulating knowledge constructions, having distinctive values can present correct and significant outcomes.
Strategies to Get Distinctive Values from a Record Utilizing Python
Utilizing the set() Operate
Python’s set() perform is a robust instrument to acquire distinctive values from an inventory. It routinely removes duplicates and returns a set object containing solely the distinctive components. We are able to then convert this set again into an inventory if wanted.
Instance
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = listing(set(my_list))
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Utilizing Record Comprehension
Record comprehension is one other concise and environment friendly strategy to get distinctive values from an inventory. We are able to filter out duplicates and acquire solely the distinctive values by iterating over the listing and checking if a component is already current in a brand new listing.
Instance
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = [x for i, x in enumerate(my_list) if x not in my_list[:i]]
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Utilizing the dict.fromkeys() Technique
The dict.fromkeys() methodology can get distinctive values from an inventory by making a dictionary with the listing components as keys. Since dictionaries can’t have duplicate keys, this methodology routinely removes duplicates and returns an inventory of distinctive values.
Instance
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = listing(dict.fromkeys(my_list))
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Utilizing the Counter() Operate
The Counter() perform from the collections module is a robust instrument for counting the occurrences of components in an inventory. We are able to acquire the distinctive values from the unique listing by changing the Counter object into an inventory.
Instance
from collections import Counter
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = listing(Counter(my_list))
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Utilizing the Pandas Library
The Pandas library supplies a complete set of information manipulation and evaluation instruments. It provides a novel() perform for acquiring distinctive values from an inventory or a pandas Sequence object.
Instance
import pandas as pd
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = pd.Sequence(my_list).distinctive().tolist()
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Additionally learn: 15 Important Python Record Capabilities & The right way to Use Them (Up to date 2024)
Comparability of Strategies
Now, let’s evaluate the above strategies primarily based on their efficiency, reminiscence utilization, and dealing with of mutable and immutable components.
Efficiency
Concerning efficiency, the set() perform and listing comprehension methodology are the quickest methods to acquire distinctive values from an inventory. They’ve a time complexity of O(n), the place n is the size of the listing. The dict.fromkeys() methodology and Counter() perform even have a time complexity of O(n), however they contain further steps that make them barely slower. The Pandas library, whereas highly effective for knowledge evaluation, is relatively slower because of its overhead.
Reminiscence Utilization
When it comes to reminiscence utilization, the set() perform and listing comprehension methodology are memory-efficient as they eradicate duplicates instantly from the listing. The dict.fromkeys() methodology and Counter() perform create further knowledge constructions, which can devour extra reminiscence. As a complete instrument, the Pandas library requires further reminiscence for its knowledge constructions and operations.
Dealing with Mutable and Immutable Parts
All of the strategies mentioned above work properly with each mutable and immutable components. Whether or not the listing incorporates integers, strings, tuples, or customized objects, these strategies can deal with them successfully and supply distinctive values accordingly.
You may also learn: Python Record Packages For Absolute Freshmen
Examples of Getting Distinctive Values from a Record in Python
Let’s discover a number of extra examples to grasp get distinctive values from an inventory in numerous situations.
Instance 1: Getting Distinctive Values from a Record of Tuples
We are able to use listing comprehension if our listing incorporates tuples and we wish to acquire distinctive values primarily based on a particular component of every tuple.
my_list = [(1, 'a'), (2, 'b'), (3, 'a'), (4, 'c'), (5, 'b')]
unique_values = [x for i, x in enumerate(my_list) if x[1] not in [y[1] for y in my_list[:i]]]
print(unique_values)
Output
[(1, ‘a’), (2, ‘b’), (4, ‘c’)]
Instance 2: Discovering Distinctive Values in a Nested Record
If our listing is nested, and we wish to acquire distinctive values throughout all ranges, we are able to use the itertools library to flatten the listing after which apply the specified methodology.
import itertools
my_list = [[1, 2, 3], [2, 3, 4], [3, 4, 5]]
flattened_list = listing(itertools.chain.from_iterable(my_list))
unique_values = listing(set(flattened_list))
print(unique_values)
Output
[1, 2, 3, 4, 5]
Suggestions and Tips for Effectively Getting Distinctive Values
Sorting the Record Earlier than Eradicating Duplicates
If the order of distinctive values shouldn’t be essential, sorting the listing earlier than eradicating duplicates can enhance efficiency. It’s because sorting brings comparable components collectively, making figuring out and eradicating duplicates simpler.
Utilizing the setdefault() Technique for Nested Lists
When working with nested lists, the setdefault() methodology can be utilized to acquire distinctive values effectively. It permits us to create a dictionary with the weather as keys and their occurrences as values. We are able to acquire the distinctive values by changing the dictionary keys again into an inventory.
Utilizing the itertools Library for Superior Operations
The itertools library supplies highly effective instruments for superior operations on lists, together with acquiring distinctive values. Capabilities like chain(), groupby(), and combos() can be utilized to control and extract distinctive values from complicated knowledge constructions.
Conclusion
On this article, we explored varied strategies to get distinctive values from an inventory in Python. We mentioned the significance of acquiring distinctive values and in contrast totally different strategies primarily based on their efficiency, reminiscence utilization, and dealing with of mutable and immutable components. We additionally supplied examples and suggestions for effectively getting distinctive values. By understanding these strategies and their functions, you’ll be able to improve your Python programming abilities and enhance the effectivity of your code.