Introduction
Checking if a component exists in an inventory is frequent in Python programming. A number of strategies can be found to perform this, whether or not you need to seek for a quantity, string, or perhaps a customized object. This text gives you methods to test if a component exists in an inventory and focus on its efficiency issues. We may also present examples and greatest practices that can assist you write environment friendly, readable code.
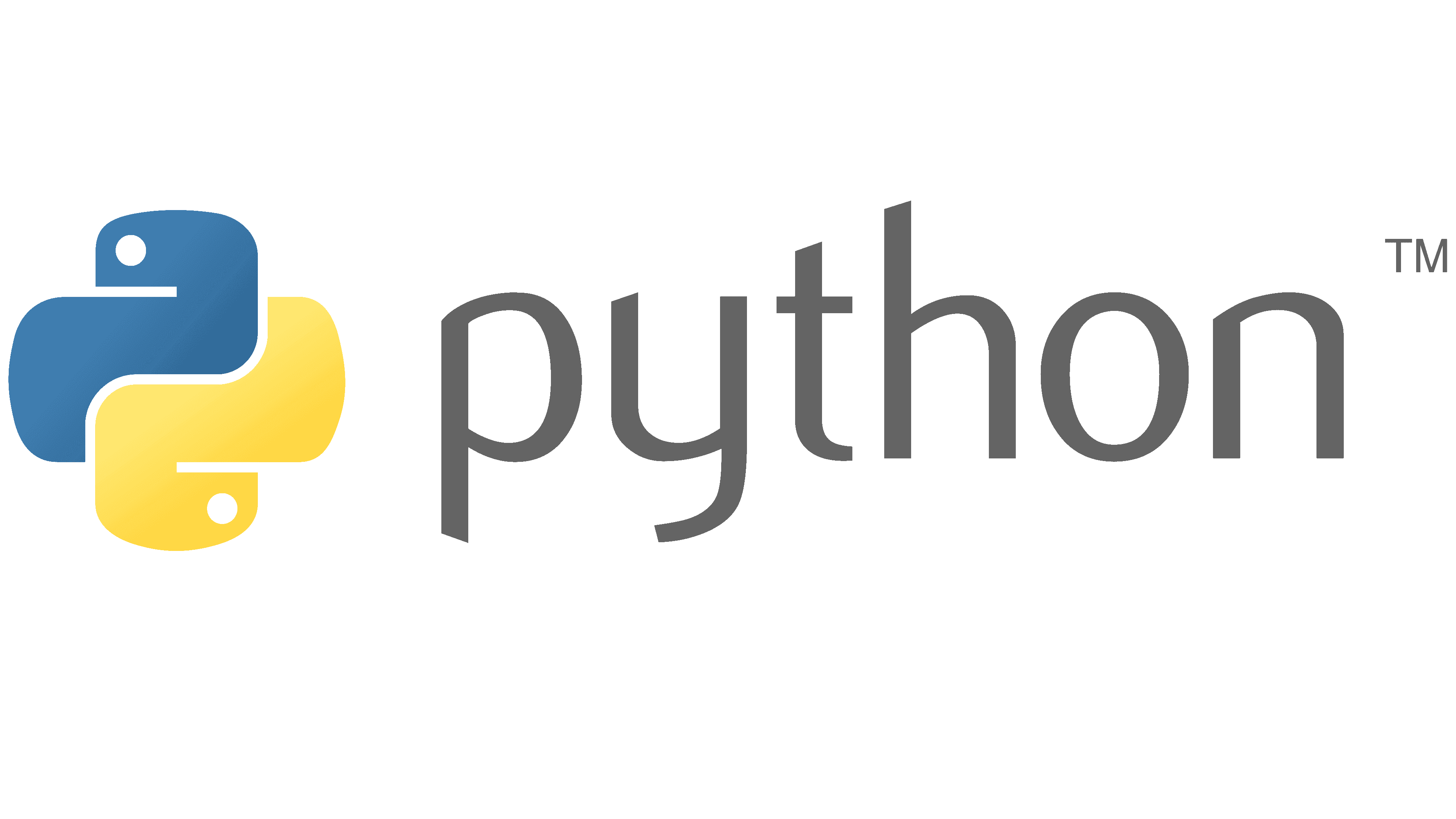
Strategies to Test if Aspect Exists in Listing
Utilizing the ‘in’ Operator
Essentially the most easy strategy to test if a component exists in an inventory is through the use of the ‘in’ operator. This operator returns True if the aspect is discovered within the checklist and False in any other case. Right here’s an instance:
numbers = [1, 2, 3, 4, 5]
if 3 in numbers:
print("Aspect discovered!")
else:
print("Aspect not discovered.")
Output
Aspect discovered!
Utilizing the ‘not in’ Operator
Equally, you should utilize the ‘not in’ operator to test if a component doesn’t exist in an inventory. This operator returns True if the aspect shouldn’t be discovered and False in any other case. Right here’s an instance:
fruits = ['apple', 'banana', 'orange']
if 'grape' not in fruits:
print("Aspect not discovered.")
else:
print("Aspect discovered!")
Output
Aspect not discovered.
Utilizing the ‘rely()’ Methodology
The ‘rely()’ methodology lets you rely the occurrences of a component in an inventory. By checking if the rely is bigger than zero, you possibly can decide if the aspect exists within the checklist. Right here’s an instance:
colours = ['red', 'blue', 'green', 'blue', 'yellow']
if colours.rely('blue') > 0:
print("Aspect discovered!")
else:
print("Aspect not discovered.")
Output
Aspect discovered!
Utilizing the ‘index()’ Methodology
The ‘index()’ methodology returns the index of the primary prevalence of a component in an inventory. If the aspect shouldn’t be discovered, it raises a ValueError. By dealing with the exception, you should utilize this methodology to test if a component exists. Right here’s an instance:
names = ['Alice', 'Bob', 'Charlie']
attempt:
index = names.index('Dave')
print("Aspect discovered at index", index)
besides ValueError:
print("Aspect not discovered.")
Output
Aspect not discovered.
Utilizing the ‘any()’ Perform
The ‘any()’ operate returns True if any aspect in an iterable is True. By passing an inventory comprehension that checks for the aspect, you possibly can decide if it exists within the checklist. Right here’s an instance:
numbers = [1, 2, 3, 4, 5]
if any(num == 3 for num in numbers):
print("Aspect discovered!")
else:
print("Aspect not discovered.")
Output
Aspect discovered!
Utilizing Listing Comprehension
Listing comprehension offers a concise strategy to create lists based mostly on present lists. By utilizing checklist comprehension, you possibly can create a brand new checklist that comprises True or False values indicating the existence of the aspect. Right here’s an instance:
numbers = [1, 2, 3, 4, 5]
exists = [num == 3 for num in numbers]
if True in exists:
print("Aspect discovered!")
else:
print("Aspect not discovered.")
Output
Aspect discovered!
Efficiency Concerns
When checking if a component exists in an inventory, it is very important take into account the efficiency implications of every methodology. Let’s analyze the time and house complexity of the totally different methods.
Time Complexity Evaluation
- Utilizing the ‘in’ operator, ‘not in’ operator, and ‘rely()’ methodology have a time complexity of O(n), the place n is the size of the checklist. It is because they should iterate by the whole checklist to find out the existence of the aspect.
- Utilizing the ‘index()’ methodology has a time complexity of O(n) within the worst case, as it might have to iterate by the whole checklist. Nonetheless, the common case has a time complexity of O(1) because it immediately accesses the aspect on the specified index.
- The ‘any()’ operate and checklist comprehension even have an O(n) time complexity as they iterate by the checklist to test for the aspect.
Area Complexity Evaluation
All of the strategies talked about above have an area complexity of O(1) aside from checklist comprehension. Listing comprehension creates a brand new checklist, which requires extra house proportional to the size of the unique checklist.
Greatest Practices for Checking if Aspect Exists in Listing
To make sure environment friendly and readable code, listed below are some greatest practices to comply with when checking if a component exists in an inventory in Python:
Utilizing Acceptable Knowledge Buildings
If you happen to steadily have to test for the existence of parts, think about using a set as an alternative of an inventory. Units present fixed time complexity for checking if a component exists, making them extra environment friendly for this job.
Writing Readable and Maintainable Code
Use significant variable names and feedback to make your code extra readable. Moreover, take into account encapsulating the checking logic in a separate operate to enhance code maintainability and reusability.
Conclusion
Checking if a component exists in an inventory is a basic operation in Python programming. By utilizing the ‘in’ operator, ‘not in’ operator, ‘rely()’ methodology, ‘index()’ methodology, ‘any()’ operate, or checklist comprehension, you possibly can simply decide the existence of a component. Nonetheless, it is very important take into account the efficiency implications and comply with greatest practices to jot down environment friendly and readable code.
Unlock the ability of information with our “Introduction to Python” course! Dive into the basics of Python programming tailor-made for knowledge science. Achieve hands-on expertise and remodel uncooked knowledge into actionable insights. Elevate your profession by mastering Python, a vital ability within the data-driven world. Enroll now for a brighter future!