Introduction
When working with knowledge in Python, it’s typically helpful to arrange data in a structured method. One such knowledge construction that may be significantly useful is a dictionary of lists. On this article, we are going to discover what a dictionary of lists is, the advantages of utilizing it, and numerous methods to create and manipulate it in Python.
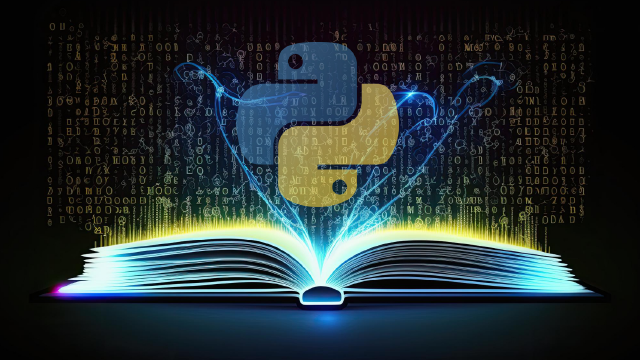
What’s a Dictionary of Lists?
A dictionary of lists is a knowledge construction in Python that permits you to retailer a number of values for a single key. It’s much like an everyday dictionary, the place every key’s related to a price, however on this case, the worth is a listing. This implies you can have a number of components for every key, making it a flexible and highly effective instrument for organizing and manipulating knowledge.
Advantages of Utilizing a Dictionary of Lists
There are a number of advantages to utilizing a dictionary of lists:
Flexibility: With a dictionary of lists, you’ll be able to retailer a number of values for a single key, permitting you to characterize complicated relationships between knowledge components.
Straightforward Entry: You possibly can simply entry and modify particular lists or components inside the dictionary utilizing the important thing.
Environment friendly Sorting: Sorting the lists inside the dictionary turns into simple, as you’ll be able to apply sorting algorithms on to the lists.
Simplified Knowledge Manipulation: Manipulating and performing operations on the info turns into extra intuitive and environment friendly with a dictionary of lists.
Making a Dictionary of Lists in Python
There are a number of methods to create a dictionary of lists in Python. Let’s discover a number of the most typical strategies:
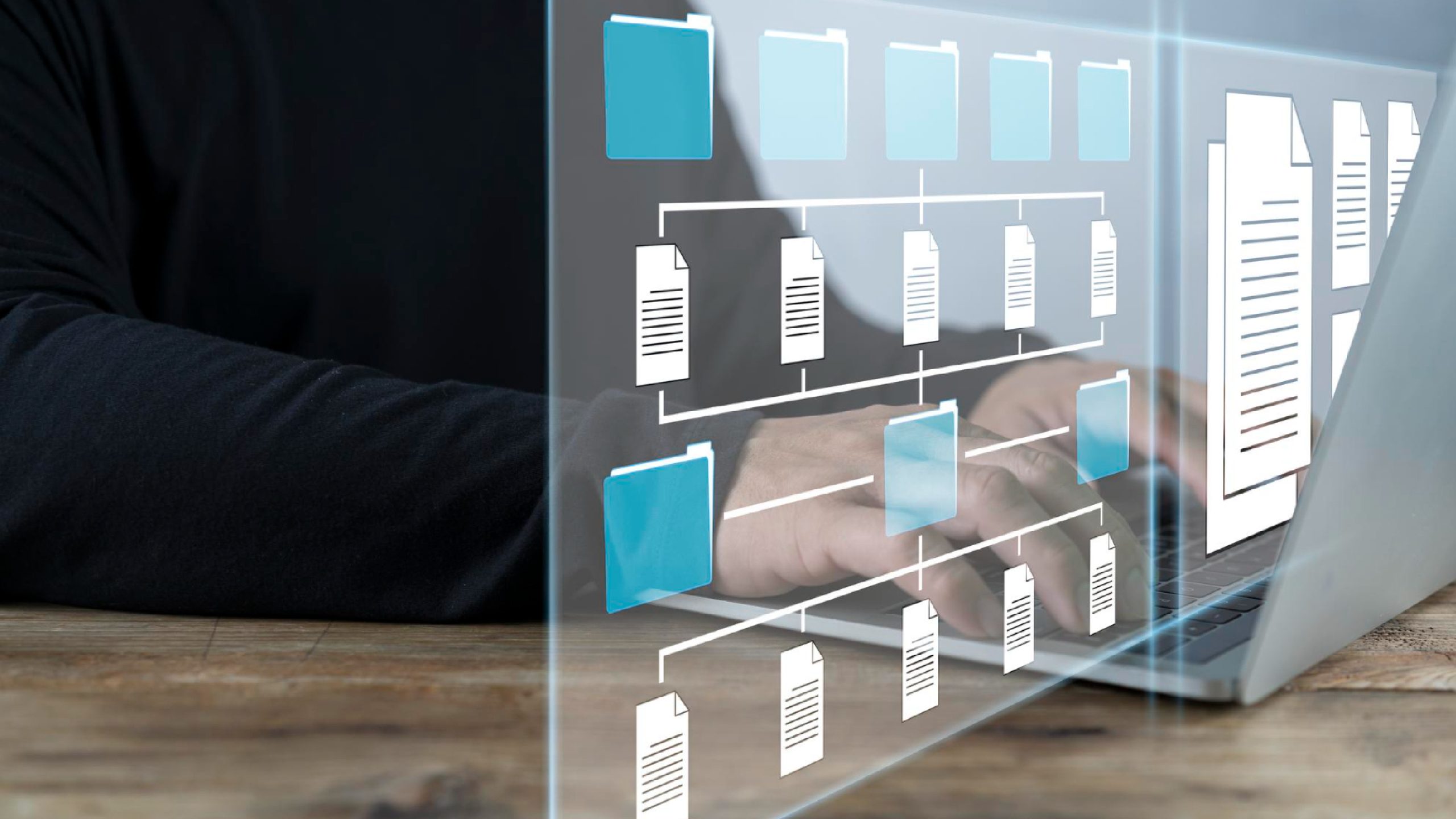
Utilizing the zip() Perform
The zip() operate can be utilized to mix two lists right into a dictionary of lists. Right here’s an instance:
keys = ['name', 'age', 'city']
values = ['John', 25, 'New York']
dictionary = dict(zip(keys, [[value] for worth in values]))
print(dictionary)
Output:
{‘title’: [‘John’], ‘age’: [25], ‘metropolis’: [‘New York’]}
On this instance, we use a listing comprehension to create a brand new checklist for every worth within the `values` checklist. The `zip()` operate then combines the `keys` and the brand new lists to create the dictionary of lists.
Utilizing a Loop and Checklist Comprehension
One other strategy to create a dictionary of lists is through the use of a loop and checklist comprehension. Right here’s an instance:
keys = ['name', 'age', 'city']
values = ['John', 25, 'New York']
dictionary = {key: [value] for key, worth in zip(keys, values)}
print(dictionary)
Ouput:
{‘title’: [‘John’], ‘age’: [25], ‘metropolis’: [‘New York’]}
On this instance, we iterate over the `keys` and `values` concurrently utilizing the `zip()` operate. We then use a dictionary comprehension to create the dictionary of lists.
Utilizing the defaultdict() Perform
The `defaultdict()` operate from the `collections` module can be used to create a dictionary of lists. Right here’s an instance:
from collections import defaultdict
dictionary = defaultdict(checklist)
dictionary['name'].append('John')
dictionary['age'].append(25)
dictionary['city'].append('New York')
On this instance, we create a `defaultdict` object with the `checklist` kind because the default manufacturing unit. This enables us to append values to the lists straight utilizing the keys.
Changing a Checklist to a Dictionary of Lists
If you have already got a listing of key-value pairs, you’ll be able to convert it right into a dictionary of lists utilizing the `setdefault()` methodology. Right here’s an instance:
knowledge = [('name', 'John'), ('age', 25), ('city', 'New York')]
dictionary = {}
for key, worth in knowledge:
dictionary.setdefault(key, []).append(worth)
On this instance, we iterate over the `knowledge` checklist and use the `setdefault()` methodology to create a brand new checklist for every key if it doesn’t exist. We then append the corresponding worth to the checklist.
Accessing and Modifying Values in a Dictionary of Lists
After getting created a dictionary of lists, you’ll be able to simply entry and modify its values. Listed below are some widespread operations:
Accessing a Particular Checklist within the Dictionary
To entry a particular checklist within the dictionary, you need to use the important thing because the index. For instance:
dictionary = {'title': ['John'], 'age': [25], 'metropolis': ['New York']}
name_list = dictionary['name']
print(name_list)
Output:
[‘John’]
On this instance, we entry the checklist related to the important thing `’title’` and assign it to the variable `name_list`.
Accessing an Component in a Particular Checklist
To entry a component in a particular checklist, you need to use the important thing to entry the checklist after which use the index to entry the factor. For instance:
dictionary = {'title': ['John'], 'age': [25], 'metropolis': ['New York']}
title = dictionary['name'][0]
print(title)
Output:
[‘John’]
On this instance, we entry the primary factor within the checklist related to the important thing `’title’` and assign it to the variable `title`.
Modifying a Checklist within the Dictionary
To switch a listing within the dictionary, you’ll be able to entry the checklist utilizing the important thing after which use checklist strategies or task to switch the weather. For instance:
dictionary = {'title': ['John'], 'age': [25], 'metropolis': ['New York']}
dictionary['name'].append('Doe')
print(dictionary)
Output:
{‘title’: [‘John’, ‘Doe’], ‘age’: [25], ‘metropolis’: [‘New York’]}
On this instance, we append the string `’Doe’` to the checklist related to the important thing `’title’`.
Including and Eradicating Components in a Checklist
So as to add or take away components in a listing inside the dictionary, you need to use checklist strategies akin to `append()`, `lengthen()`, `insert()`, `take away()`, or `pop()`. For instance:
dictionary = {'title': ['John'], 'age': [25], 'metropolis': ['New York']}
dictionary['name'].append('Doe')
dictionary['age'].take away(25)
On this instance, we append the string `’Doe’` to the checklist related to the important thing `’title’` and take away the worth `25` from the checklist related to the important thing `’age’`.
Widespread Operations and Manipulations with a Dictionary of Lists
A dictionary of lists supplies numerous operations and manipulations that may be carried out on the info. Let’s discover some widespread ones:
Sorting the Lists within the Dictionary
To kind the lists inside the dictionary, you need to use the `sorted()` operate or the `kind()` methodology. For instance:
dictionary = {'title': ['John', 'Alice', 'Bob'], 'age': [25, 30, 20]}
dictionary['name'].kind()
sorted_age = sorted(dictionary['age'])
print(sorted_age)
Output:
[20, 25, 30]
On this instance, we kind the checklist related to the important thing `’title’` in ascending order utilizing the `kind()` methodology. We additionally use the `sorted()` operate to create a brand new sorted checklist from the checklist related to the important thing `’age’`.
Merging A number of Lists within the Dictionary
To merge a number of lists inside the dictionary, you need to use the `lengthen()` methodology. For instance:
dictionary = {'title': ['John'], 'age': [25], 'metropolis': ['New York']}
dictionary['name'].lengthen(['Alice', 'Bob'])
On this instance, we lengthen the checklist related to the important thing `’title’` by including the weather `’Alice’` and `’Bob’`.
Filtering and Trying to find Values within the Lists
To filter or seek for particular values within the lists inside the dictionary, you need to use checklist comprehensions or built-in capabilities akin to `filter()` or `index()`. For instance:
dictionary = {'title': ['John', 'Alice', 'Bob'], 'age': [25, 30, 20]}
filtered_names = [name for name in dictionary['name'] if title.startswith('A')]
index_of_bob = dictionary['name'].index('Bob')
On this instance, we use a listing comprehension to filter the names that begin with the letter `’A’` from the checklist related to the important thing `’title’`. We additionally use the `index()` methodology to search out the index of the worth `’Bob’` in the identical checklist.
Counting and Summing Components within the Lists
To rely or sum the weather within the lists inside the dictionary, you need to use the `len()` operate or the `sum()` operate. For instance:
dictionary = {'title': ['John', 'Alice', 'Bob'], 'age': [25, 30, 20]}
count_names = len(dictionary['name'])
sum_age = sum(dictionary['age'])
On this instance, we use the `len()` operate to rely the variety of names within the checklist related to the important thing `’title’`. We additionally use the `sum()` operate to calculate the sum of the ages within the checklist related to the important thing `’age’`.
Ideas and Tips for Working with a Dictionary of Lists
Listed below are some ideas and tips to reinforce your expertise when working with a dictionary of lists:
Effectively Initializing an Empty Dictionary of Lists
To effectively initialize an empty dictionary of lists, you need to use the `defaultdict()` operate from the `collections` module. For instance:
from collections import defaultdict
dictionary = defaultdict(checklist)
On this instance, we create a `defaultdict` object with the `checklist` kind because the default manufacturing unit. This enables us to append values to the lists straight utilizing the keys with out explicitly initializing them.
Dealing with Empty Lists within the Dictionary
When working with a dictionary of lists, it is very important deal with circumstances the place a listing is empty. You need to use conditional statements or the `if` assertion to verify if a listing is empty earlier than performing operations on it. For instance:
dictionary = {'title': [], 'age': [25], 'metropolis': ['New York']}
if dictionary['name']:
# Carry out operations on the non-empty checklist
move
else:
# Deal with the case when the checklist is empty
move
On this instance, we verify if the checklist related to the important thing `’title’` is empty earlier than performing any operations on it.
Avoiding Duplicate Values within the Lists
To keep away from duplicate values within the lists inside the dictionary, you need to use the `set()` operate to transform the checklist to a set after which again to a listing. For instance:
dictionary = {'title': ['John', 'Alice', 'Bob', 'John'], 'age': [25, 30, 20, 25]}
dictionary['name'] = checklist(set(dictionary['name']))
On this instance, we convert the checklist related to the important thing `’title’` to a set, which routinely removes duplicate values. We then convert the set again to a listing and assign it to the identical key.
Conclusion
On this article, now we have explored the idea of a dictionary of lists in Python. We’ve got realized about its advantages, numerous strategies to create and manipulate it, and a few ideas and tips to reinforce our expertise when working with it. By using a dictionary of lists, we will effectively manage and manipulate knowledge in a structured method, making our code extra readable and maintainable.
You possibly can enroll in our free Python Course right this moment!
Continuously Requested Questions
A. A dictionary of lists is a knowledge construction the place every key’s related to a listing of values. It permits storing a number of values for a single key, offering flexibility in organizing and manipulating knowledge.
A. You possibly can create a dictionary of lists utilizing strategies like zip()
, loop and checklist comprehension, defaultdict()
, or changing a listing to a dictionary of lists utilizing setdefault()
.
A. Accessing includes utilizing keys to retrieve particular lists or components, whereas modification might be performed by checklist strategies or direct task.
A. Widespread operations embrace sorting lists, merging a number of lists, filtering/looking for values, and counting/summing components inside lists.