Introduction
Matplotlib.pyplot.subplots() is a strong operate in Python that enables customers to create a grid of subplots inside a single determine. This operate is part of the Matplotlib library, which is broadly used for knowledge visualization in Python. With subplots(), customers can simply create a number of plots in a single determine, making evaluating and analyzing totally different datasets or variables simpler.
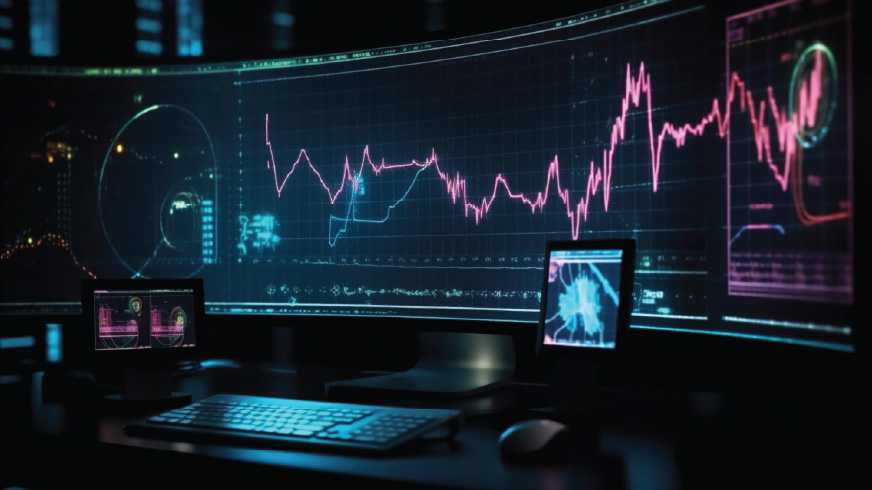
Advantages of Utilizing Matplotlib.pyplot.subplots()
Utilizing Matplotlib.pyplot.subplots() provide a number of advantages for knowledge visualization duties. Firstly, it gives a handy solution to arrange and show a number of plots in a single determine. This may be notably helpful when evaluating totally different points of a dataset or when presenting numerous visualizations.
Moreover, subplots() enable customers to customise the format and association of the subplots. Customers can specify the variety of rows and columns within the subplot grid and the dimensions and spacing between every subplot. This flexibility permits customers to create visually interesting and informative plots.
Additionally Learn: Introduction to Matplotlib utilizing Python for Novices
Understanding the Subplots Grid
The subplot grid is the association of subplots inside a determine created utilizing Matplotlib.pyplot.subplots(). It consists of rows and columns, every cell representing a subplot. The consumer can specify consumer can choose the variety of rows and columns relying on the specified format.
The subplots are accessed utilizing indexing, much like accessing components in a matrix. For instance, to entry the subplot within the first row and second columns, the indexing could be [0, 1]. This permits customers to change and customise particular person subplots inside the grid rapidly.
The subplot grid might be created utilizing the subplots() operate, which returns a Determine object and an array of Axes objects. The Determine object represents your complete determine, whereas the Axes objects characterize every subplot. These Axes objects can be utilized to change the properties of every subplot, such because the title, labels, and knowledge.
Making a Fundamental Subplot Grid
This part will discover methods to create a fundamental subplot grid utilizing the `matplotlib.pyplot.subplots()` operate in Python. This operate permits us to create a grid of subplots inside a single determine, making it simpler to visualise a number of plots concurrently.
Syntax and Parameters
The syntax for making a subplot grid is as follows:
Code:
fig, axes = plt.subplots(nrows, ncols)
Right here, `nrows` and `ncols` characterize the variety of rows and columns within the subplot grid. The operate returns two objects: fig,
which implies your complete determine and axes,
which is an array of axes objects representing every subplot.
Instance: Making a 2×2 Subplot Grid
Let’s think about an instance to know methods to create a 2×2 subplot grid. First, we have to import the mandatory libraries:
Code:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 2*np.pi, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
y4 = np.exp(x)
fig, axes = plt.subplots(2, 2)
axes[0, 0].plot(x, y1)
axes[0, 0].set_title('Sin(x)')
axes[0, 1].plot(x, y2)
axes[0, 1].set_title('Cos(x)')
axes[1, 0].plot(x, y3)
axes[1, 0].set_title('Tan(x)')
axes[1, 1].plot(x, y4)
axes[1, 1].set_title('Exp(x)')
plt.tight_layout()
plt.present()
Output:
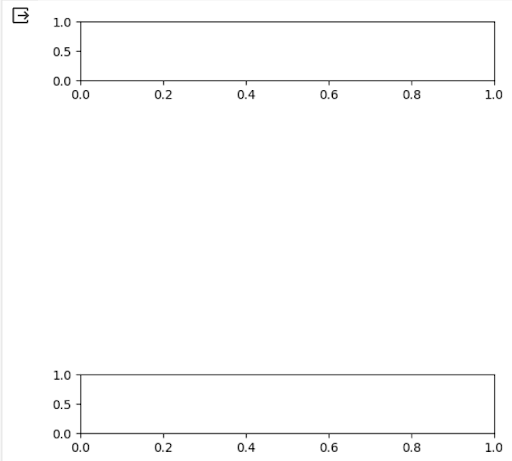
On this instance, we create a 2×2 subplot grid utilizing the `subplots()` operate. We then entry every subplot utilizing indexing on the `axes` array and plot our knowledge accordingly. Lastly, we use `tight_layout()` to regulate the spacing between subplots and `present()` to show the determine.
Through the use of the `matplotlib.pyplot.subplots()` operate, we will simply create and customise subplot grids in Python. This permits us to visualise a number of plots in a single determine, making knowledge evaluation and presentation handy.
Adjusting Subplot Spacing
You’ll be able to alter the spacing between them when creating subplots utilizing the `subplots()` operate in Matplotlib. This may be helpful while you wish to customise the format of your plots or when you could have a number of subplots and wish to optimize the spacing between them.
Utilizing the `wspace` and `hspace` Parameters:
To regulate the spacing between subplots, use the `wspace` and `hspace` parameters of the `subplots()` operate. These parameters management the width and top spacing between subplots, respectively.
The `wspace` parameter controls the horizontal spacing between subplots, whereas the `hspace` parameter controls the vertical spacing. Adjusting these parameters lets you create kind of area between your subplots.
For instance, you may set the `wspace` and `hspace` parameters to the next worth to extend the spacing between subplots. However, if you wish to lower the spacing, you may set these parameters to a decrease worth.
Instance: Adjusting Subplot Spacing
Let’s think about an instance to display methods to alter subplot spacing. Suppose we’ve a determine with two subplots organized vertically. We wish to enhance the spacing between these subplots.
We are able to set the `wspace` parameter to 0.2 and the `hspace` parameter to 0.5 to attain this. It will create more room between the subplots, each horizontally and vertically.
Code:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(2, 1, figsize=(6, 6))
fig.subplots_adjust(wspace=1, hspace=5)
# Plotting code for the subplots
plt.present()
Output:
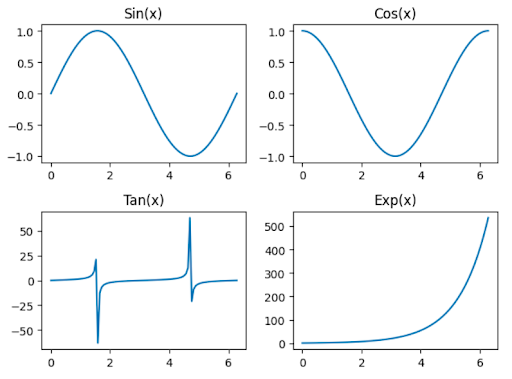
Within the above instance, we first create a determine with two subplots utilizing the `subplots()` operate. Then, we use the `subplots_adjust()` operate to regulate the spacing between the subplots by setting the `wspace` and `hspace` parameters.
You’ll be able to create visually interesting plots with the specified format and spacing between subplots by customizing the subplot spacing.
Setting Subplot Titles and Axis Labels
On this part, we’ll discover methods to add titles to subplots and axis labels to boost the visible illustration of our plots.
Including Titles to Subplots
We can use the `set_title()` operate so as to add titles to subplots. This operate permits us to specify the title for every subplot. We are able to present context and readability to our plots by giving a descriptive title.
For instance, let’s say we’ve a determine with two subplots representing the distribution of two variables. We are able to add titles to those subplots utilizing the next code:
Code:
fig, (ax1, ax2) = plt.subplots(1, 2)
ax1.set_title("Distribution of Variable A")
ax2.set_title("Distribution of Variable B")
Utilizing the `set_title()` operate, we will simply customise the titles of our subplots to convey the data we wish to current.
Including Axis Labels to Subplots
Axis labels are important for offering details about the variables represented on a plot’s x and y axes. So as to add axis labels to subplots, we will use the `set_xlabel()` and `set_ylabel()` features.
As an illustration, think about a determine with two subplots representing the connection between two variables. We are able to add axis labels to those subplots utilizing the next code:
Code:
fig, (ax1, ax2) = plt.subplots(1, 2)
ax1.set_xlabel("Variable A")
ax1.set_ylabel("Variable B")
ax2.set_xlabel("Variable C")
ax2.set_ylabel("Variable D")
Through the use of the `set_xlabel()` and `set_ylabel()` features, we will present clear labels for every subplot’s x and y axes, making it simpler for viewers to interpret the info.
Instance: Setting Subplot Titles and Axis Labels
As an example the method of setting subplot titles and axis labels, let’s think about an instance the place we’ve a determine with three subplots representing totally different points of a dataset.
Code:
import matplotlib.pyplot as plt
import numpy as np
# Producing pattern knowledge
x = np.arange(1, 6)
y1 = np.random.randint(1, 10, dimension=5)
y2 = np.random.randint(1, 10, dimension=5)
y3 = np.random.randint(1, 10, dimension=5)
fig, (ax1, ax2, ax3) = plt.subplots(1, 3)
ax1.plot(x, y1)
ax1.set_title("Plot 1")
ax1.set_xlabel("X-axis")
ax1.set_ylabel("Y-axis")
ax2.scatter(x, y2)
ax2.set_title("Plot 2")
ax2.set_xlabel("X-axis")
ax2.set_ylabel("Y-axis")
ax3.bar(x, y3)
ax3.set_title("Plot 3")
ax3.set_xlabel("X-axis")
ax3.set_ylabel("Y-axis")
# Plotting code for the subplots
plt.present()
Output:
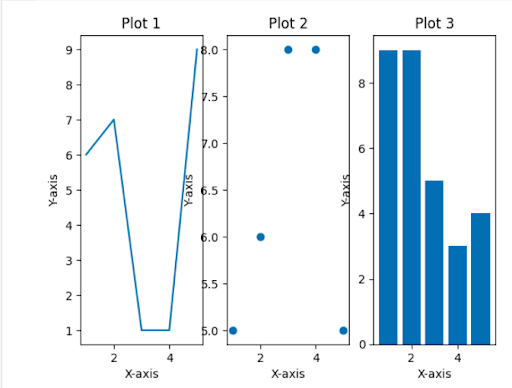
On this instance, we create a determine with three subplots, every representing a special sort of plot. We set titles for every subplot utilizing set_title () and supply axis labels utilizing set_xlabel () and set_ylabel (). This permits us to obviously label and describe every subplot, making it simpler for viewers to know the introduced knowledge.
By following these steps, we will successfully set subplot titles and axis labels to boost the visible illustration of our plots.
Controlling Subplot Sizes and Facet Ratios
When creating subplots utilizing Matplotlib’s `subplots()` operate, you may see the facet ratios of the subplots. This lets you customise the format of your plots to suit your wants finest.
suitng the figsize Parameter
One solution to management the dimensions of the subplots is through the use of the `figsize` parameter. This parameter takes in a tuple of two values, representing the determine’s width and top in inches. By adjusting these values, you may change the general dimension of the subplots.
For instance, if you wish to create a determine with subplots which can be 8 inches large and 6 inches tall, you may set the `figsize` parameter to `(8, 6)`:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(2, 2, figsize=(8, 6))
It will create a determine with a grid of two rows and two columns, the place every subplot has a dimension of 8 inches by 6 inches.
Instance: Controlling Subplot Sizes and Facet Ratios
Let’s think about an instance to additional illustrate methods to management subplot sizes and facet ratios. Suppose we wish to create a determine with two subplots, the place the primary subplot is twice as large because the second subplot.
Code:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(1, 2, figsize=(10, 5))
axs[0].plot([1, 2, 3, 4], [1, 4, 9, 16])
axs[0].set_title('Subplot 1')
axs[1].plot([1, 2, 3, 4], [1, 8, 27, 64])
axs[1].set_title('Subplot 2')
plt.present()
Output:
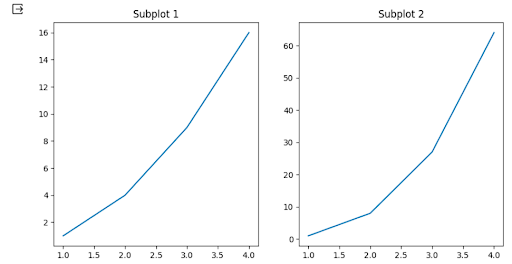
On this instance, we create a determine with a grid of 1 row and a pair of columns. We set the `figsize` parameter to `(10, 5)`, which implies the determine might be 10 inches large and 5 inches tall.
The primary subplot is plotted utilizing the info `[1, 2, 3, 4]` for the x-axis and `[1, 4, 9, 16]` for the y-axis. The second subplot is plotted utilizing the info `[1, 2, 3, 4]` for the x-axis and `[1, 8, 27, 64]` for the y-axis.
Adjusting the `figsize` parameter lets you management the general dimension of the subplots, enabling you to create customized layouts that finest fit your wants.
Conclusion
On this article about Matplotlib.pyplot.subplots() in Python, we discovered methods to management the sizes and facet ratios of subplots in Matplotlib. Utilizing the `figsize` parameter, we will alter the general dimension of the subplots. This permits us to create customized layouts that finest match our necessities. Experiment with totally different values for the `figsize` parameter to attain your plots’ desired subplot sizes and facet ratios.