Introduction
Python stands out for its simplicity, versatility, and energy within the realm of programming languages. The modulo operator (%) holds a particular place amongst its myriad built-in operators, providing a handy means to calculate remainders and carry out cyclic operations. Nevertheless, regardless of its obvious simplicity, mastering the modulo operator could be a stumbling block for a lot of Python fans. On this complete information, we delve deep into the intricacies of the Python modulo operator. So whether or not you’re a newbie simply beginning with Python or an skilled programmer trying to deepen your understanding.
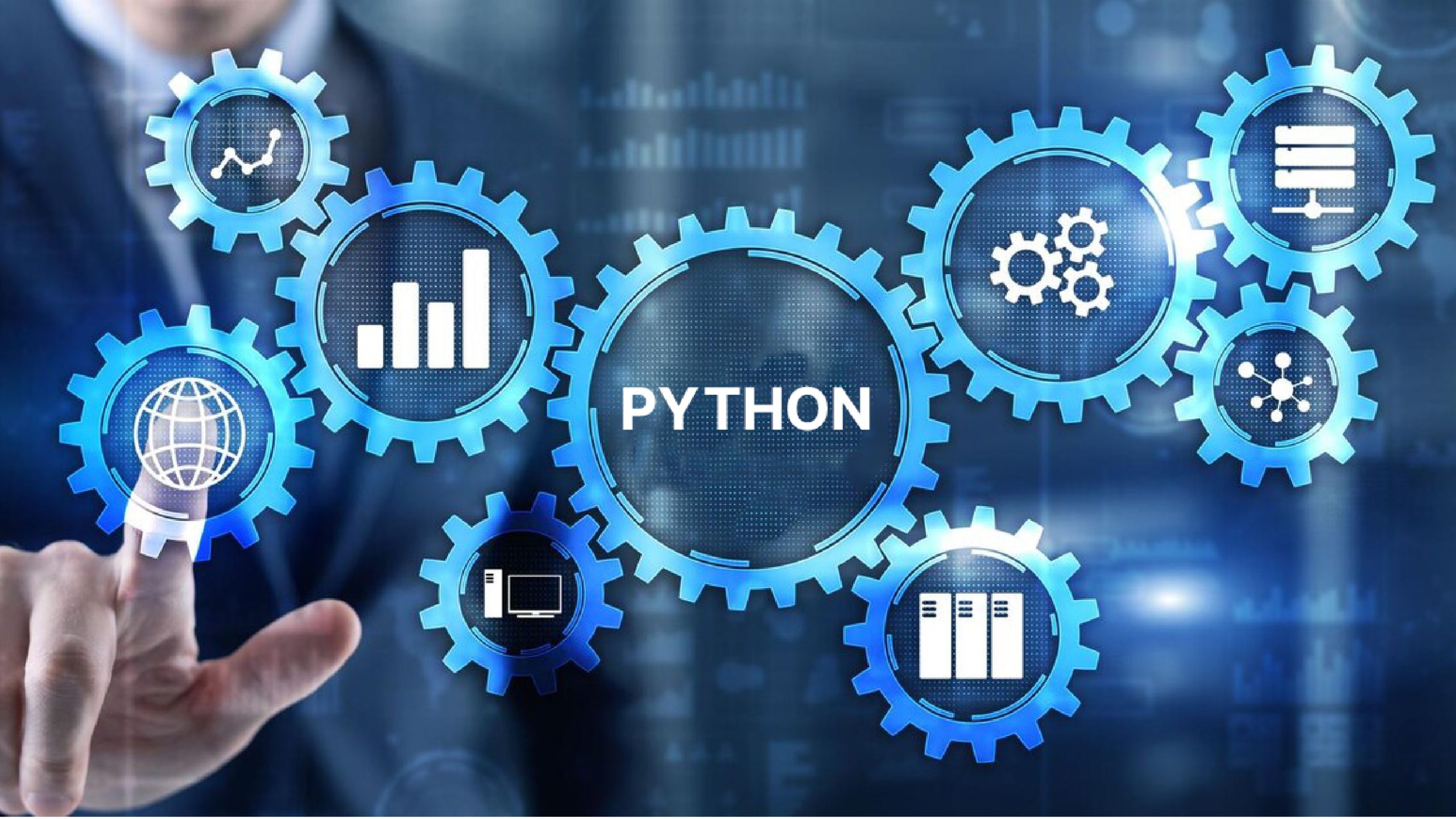
Modulo Operator Fundamentals
What’s the Python Modulo Operator?
The Python Modulo Operator, represented by the image %, is a mathematical operator that calculates the rest of a division operation.
Right here’s a easy instance: 10 % 3 would return 1, as a result of while you divide 10 by 3, you get a quotient of three and a the rest of 1.
The Modulo Operator is not only restricted to integers. It can be used with floating-point numbers. For example, 10.5 % 3 would return 1.5.
One fascinating facet of the Python Modulo Operator is its conduct with unfavourable numbers. When you have a unfavourable quantity because the dividend, the outcome will likely be optimistic but when the divisor is unfavourable then the rest may even be unfavourable. For instance, -10 % 3 would return 1 however 10 % -3 can be -1.
The Python Modulo Operator is sort of versatile and can be utilized in quite a lot of real-world eventualities, comparable to calculating the parity of a quantity (even or odd), wrapping values inside a variety, and extra.
The Modulo Operator, usually symbolized by the % signal, is a mathematical operator that finds the rest of division between two numbers. It’s a basic idea in programming and arithmetic with a variety of functions.
Division: The modulo operation includes two numbers. The primary quantity is split by the second quantity. For instance, if we have now 10 % 3, 10 is split by 3.
Code:
dividend = 10
divisor = 3
the rest = dividend % divisor
print("The rest of {} divided by {} is {}.".format(dividend, divisor, the rest))
Output:
The rest of 10 divided by 3 is 1.
Discovering the The rest: As a substitute of returning the results of the division, the modulo operation returns the rest. In our instance, 10 % 3 would return 1, as a result of 10 divided by 3 equals 3 with a the rest of 1.
Code:
dividend = 10
divisor = 3
quotient = dividend // divisor
the rest = dividend % divisor
print("The results of {} divided by {} is {} with a the rest of {}.".format(dividend, divisor, quotient, the rest))
Output:
The results of 10 divided by 3 is 3 with a the rest of 1.
Knowledge Sorts: The modulo operator can use integers and floating-point numbers. For instance, 10 % 3 and 10.5 % 3.2 are each legitimate.
Code:
int_dividend = 10
int_divisor = 3
int_remainder = int_dividend % int_divisor
print("The rest of {} divided by {} is {}.".format(int_dividend, int_divisor, int_remainder))
Output:
The rest of 10 divided by 3 is 1.
Code:
float_dividend = 10.5
float_divisor = 3.2
float_remainder = float_dividend % float_divisor
print("The rest of {} divided by {} is {}.".format(float_dividend, float_divisor, float_remainder))
Output:
The rest of 10.5 divided by 3.2 is 0.9000000000000004.
Detrimental Numbers: When coping with unfavourable numbers, the Python Modulo Operator follows the “floored division” conference. For instance, -10 % 3 would return 2, not -1. It is because -10 // 3 equals -4 with a the rest of 2.
Code:
negative_dividend = -10
divisor = 3
the rest = negative_dividend % divisor
print("The rest of {} divided by {} is {}.".format(negative_dividend, divisor, the rest))
floored_result = negative_dividend // divisor
print("The results of {} divided by {} utilizing floored division is {}.".format(negative_dividend, divisor, floored_result))
Output:
The rest of -10 divided by 3 is 2.
The results of -10 divided by 3 utilizing floored division is -4.
Zero Division: One vital factor to recollect is that the divisor (the second quantity) can’t be zero, as division by zero is undefined in arithmetic. If you happen to attempt to carry out a modulo operation with zero because the divisor, Python will elevate a ZeroDivisionError.
Code:
dividend = 10
divisor = 3
the rest = dividend % divisor
print("The rest of {} divided by {} is {}.".format(dividend, divisor, the rest))
Output:
The rest of 10 divided by 3 is 1.
How Does the Python Modulo Operator Work?
The Python Modulo Operator, denoted by %, works by dividing the quantity on the left by the quantity on the best after which returning the rest of that division.
Let’s break it down with an instance. If we have now 10 % 3:
- Python first performs the division: 10 ÷ 3. The results of this operation is 3.33 after we carry it out to a couple decimal locations.
- Nevertheless, since we’re within the the rest, Python seems to be at what number of instances 3 can match into 10 with out exceeding 10. On this case, 3 can match into 10 thrice precisely, which provides us 9.
- Lastly, Python calculates the distinction between the unique quantity (10) and the most important quantity that’s lower than 10 and is a a number of of 3 (which is 9). The distinction is 1, so 10 % 3 returns 1.
The Python Modulo Operator may also work with floating-point numbers. For instance, 10.5 % 3 would carry out the division 10.5 ÷ 3, decide that 3 suits into 10.5 thrice with a bit left over, and return that bit left over, which on this case is 1.5.
Modulo Operator with Totally different Numeric Sorts
Python Modulo Operator with integers
Utilizing the Python Modulo Operator with integers is simple. The image % represents the operator. Right here’s how you need to use it:
Select two integers: The primary is the dividend (the quantity to be divided), and the second is the divisor (the quantity by which the dividend is split). For instance, let’s select 10 because the dividend and three because the divisor.
Apply the Modulo Operator: You’d write this operation as 10 % 3 in Python. This expression tells Python to divide 10 by 3 and return the rest.
Interpret the outcome: Once you run 10 % 3 in a Python atmosphere, it is going to return 1. It is because 3 goes into 10 thrice, which equals 9, and leaves a the rest of 1.
Code:
dividend = 10
divisor = 3
the rest = dividend % divisor
print("The rest of {} divided by {} is {}.".format(dividend, divisor, the rest))
Output:
The rest of 10 divided by 3 is 1.
Python Modulo Operator with floats
The Python Modulo Operator, represented by %, can be used with floating-point numbers (or floats). Right here’s how you are able to do it:
Select two floats: The primary is the dividend (the quantity to be divided), and the second is the divisor (the quantity by which the dividend is split). For instance, let’s select 10.5 because the dividend and three.2 because the divisor.
Apply the Modulo Operator: You’d write this operation as 10.5 % 3.2 in Python. This expression tells Python to divide 10.5 by 3.2 and return the rest.
Interpret the outcome: Once you run 10.5 % 3.2 in a Python atmosphere, it is going to return 0.9. It is because 3.2 goes into 10.5 thrice, which equals 9.6, and leaves a the rest of 0.9.
Code:
dividend = 10.5
divisor = 3.2
the rest = dividend % divisor
print("The rest of {} divided by {} is {}.".format(dividend, divisor, the rest))
Output:
The rest of 10.5 divided by 3.2 is 0.9.
Python Modulo Operator with a unfavourable quantity
The Python Modulo Operator, represented by %, behaves a bit otherwise when used with unfavourable numbers.
Select two numbers: One or each of those will be unfavourable. For instance, let’s select -10 because the dividend and 3 because the divisor.
Apply the Modulo Operator: You’d write this operation as -10 % 3 in Python. This expression tells Python to divide -10 by 3 and return the rest.
Interpret the outcome: Once you run -10 % 3 in a Python atmosphere, it is going to return 2. It is because 3 goes into -10 thrice, which equals -9, and leaves a the rest of 2.
Code:
dividend = -10
divisor = 3
the rest = dividend % divisor
print("The rest of {} divided by {} is {}.".format(dividend, divisor, the rest))
Output:
The rest of -10 divided by 3 is 2.
This might sound counterintuitive at first, but it surely’s primarily based on Python’s choice to make the results of the modulo operation have the identical signal because the divisor. This is named “floored division”.
Override .__mod__() in Python Courses to Use Them with the Modulo Operator?
In Python, you may customise the conduct of operators for user-defined lessons by overriding particular strategies. The .__mod__() technique is one such particular technique that may be overridden to customise the conduct of the modulo operator (%). Right here’s how you are able to do it:
Outline a category
First, you want to outline a category. For instance, let’s create a category named MyNumber.
class MyNumber:
def __init__(self, worth):
self.worth = worth
Override the .__mod__() technique
Inside the category, you may outline a way named .__mod__(). This technique ought to take one argument apart from self, representing the opposite operand within the modulo operation.
class MyNumber:
def __init__(self, worth):
self.worth = worth
def __mod__(self, different):
return self.worth % different.worth ** 2
On this instance, the .__mod__() technique has been overridden to return the rest of the division of the worth of the present object by the sq. of the worth of the opposite object.
Use the modulo operator with cases of the category
Now, you may create cases of MyNumber and use the modulo operator with them.
# Create two cases of MyNumber
num1 = MyNumber(10)
num2 = MyNumber(3)
# Use the modulo operator with num1 and num2
outcome = num1 % num2
print("The results of the customized modulo operation is {}.".format(outcome))
Output:
The results of the customized modulo operation is 1.
Superior Makes use of of the Python Modulo Operator
The Python Modulo Operator, represented by %, is not only for locating the rest of a division operation. It has a number of superior makes use of that may be extremely helpful in your coding journey. Listed here are just a few examples:
Formatting Strings
In Python, the modulo operator can be utilized for string formatting. For instance, you need to use it to insert values right into a string with placeholders:
identify = "Alice"
age = 25
print("Whats up, my identify is %s and I'm %d years previous." % (identify, age))
Working with Time
The modulo operator can be utilized to transform seconds into hours, minutes, and seconds, which is especially helpful when working with time information:
total_seconds = 3661
hours = total_seconds // 3600
remaining_minutes = (total_seconds % 3600) // 60
remaining_seconds = (total_seconds % 3600) % 60
print("%d hours, %d minutes, and %d seconds" % (hours, remaining_minutes, remaining_seconds))
Creating Round Lists
The modulo operator can be utilized to create round lists, that are lists that wrap round on the finish. That is helpful in quite a lot of eventualities, comparable to sport improvement or information evaluation:
gadgets = ['a', 'b', 'c', 'd', 'e']
for i in vary(10):
print(gadgets[i % len(items)])
Producing Alternating Patterns in Knowledge Visualization
You should use the modulo operator to cycle by means of an inventory of colours or line kinds when plotting a number of strains on a single graph. This ensures that every line has a definite model, enhancing the readability of the graph.
import matplotlib.pyplot as plt
import numpy as np
colours = ['b', 'g', 'r', 'c', 'm', 'y', 'k']
x = np.linspace(0, 10, 100)
y = [np.sin(x + i) for i in range(7)]
for i in vary(7):
plt.plot(x, y[i], coloration=colours[i % len(colors)])
plt.present()
Making a Easy Hash Perform
The modulo operator can be utilized to create a easy hash operate, which maps information of arbitrary measurement to fixed-size values. That is helpful in lots of areas of pc science, together with information retrieval and cryptography.
def simple_hash(input_string, table_size):
sum = 0
for pos in vary(len(input_string)):
sum = sum + ord(input_string[pos])
return sum % table_size
print(simple_hash("Whats up, World!", 10))
Implementing a Round Buffer
A round buffer is an information construction that makes use of a single, fixed-size buffer as if linked end-to-end. This construction lends itself to buffering information streams. The modulo operator can calculate the index within the buffer to which the following worth (or the following a number of values) will likely be written.
class CircularBuffer:
def __init__(self, measurement):
self.buffer = [None] * measurement
self.measurement = measurement
self.index = 0
def add(self, worth):
self.buffer[self.index] = worth
self.index = (self.index + 1) % self.measurement
def __str__(self):
return str(self.buffer)
buffer = CircularBuffer(5)
for i in vary(10):
buffer.add(i)
print(buffer)
Use the Python Modulo Operator to Clear up Actual-World Issues?
The Python Modulo Operator, represented by %, is a flexible device that can be utilized to unravel quite a lot of real-world issues. Listed here are just a few examples:
Figuring out if a quantity is even or odd: In Python, you need to use the modulo operator to shortly examine if a quantity is even or odd. If quantity % 2 equals 0, the quantity is even. If it equals 1, the quantity is odd.
quantity = 7
if quantity % 2 == 0:
print("{} is even.".format(quantity))
else:
print("{} is odd.".format(quantity))
Making a wrapping impact: The modulo operator can be utilized to create a wrapping impact, which is helpful in lots of areas comparable to sport improvement. For instance, if in case you have an inventory of 5 components and also you need to get the following ingredient in a round method, you need to use (index + 1) % 5.
components = ['a', 'b', 'c', 'd', 'e']
index = 4
next_index = (index + 1) % len(components)
print("The following ingredient after {} is {}.".format(components[index], components[next_index]))
Changing seconds to hours, minutes, and seconds: When you have numerous seconds, you need to use the modulo operator to transform it into hours, minutes, and seconds.
seconds = 3661
hours = seconds // 3600
minutes = (seconds % 3600) // 60
remaining_seconds = (seconds % 3600) % 60
print("{} seconds is the same as {} hours, {} minutes, and {} seconds.".format(seconds, hours, minutes, remaining_seconds))
Calculating Leap Years: The modulo operator can be utilized to find out if a yr is a intercalary year. A yr is a intercalary year whether it is divisible by 4, however not divisible by 100, until it is usually divisible by 400.
yr = 2000
if yr % 4 == 0 and (yr % 100 != 0 or yr % 400 == 0):
print("{} is a intercalary year.".format(yr))
else:
print("{} isn't a intercalary year.".format(yr))
Creating Alternating Patterns: The modulo operator can be utilized to create alternating patterns, which will be helpful in quite a lot of eventualities, comparable to alternating row colours in a desk for higher readability.
for i in vary(10):
if i % 2 == 0:
print("That is an excellent row.")
else:
print("That is an odd row.")
Making certain Restricted Enter Vary: The modulo operator can be utilized to make sure that an enter quantity falls inside a sure vary. For instance, when you’re constructing a clock and also you need to make sure that the entered hour falls throughout the 0-23 vary, you need to use the modulo operator.
hour = 25
hour = hour % 24
print("The hour on a 24-hour clock is {}.".format(hour))
Frequent Errors and Deal with Them
You may encounter just a few widespread errors when working with the Python Modulo Operator. Right here’s how one can deal with them:
ZeroDivisionError
This error happens while you attempt to divide by zero. Within the context of the modulo operation, it occurs when the divisor is zero. To deal with this error, you need to use a try-except block:
strive:
outcome = 10 % 0
besides ZeroDivisionError:
print("Error: Division by zero isn't allowed.")
TypeError
This error happens while you attempt to use the modulo operator with incompatible sorts, comparable to a string and an integer. To deal with this error, you may make sure that each operands are numbers:
strive:
outcome = "10" % 3
besides TypeError:
print("Error: Modulo operation requires numbers.")
AttributeError
If you happen to’re working with customized lessons and also you haven’t carried out the .__mod__() technique, you may encounter this error when making an attempt to make use of the modulo operator. To deal with this error, you may implement the .__mod__() technique in your class:
class MyClass:
def __init__(self, worth):
self.worth = worth
def __mod__(self, different):
return self.worth % different.worth
strive:
outcome = MyClass(10) % MyClass(3)
besides AttributeError:
print("Error: Modulo operation not supported for this class.")
Certain, listed below are three extra widespread errors and how one can deal with them when working with the Python Modulo Operator:
Floating Level Precision Errors
When working with floating-point numbers, you may encounter precision errors as a result of approach these numbers are represented in reminiscence. To deal with this, you need to use the spherical() operate to restrict the variety of decimal locations:
outcome = 10.2 % 3.1
print("The result's {:.2f}.".format(outcome))
Modulo with Complicated Numbers
The modulo operation isn’t outlined for advanced numbers in Python. If you happen to attempt to use the modulo operator with advanced numbers, you’ll get a TypeError. To deal with this, you may examine if the operands are advanced earlier than performing the operation:
strive:
outcome = (1+2j) % (3+4j)
besides TypeError:
print("Error: Modulo operation isn't supported for advanced numbers.")
Modulo with NoneType
If one of many operands is None, you’ll get a TypeError. To deal with this, you may examine if the operands are None earlier than performing the operation:
strive:
outcome = None % 3
besides TypeError:
print("Error: Modulo operation requires numbers, not NoneType.")
Conclusion
The Python Modulo Operator is a flexible device that can be utilized in varied methods, from primary arithmetic to superior programming ideas. We’ve explored its utilization with totally different numeric sorts, how one can override the .__mod__() technique in Python lessons, and its real-world functions. We’ve additionally delved into superior makes use of and customary errors. Understanding the Python Modulo Operator is essential to mastering Python arithmetic and may open up new prospects in your coding journey.