Introduction
A bubble chart is a kind of knowledge visualization that shows knowledge factors as bubbles on a two-dimensional graph. Every bubble represents a knowledge level, and its dimension and shade can be utilized to convey extra data. On this article, we are going to discover the advantages of utilizing bubble charts in knowledge visualization and learn to create and customise bubble charts in Python.
Advantages of Utilizing Bubble Charts in Information Visualization
Bubble charts supply a number of benefits in knowledge visualization. Firstly, they permit us to characterize three variables concurrently – the x-axis, the y-axis, and the scale of the bubble. This makes it simpler to determine patterns and relationships between variables. Moreover, utilizing shade in bubble charts can present additional insights by representing a fourth variable. Bubble charts are useful when coping with massive datasets, as they will successfully show many knowledge factors with out overwhelming the viewer.
Getting Began with Bubble Charts in Python
To start out creating bubble charts in Python, we should set up the required libraries and import the required modules.
Putting in the Required Libraries
Earlier than we start, ensure you have the next libraries put in:
- Matplotlib: A well-liked knowledge visualization library in Python.
- Plotly: An interactive knowledge visualization library.
Importing the Vital Modules
As soon as the libraries are put in, we will import the required modules in our Python script:
import matplotlib.pyplot as plt
import plotly.categorical as px
Making a Fundamental Bubble Chart in Python
Now that now we have the required libraries and modules let’s create a fundamental bubble chart in Python.
Making ready the Information
We want knowledge containing three variables – x, y, and size- to create a bubble chart. Let’s assume now we have the next knowledge:
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40, 50]
dimension = [100, 200, 300, 400, 500]
Plotting the Bubble Chart
Utilizing Matplotlib, we will plot the bubble chart as follows:
plt.scatter(x, y, s=dimension)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Fundamental Bubble Chart')
plt.present()
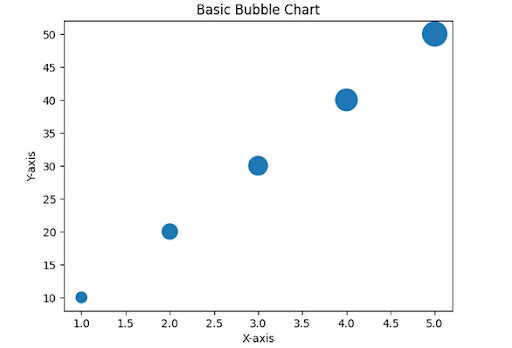
Customizing the Bubble Chart
We will customise the bubble chart by including labels, altering colours, and adjusting the scale of the bubbles. Right here’s an instance:
plt.scatter(x, y, s=dimension, c="pink", alpha=0.5)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Personalized Bubble Chart')
plt.present()
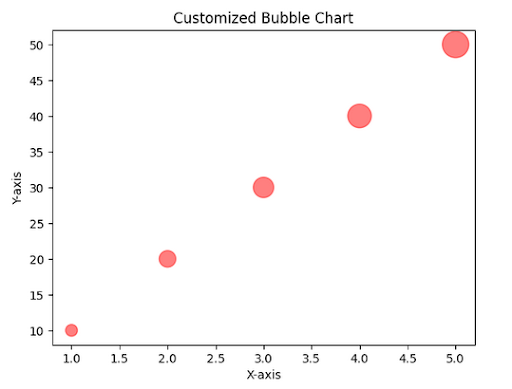
Superior Strategies for Enhancing Bubble Charts
We will incorporate extra options resembling shade and dimension variations, labels, and dealing with a number of knowledge factors and classes to reinforce bubble charts.
Including Coloration and Dimension to Bubbles
We will use the ‘c’ parameter within the scatter perform to specify the colour of the bubbles primarily based on a fourth variable. Equally, the ‘s’ parameter can be utilized to regulate the scale of the bubbles primarily based on a fifth variable.
Incorporating Labels and Annotations
To make the bubble chart extra informative, we will add labels to the bubbles utilizing the ‘textual content’ parameter within the scatter perform. Moreover, annotations might be added to focus on particular knowledge factors or present extra context.
Dealing with A number of Information Factors and Classes
Bubble charts can deal with a number of knowledge factors and classes by plotting totally different knowledge units on the identical chart. This may be achieved by calling the scatter perform quite a few instances with different knowledge and customizing every set of bubbles accordingly.
Interactive Bubble Charts with Plotly
Plotly is a robust library that enables us to create interactive and dynamic visualizations, together with bubble charts.
Putting in and Importing Plotly
To make use of Plotly, we have to set up it utilizing the next command:
pip set up plotly
After set up, we will import the required module:
import plotly.categorical as px
Creating Interactive Bubble Charts
Plotly gives a easy and intuitive API to create interactive bubble charts. Right here’s an instance:
import pandas as pd
# Pattern knowledge
knowledge = {
'x': [1, 3, 4, 6, 8],
'y': [10, 25, 40, 35, 50],
'dimension': [100, 300, 500, 200, 400],
'shade': ['red', 'blue', 'green', 'yellow', 'orange'],
'label': ['A', 'B', 'C', 'D', 'E']
}
# Creating DataFrame
df = pd.DataFrame(knowledge)
fig = px.scatter(df, x='x', y='y', dimension="dimension", shade="shade", hover_data=['label'], width=800, top=500)
fig.present()
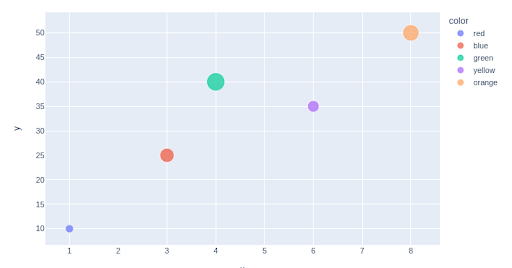
Including Interactivity and Customization Choices
Plotly permits us so as to add interactivity and customization choices to our bubble charts. We will allow zooming, panning, and hover results to offer a extra participating person expertise. Moreover, we will customise the chart’s look by altering the colour palette, marker model, and axis labels.
Suggestions and Methods for Creating Efficient Bubble Charts
To create efficient bubble charts, take into account the next suggestions and methods:
- Selecting the Proper Information and Variables: The suitable knowledge and variables are essential for creating significant bubble charts. Be certain that the variables chosen are related and supply invaluable insights.
- Designing Clear and Informative Labels: Labels convey data in bubble charts. Design clear and informative labels which might be simple to learn and perceive.
- Adjusting Bubble Sizes and Colours for Readability: To enhance readability, modify the sizes and colours of the bubbles primarily based on the info being represented. Use a shade palette that’s visually interesting and straightforward to interpret.
- Making certain Consistency and Accuracy in Information Illustration: Preserve consistency and accuracy in representing knowledge in bubble charts. Keep away from distorting the sizes or colours of the bubbles, as it could result in misinterpretation.
- Incorporating Information Insights and Storytelling: Use bubble charts as a storytelling instrument to convey knowledge insights successfully. Spotlight key findings and developments to have interaction the viewers and make the visualization extra impactful.
Conclusion
Bubble charts are a robust instrument for visualizing knowledge in Python. They permit us to characterize a number of variables concurrently and supply insights into complicated datasets. Following this text’s strategies and finest practices, you may create informative and visually interesting bubble charts that successfully talk your knowledge. So, begin exploring the world of bubble charts in Python and unlock the potential of your knowledge visualization.