Welcome to the Python Error Dealing with MCQ Quiz! Error dealing with is a necessary side of programming, and Python offers highly effective mechanisms to handle exceptions and errors that will happen throughout program execution. This quiz goals to check your understanding of varied ideas associated to error dealing with in Python, together with attempt
and besides
blocks, elevating and catching exceptions, utilizing lastly
blocks, and extra. Every query is multiple-choice, with just one appropriate reply. Take your time to rigorously learn every query and select the best choice. Let’s start and discover Python error dealing with collectively!
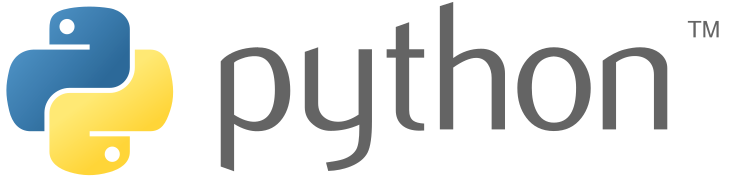
Q1. What’s the function of the attempt block in Python error dealing with?
a) To outline the block of code the place an exception might happen
b) To catch and deal with exceptions that happen inside the block
c) To make sure that the code executes with none errors
d) To terminate this system if an exception happens
Reply: a
Clarification: The attempt block defines a block of code by which exceptions might happen.
Q2. Which key phrase is used to catch exceptions in Python?
a) attempt
b) catch
c) besides
d) deal with
Reply: c
Clarification: The besides key phrase is used to catch and deal with exceptions in Python.
Q3. What’s raised when a Python program encounters an error throughout execution?
a) Error
b) Exception
c) Fault
d) Bug
Reply: b
Clarification: In Python, errors throughout execution are represented as exceptions.
This fall. Which of the next is NOT a regular Python exception?
a) KeyError
b) ValueException
c) IndexError
d) TypeError
Reply: b
Clarification: ValueException is just not a regular Python exception. It needs to be ValueError.
Q5. How will you deal with a number of exceptions in a single besides block?
a) Separate the exceptions utilizing commas
b) Use nested besides blocks
c) Use the besides key phrase solely as soon as
d) It’s not attainable to deal with a number of exceptions in a single besides block
Reply: a
Clarification: You may separate a number of exceptions utilizing commas in a single besides block.
Q6. Which Python key phrase is used to boost an exception manually?
a) throw
b) increase
c) exception
d) set off
Reply: b
Clarification: The increase key phrase is used to boost exceptions manually in Python.
Q7. What does the lastly block in Python error dealing with guarantee?
a) It ensures the code inside it can all the time execute, no matter whether or not an exception happens or not.
b) It ensures this system will terminate if an exception happens.
c) It ensures that this system will skip executing the code if an exception happens.
d) It ensures that solely the code inside the lastly block will execute if an exception happens.
Reply: a
Clarification: The lastly block ensures that the code inside it can all the time execute, no matter whether or not an exception happens or not.
Q8. Which of the next is true concerning the else block in Python error dealing with?
a) It’s executed if an exception happens.
b) It’s executed if no exception happens within the attempt block.
c) It handles exceptions occurring within the attempt block.
d) It’s executed as a substitute of the lastly block.
Reply: b
Clarification: Python executes the else block if no exception happens within the attempt block.
Q9. What’s the function of the assert assertion in Python?
a) To deal with exceptions
b) To terminate this system
c) To verify if a situation is true
d) To boost an exception
Reply: c
Clarification: The assert assertion checks if a situation is true in Python. If the situation is fake, it raises an AssertionError.
Q10.Which of the next strategies is NOT a generally used technique of the Exception class in Python?
a) __str__()
b) __init__()
c) __cause__()
d) __repr__()
Reply: c
Clarification: __cause__() is just not a technique of the Exception class. It’s used to get the reason for the exception.
Q11. Which of the next statements is true concerning the besides clause in Python?
a) It’s obligatory in a try-except block.
b) It catches all exceptions by default.
c) It have to be positioned earlier than the attempt block.
d) It might probably specify the kind of exceptions to catch.
Reply: d
Clarification: The besides clause can specify the kind of exceptions to catch.
Q12. What’s the output of the next code?
attempt:
x = 10 / 0
besides ZeroDivisionError:
print("Division by zero")
lastly:
print("Lastly block")
a) Division by zero
Lastly block
b) Lastly block
c) Division by zero
d) ZeroDivisionError
Reply: a
Clarification: The code will increase a ZeroDivisionError, catch it, print “Division by zero”, after which execute the lastly block printing “Lastly block”.
Q13. Which of the next key phrases is used to deal with the exception block in Python?
a) hand
b) rescue
c) besides
d) catch
Reply: c
Clarification: besides is used to deal with exception blocks in Python.
Q14. What does the next Python code do?
attempt:
# Some code that will increase an exception
besides:
move
a) It raises an exception.
b) It catches all exceptions and ignores them.
c) It terminates this system.
d) It handles exceptions gracefully.
Reply: b
Clarification: This code catches all exceptions and ignores them as a result of move assertion.
Q15. What occurs if an exception happens within the lastly block itself?
a) The exception is caught by the besides block.
b) This system terminates.
c) The exception propagates up the decision stack.
d) The exception is ignored.
Reply: c
Clarification: If an exception happens within the lastly block itself, it propagates up the decision stack.
Q16. Which of the next is NOT a typical built-in exception in Python?
a) KeyError
b) FileNotFoundError
c) IndexError
d) SyntaxError
Reply: d
Clarification: SyntaxError is a typical syntax error, however it’s not a built-in exception class.
Q17. What’s the function of the sys.exc_info() perform in Python?
a) It raises an exception.
b) It returns details about the present exception being dealt with.
c) It terminates this system.
d) It prints the traceback of the exception.
Reply: b
Clarification: sys.exc_info() returns a tuple of details about the present exception being dealt with in Python.
Q18. Which key phrase is used to re-raise the final exception that was caught in Python?
a) rethrow
b) rethrow_last
c) raise_last
d) increase
Reply: d
Clarification: In Python, builders use the increase key phrase to re-raise the final exception that was caught.
Q19. What’s the output of the next code?
attempt:
increase Exception("An error occurred")
besides Exception as e:
print(e)
lastly:
print("Lastly block")
a) An error occurred
Lastly block
b) Lastly block
c) An error occurred
d) Exception
Reply: a
Clarification: The code raises an Exception, catches it, prints the error message, after which executes the lastly block.
Q20. Which assertion is true about dealing with exceptions in Python?
a) An exception handler can catch exceptions raised by features it calls.
b) An exception handler can’t catch exceptions raised by features it calls.
c) An exception handler solely catches exceptions raised in the identical block.
d) An exception handler can solely catch exceptions of the identical kind.
Reply: a
Clarification: An exception handler can catch exceptions raised by features it calls.
Q21. What’s the function of the traceback module in Python?
a) It means that you can customise error messages.
b) It prints detailed details about exceptions.
c) It handles exceptions in multi-threaded purposes.
d) It raises exceptions based mostly on user-defined circumstances.
Reply: b
Clarification: The traceback module offers features to print detailed details about exceptions.
Q22. Which of the next statements is true concerning the assert assertion in Python?
a) It’s used to deal with exceptions.
b) It terminates this system if a situation is true.
c) It raises an exception if a situation is fake.
d) It’s just like the try-except block.
Reply: c
Clarification: The assert assertion raises an AssertionError if a situation is fake.
Q23. How will you create a customized exception class in Python?
a) By inheriting from the Exception class
b) By utilizing the throw key phrase
c) By utilizing the custom_exception key phrase
d) By defining a perform with the title of the exception
Reply: a
Clarification: You may create a customized exception class by inheriting from the Exception class.
Q24. What does the sys.exit() perform do in Python?
a) Raises an exception
b) Terminates this system
c) Prints a message to the console
d) Handles exceptions
Reply: b
Clarification: sys.exit() terminates this system.
Q25. Which of the next is true concerning the else block in Python error dealing with?
a) It’s executed if an exception happens.
b) It handles exceptions occurring within the attempt block.
c) It’s executed provided that an exception happens.
d) It’s executed if no exception happens within the attempt block.
Reply: d
Clarification: If no exception happens within the attempt block, Python executes the else block.
Q26. What’s the function of the increase assertion in Python?
a) To catch exceptions
b) To disregard exceptions
c) To re-raise exceptions
d) To terminate this system
Reply: c
Clarification: In Python, builders use the increase assertion to re-raise exceptions.
Q27. Which of the next is NOT a regular Python exception?
a) SyntaxError
b) ZeroDivisionError
c) OverflowError
d) RuntimeError
Reply: c
Clarification: OverflowError is a regular Python exception, however it’s not as frequent because the others listed.
Q28. What’s the function of the try-except-else block in Python?
a) To catch exceptions and execute different code
b) To execute code that ought to all the time run
c) To deal with exceptions and execute code if no exception happens
d) To terminate this system if an exception happens
Reply: c
Clarification: In Python, builders use the try-except-else block to deal with exceptions and execute code if no exception happens.
Q29. Which key phrase is used to outline a customized exception class in Python?
a) catch
b) increase
c) class
d) exception
Reply: c
Clarification: In Python, builders use the category key phrase to outline customized exception courses.
Q30. What’s the function of the lastly block in Python error dealing with?
a) To deal with exceptions
b) To boost exceptions
c) To make sure that sure code will all the time be executed
d) To terminate this system
Reply: c
Clarification: The lastly block ensures that sure code executes, no matter whether or not an exception happens.
Q31. Which of the next exceptions is raised when making an attempt to entry an index in an inventory that doesn’t exist?
a) ValueError
b) KeyError
c) IndexError
d) TypeError
Reply: c
Clarification: Trying to entry an index that’s out of vary in a sequence like an inventory raises an IndexError.
Q32. In a try-except block, can we’ve got a number of besides blocks?
a) No, just one besides block is allowed
b) Sure, however provided that they deal with various kinds of exceptions
c) Sure, whatever the sorts of exceptions they deal with
d) Sure, however provided that they’re nested inside one another
Reply: b
Clarification: In Python, ordering a number of besides blocks from most particular to least particular is taken into account good apply as a result of Python checks them so as and executes the primary matching block.
Q33. Which of the next statements is true concerning the order of besides blocks in a try-except assemble?
a) The order of besides blocks doesn’t matter
b) Probably the most particular exception handlers ought to come first
c) Probably the most common exception handlers ought to come first
d) Python raises a SyntaxError if besides blocks aren’t within the appropriate order
Reply: b
Clarification: In Python, it’s good apply to order a number of besides blocks from most particular to least particular as a result of Python checks them so as and executes the primary matching block.
Congratulations on finishing the Python Error Dealing with MCQ Quiz! We hope this quiz has helped reinforce your understanding of Python error dealing with ideas and strategies. Managing exceptions successfully is essential for writing strong and dependable Python code. By mastering error dealing with, you’ll be able to make sure that your packages gracefully deal with surprising conditions and supply significant suggestions to customers. Maintain working towards and exploring Python’s error dealing with mechanisms to change into a proficient Python developer. When you’ve got any questions or wish to delve deeper into any matter, don’t hesitate to proceed your studying journey. Comfortable coding!
You can even enroll in out free Python Course As we speak!
Learn our extra articles associated to MCQs in Python: