Introduction
Getters and setters are important to object-oriented programming (OOP) in Python. They supply a technique to encapsulate knowledge and management entry to it. On this article, we are going to discover what getters and setters are, their advantages, and methods to implement them in Python. We will even talk about finest practices, present examples, examine them with direct attribute entry, and spotlight widespread pitfalls and errors.
What are Getters and Setters?
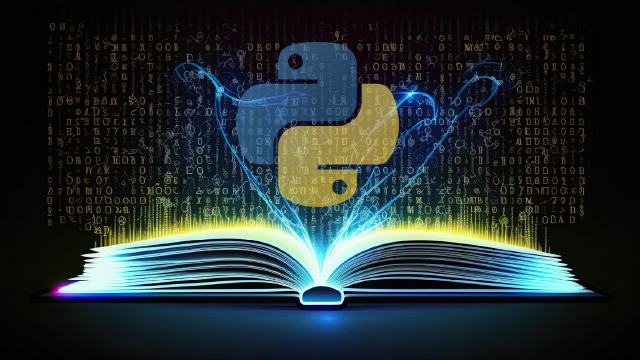
Getters and setters enable us to retrieve and modify the values of personal attributes in a category. They supply a degree of abstraction by separating the inner illustration of knowledge from its exterior entry. Getters are used to retrieve the worth of an attribute, whereas setters are used to switch or set the worth of an attribute.
Advantages of Utilizing Getters and Setters in Python
Utilizing getters and setters in Python provides a number of advantages. Firstly, they assist encapsulate knowledge and management entry to it. By non-public attributes and offering getters and setters, we will be certain that the information is accessed and modified solely by means of the outlined strategies. This helps in sustaining knowledge integrity and prevents unauthorized entry.
Secondly, getters and setters enable us to implement knowledge validation and be certain that solely legitimate values are assigned to attributes. We are able to add situations and checks within the setter strategies to validate the enter earlier than assigning it to the attribute. This helps preserve knowledge integrity and stop the introduction of invalid or inconsistent knowledge.
Thirdly, getters and setters present compatibility and adaptability. If we determine to alter the inner illustration of knowledge or add further logic sooner or later, we will achieve this with out affecting the exterior interface of the category. The exterior code that makes use of the category will proceed to work seamlessly with the up to date implementation.
Lastly, utilizing getters and setters helps doc and talk the category’s intent. By offering significant names for the getter and setter strategies, we will convey the aim and utilization of the attributes to different builders who may use our code. This improves code readability and maintainability.
Implementing Getters and Setters in Python
There are a number of methods to implement getters and setters in Python. Let’s discover among the widespread approaches:
Utilizing Property Decorators
Python supplies a built-in property decorator that permits us to outline getters and setters concisely and elegantly. The property decorator converts a technique right into a read-only attribute, and we will outline a setter technique utilizing the identical decorator.
class Individual:
def __init__(self, identify):
self._name = identify
@property
def identify(self):
return self._name
@identify.setter
def identify(self, worth):
self._name = worth
The above instance defines a `Individual` class with a personal attribute _name.
We use the `@property` decorator to outline a getter technique `identify` that returns the worth of _name.
We additionally describe a setter technique `identify` utilizing the `@identify.setter` decorator, which permits us to switch the worth of _name.
Manually Defining Getter and Setter Strategies
One other method to implementing getters and setters is by manually defining the strategies. This provides us extra management over the implementation and permits us so as to add further logic if wanted.
class Individual:
def __init__(self, identify):
self._name = identify
def get_name(self):
return self._name
def set_name(self, worth):
self._name = worth
The above instance defines a `Individual` class with a personal attribute _name.
We manually outline a getter technique `get_name` that returns the worth of `_name`, and a setter technique `set_name` that permits us to switch the worth of `_name`.
Greatest Practices for Utilizing Getters and Setters in Python
When utilizing getters and setters in Python, it’s important to observe some finest practices to make sure clear and maintainable code. Let’s talk about a few of these practices:
- Encapsulating Knowledge and Controlling Entry: The first function of getters and setters is to encapsulate knowledge and management entry to it. Making attributes non-public by conference (utilizing an underscore prefix) and offering getters and setters for accessing and modifying the information is really useful. This helps in sustaining knowledge integrity and prevents unauthorized entry.
- Guaranteeing Knowledge Integrity and Validation: Getters and setters present a chance to validate the enter earlier than assigning it to an attribute. Including validation checks within the setter strategies is sweet apply to make sure that solely legitimate values are assigned. This helps in sustaining knowledge integrity and prevents the introduction of invalid or inconsistent knowledge.
- Offering Compatibility and Flexibility: Through the use of getters and setters, we will change the inner illustration of knowledge or add further logic with out affecting the exterior interface of the category. It is strongly recommended to make use of getters and setters even when they appear pointless. This supplies compatibility and adaptability for future modifications.
- Documenting and Speaking Intent: Meaningful names for getter and setter strategies assist in establishing and speaking the intent of the category. It’s good apply to make use of descriptive names that convey the aim and utilization of the attributes. This improves code readability and makes it simpler for different builders to know and use our code.
Examples of Utilizing Getters and Setters in Python
Let’s discover some examples to know methods to use getters and setters in Python.
Fundamental Getter and Setter Strategies
class Circle:
def __init__(self, radius):
self._radius = radius
def get_radius(self):
return self._radius
def set_radius(self, radius):
if radius > 0:
self._radius = radius
else:
elevate ValueError("Radius have to be better than 0")
circle = Circle(5)
print(circle.get_radius()) # Output: 5
circle.set_radius(10)
print(circle.get_radius()) # Output: 10
circle.set_radius(-5) # Raises ValueError
Within the above instance, we outline a `Circle` class with a personal attribute `_radius`. We offer getter and setter strategies `get_radius` and `set_radius` to entry and modify the worth of `_radius`. The setter technique features a validation test to make sure that the radius is bigger than 0.
Utilizing Getters and Setters for Calculated Properties
class Rectangle:
def __init__(self, size, width):
self._length = size
self._width = width
def get_area(self):
return self._length * self._width
def set_length(self, size):
if size > 0:
self._length = size
else:
elevate ValueError("Size have to be better than 0")
def set_width(self, width):
if width > 0:
self._width = width
else:
elevate ValueError("Width have to be better than 0")
rectangle = Rectangle(5, 10)
print(rectangle.get_area()) # Output: 50
rectangle.set_length(8)
rectangle.set_width(12)
print(rectangle.get_area()) # Output: 96
The above instance defines a `Rectangle` class with non-public attributes `_length` and `_width`. We offer a getter technique, `get_area`, to calculate and return the realm of the rectangle. We additionally present setter strategies `set_length` and `set_width` to switch the values of `_length` and `_width`.
Implementing Learn-Solely and Write-Solely Properties
class BankAccount:
def __init__(self, steadiness):
self._balance = steadiness
@property
def steadiness(self):
return self._balance
@steadiness.setter
def steadiness(self, worth):
elevate AttributeError("Can't modify steadiness straight")
@property
def is_overdrawn(self):
return self._balance < 0
account = BankAccount(1000)
print(account.steadiness) # Output: 1000
account.steadiness = 2000 # Raises AttributeError
print(account.is_overdrawn) # Output: False
account._balance = -500
print(account.is_overdrawn) # Output: True
The above instance defines a `BankAccount` class with a personal attribute `_balance`. We use the `@property` decorator to outline a getter technique `steadiness` that returns the worth of `_balance`. We additionally outline a setter technique, `steadiness,` that raises an `AttributeError` to forestall direct steadiness modification. Moreover, we outline a read-only property `is_overdrawn` that returns `True` if the steadiness is detrimental.
Making use of Getters and Setters in Inheritance and Polymorphism
class Animal:
def __init__(self, identify):
self._name = identify
def get_name(self):
return self._name
def set_name(self, identify):
self._name = identify
class Canine(Animal):
def __init__(self, identify, breed):
tremendous().__init__(identify)
self._breed = breed
def get_breed(self):
return self._breed
def set_breed(self, breed):
self._breed = breed
canine = Canine("Buddy", "Labrador")
print(canine.get_name()) # Output: Buddy
print(canine.get_breed()) # Output: Labrador
canine.set_name("Max")
canine.set_breed("Golden Retriever")
print(canine.get_name()) # Output: Max
print(canine.get_breed()) # Output: Golden Retriever
The above instance defines an `Animal` class with a personal attribute `_name` and getter and setter strategies. We then outline a `Canine` class that inherits from `Animal` and provides a personal attribute `_breed` together with getter and setter strategies for it. We create an occasion of `Canine` and show methods to use the getter and setter strategies for inherited and added attributes.
Comparability with Direct Attribute Entry in Python
Direct attribute entry refers to accessing and modifying attributes straight with out utilizing getters and setters. Whereas direct attribute entry is less complicated and extra concise, utilizing getters and setters provides a number of benefits.
Execs and Cons of Direct Attribute Entry
Direct attribute entry is easy and requires much less code. It’s appropriate for easy courses the place knowledge integrity and validation are usually not vital. Nevertheless, direct attribute entry lacks encapsulation and management over knowledge entry. It might result in unauthorized modification of attributes and the introduction of invalid or inconsistent knowledge.
When to Use Getters and Setters vs. Direct Attribute Entry
Getters and setters must be used when encapsulating knowledge, controlling entry, and making certain knowledge integrity are vital. They’re notably helpful when validation and extra logic are required throughout attribute project. Direct attribute entry can be utilized in easy circumstances the place knowledge integrity and validation are usually not vital.
Widespread Pitfalls and Errors with Getters and Setters
Whereas getters and setters are highly effective instruments, there are some widespread pitfalls and errors to keep away from.
- Overusing or Misusing Getters and Setters: You will need to steadiness and keep away from overusing or misusing them. Not each attribute wants a getter and setter, particularly if it’s a easy attribute with no further logic or validation necessities. Overusing getters and setters can result in pointless complexity and decreased code readability.
- Not Implementing Correct Validation or Error Dealing with: It’s essential to implement correct validation and error dealing with when utilizing setters. Failing to take action can lead to invalid or inconsistent knowledge. It’s good apply to lift applicable exceptions or errors when assigning invalid values to attributes.
- Creating Complicated or Inefficient Getter and Setter Strategies: Getter and setter strategies must be stored easy and environment friendly. Keep away from including pointless complexity or performing costly operations inside these strategies. Complicated or inefficient getter and setter strategies can influence the efficiency of the code and make it tougher to know and preserve.
- Failing to Doc or Talk the Use of Getters and Setters: You will need to doc and talk the usage of getters and setters within the code. Failing to take action could make it troublesome for different builders to know and use the code accurately. Use significant names for getter and setter strategies and doc their function and utilization.
Conclusion
Getters and setters are highly effective instruments in Python that enable us to encapsulate knowledge, management entry to it, and guarantee knowledge integrity. They supply abstraction and adaptability that enhance code maintainability and readability. By following finest practices and avoiding widespread pitfalls, we will leverage the advantages of getters and setters in our Python code.