Introduction
When working with Python, it’s important to grasp the idea of sophistication and occasion attributes. These attributes are essential in defining the conduct and traits of objects in a program. This text will discover the variations between class and occasion attributes, tips on how to declare and entry them, and greatest practices for utilizing them successfully.
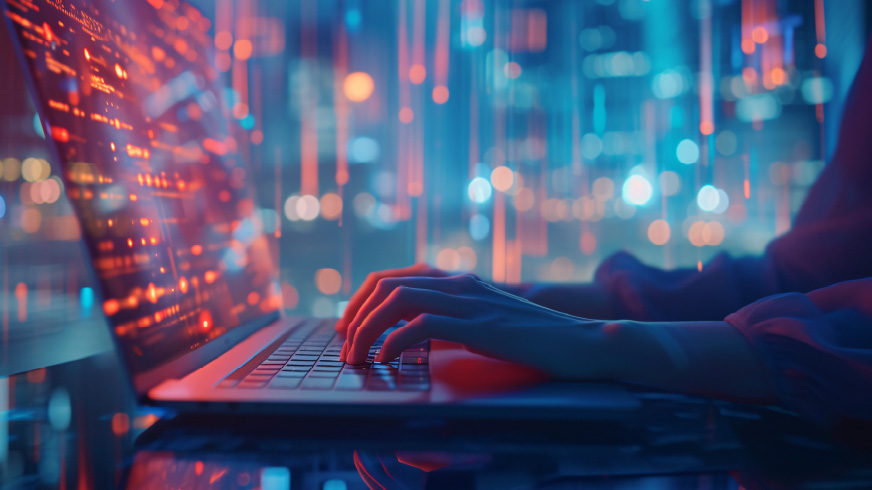
Understanding Class and Occasion Attributes
Class attributes are attributes which are shared by all situations of a category. They’re outlined inside the class definition and are accessible to all of the circumstances of that class. However, occasion attributes are distinctive to every occasion of a category. They’re outlined inside the strategies of a category and are particular to the occasion they belong to.
Declaring Class Attributes
To declare a category attribute, you outline it inside the class definition. Let’s contemplate an instance the place we’ve a category referred to as “Automotive” with a category attribute referred to as “wheels” representing the variety of wheels a automobile has.
class Automotive:
wheels = 4
The syntax for declaring class attributes is simple. You outline the attribute inside the class definition, outdoors of any strategies or capabilities.
Accessing Class Attributes
Class attributes could be accessed utilizing the dot notation, similar to occasion attributes. For instance, to entry the “wheels” attribute of the “Automotive” class, you’d use the next syntax:
print(Automotive.wheels)
Modifying Class Attributes
Class attributes could be modified by accessing them utilizing the category title and reassigning a brand new worth to them. For instance, if we need to change the variety of wheels for all automobiles to six, we will do the next:
Automotive.wheels = 6
Declaring Occasion Attributes
Occasion attributes are declared inside the strategies of a category. They’re particular to every class occasion and may fluctuate from one occasion to a different. Let’s contemplate an instance the place we’ve a category referred to as “Individual” with an occasion attribute referred to as “title” that represents an individual’s title.
class Individual:
def __init__(self, title):
self.title = title
Syntax for Declaring Occasion Attributes
Occasion attributes are declared inside a category’s `__init__` methodology. The `__init__` methodology is a novel methodology in Python that’s mechanically referred to as when a brand new class occasion is created. Inside this methodology, you’ll be able to outline and initialize occasion attributes.
Accessing Occasion Attributes
Occasion attributes could be accessed utilizing the dot notation. For instance, to entry the “title” attribute of a “Individual” occasion, you’d use the next syntax:
particular person = Individual("John")
print(particular person.title)
Modifying Occasion Attributes
Occasion attributes could be modified by accessing them utilizing the occasion title and reassigning a brand new worth. For instance, if we need to change the title of an individual, we will do the next:
person.title = "Jane"
Distinction Between Class and Occasion Attributes
The principle distinction between class and occasion attributes is that every one situations of a category share class attributes, whereas occasion attributes are particular to every occasion. Class attributes are outlined inside the class definition. They’re accessible to all of the circumstances, whereas occasion attributes are outlined inside the strategies of a category and are particular to every occasion.
Utilizing Class and Occasion Attributes Collectively
Class and occasion attributes can be utilized collectively to outline the conduct and traits of objects in a program. For instance, let’s contemplate a category referred to as “Rectangle” with class attributes for the width and peak and an occasion attribute for the colour of every rectangle.
class Rectangle:
width = 10
peak = 5
def __init__(self, shade):
self.shade = shade
On this instance, the width and peak are class attributes shared by all situations of the “Rectangle” class, whereas the colour is an occasion attribute particular to every rectangle.
Inheritance and Attribute Decision
When working with inheritance in Python, attribute decision follows a selected order generally known as the Methodology Decision Order (MRO). This order determines how attributes are inherited and accessed in a category hierarchy.
Finest Practices for Utilizing Class and Occasion Attributes
Following some greatest practices to make use of class and occasion attributes successfully is crucial. These practices embrace utilizing correct naming conventions, avoiding mutable default values, utilizing class attributes for constants, and documenting attribute utilization.
Naming Conventions: When naming class and occasion attributes, it’s really helpful to make use of lowercase letters and underscores for improved readability. For instance, as a substitute of “myAttribute,” it’s higher to make use of “my_attribute.”
- Avoiding Mutable Default Values: When defining occasion attributes, it’s necessary to keep away from utilizing mutable default values equivalent to lists or dictionaries. It’s because mutable default values are shared amongst all class situations, resulting in surprising conduct. As a substitute, initialize mutable attributes inside the `__init__` methodology.
- Utilizing Class Attributes for Constants: Class attributes can outline constants inside a category. Constants are values that don’t change all through the execution of a program. Through the use of class attributes for constants, you’ll be able to simply entry and modify them if wanted.
- Documenting Attribute Utilization: To enhance code readability and maintainability, it’s important to doc the utilization of sophistication and occasion attributes. This may be performed utilizing feedback or docstrings to offer details about the aim and conduct of every attribute.
Conclusion
In conclusion, class and occasion attributes are important ideas in Python programming. They permit us to outline the conduct and traits of objects in a program. We are able to write extra environment friendly and maintainable code by understanding tips on how to declare, entry, and modify class and occasion attributes and following greatest practices. So, use class and occasion attributes successfully in your Python applications to reinforce their performance and readability.