Introduction
Python’s Counter is a strong information construction conveniently counts components in an iterable. It’s a part of the collections module and provides numerous functionalities for counting, combining, and manipulating information. On this article, we’ll discover the fundamentals of Counters, on a regular basis use circumstances, superior strategies, and suggestions for optimizing efficiency utilizing Python’s Counter successfully.
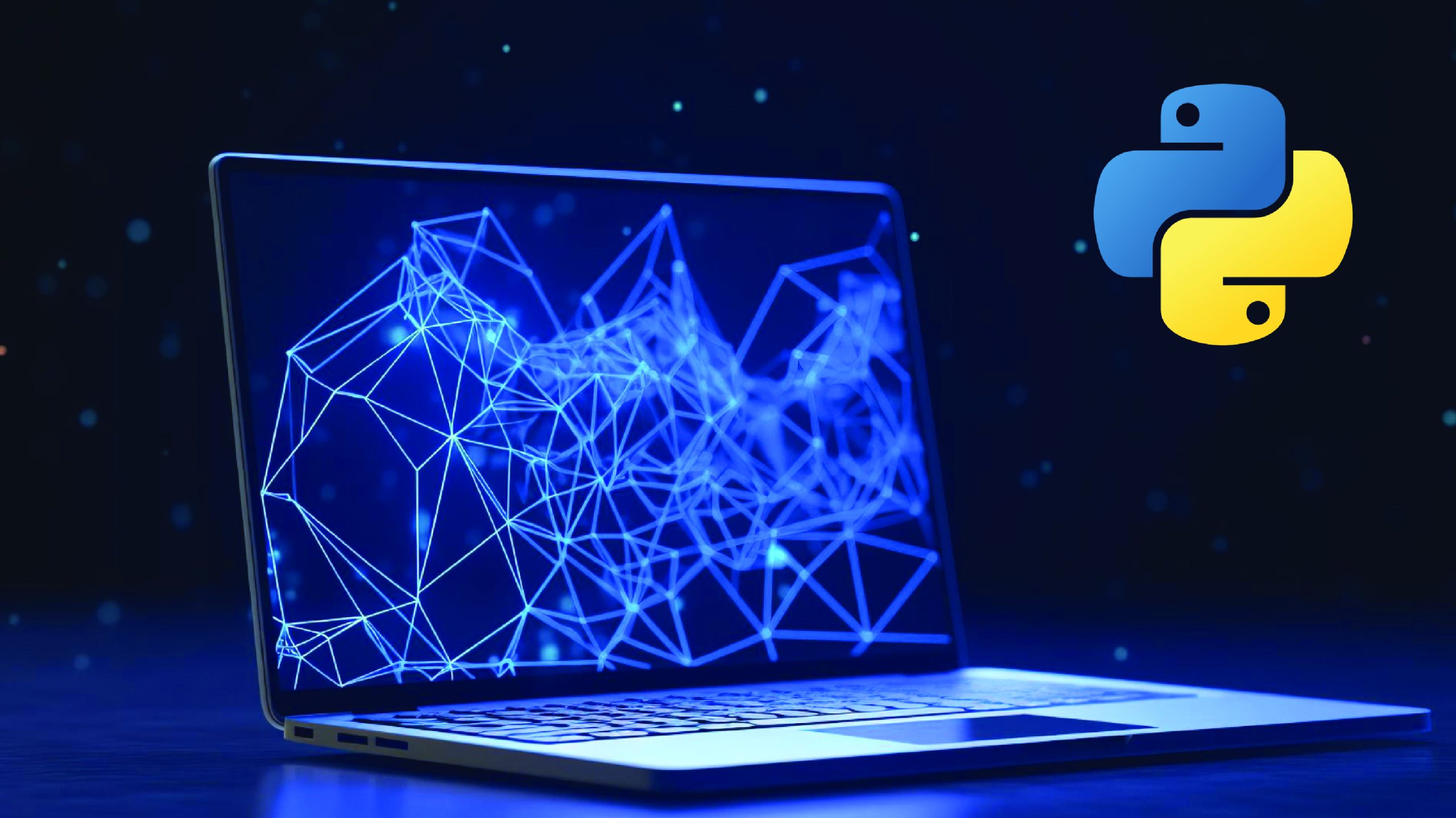
Additionally Learn: Python Enumerate(): Simplify Looping With Counters
Understanding the Fundamentals of Counters
Making a Counter Object
To create a Counter object, we will merely move an iterable to the Counter() constructor. The iterable is usually a listing, tuple, string, or every other sequence. For instance:
from collections import Counter
my_list = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
counter = Counter(my_list)
print(counter)
Output:
Counter({1: 3, 2: 3, 3: 2, 4: 1, 5: 1}
Accessing and Modifying Counter Parts
We will entry the depend of a selected component in a Counter utilizing the sq. bracket notation. Moreover, we will modify the depend of a component by assigning a brand new worth to it. For instance:
counter = Counter({'a': 3, 'b': 2, 'c': 1})
print(counter['a']) # Output: 3
counter['b'] = 5
print(counter) # Output: Counter({'a': 3, 'b': 5, 'c': 1})
Counting Parts in an Iterable
Counters are notably helpful for counting the frequency of components in an iterable. We will use the Counter’s most_common() methodology to get a listing of components and their counts, sorted by the depend in descending order. For instance:
textual content = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."
counter = Counter(textual content.decrease().cut up())
print(counter.most_common(3))
Output:
[(‘ipsum’, 1), (‘lorem’, 1), (‘dolor’, 1)]
Combining Counters
We will mix a number of Counters utilizing the addition operator (+). This operation sums the counts of widespread components in each Counters. For instance:
counter1 = Counter({'a': 3, 'b': 2, 'c': 1})
counter2 = Counter({'b': 4, 'c': 2, 'd': 1})
combined_counter = counter1 + counter2
print(combined_counter)
Output:
Counter({‘b’: 6, ‘a’: 3, ‘c’: 3, ‘d’: 1})
Eradicating Parts from Counters
To take away components from a Counter, we will use the del key phrase adopted by the component we wish to delete. This operation fully removes the component from the Counter. For instance:
counter = Counter({'a': 3, 'b': 2, 'c': 1})
del counter['b']
print(counter)
Output:
Counter({‘a’: 3, ‘c’: 1})
Widespread Use Circumstances for Python’s Counter
Discovering Most Widespread Parts
Counters may discover the most typical components in any iterable. The most_common() methodology returns a listing of components and their counts, sorted by the depend in descending order. For instance:
my_list = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
counter = Counter(my_list)
print(counter.most_common(2))
Output:
[(1, 3), (2, 3)]
Figuring out Duplicate Parts
Counters can assist determine duplicate components in an iterable by checking if the depend of any component is larger than 1. This may be helpful in information cleansing and deduplication duties. For instance:
my_list = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
counter = Counter(my_list)
duplicates = [element for element, count in counter.items() if count > 1]
print(duplicates)
Output:
[1, 2, 3]
Implementing Multisets and Luggage
Counters can be utilized to implement multisets and baggage, that are collections that permit duplicate components. By treating the weather as keys and their counts as values, we will carry out numerous operations on multisets and baggage effectively. For instance:
multiset = Counter({'a': 3, 'b': 2, 'c': 1})
print(multiset['a']) # Output: 3
bag = Counter({'a': 3, 'b': 2, 'c': 1})
print(bag['a']) # Output: 3
Monitoring Stock and Inventory Ranges
Counters can monitor stock and inventory ranges in a retail or warehouse administration system. We will simply replace and retrieve the inventory ranges by associating every merchandise with its depend. For instance:
stock = Counter(apples=10, oranges=5, bananas=3)
print(stock['apples']) # Output: 10
stock['apples'] -= 2
print(stock['apples']) # Output: 8
Superior Methods with Python’s Counter
Subtraction and Intersection of Counters
Counters help subtraction and intersection operations. Subtracting one Counter from one other subtracts the counts of widespread components, whereas intersecting two Counters retains the minimal depend of widespread components. For instance:
counter1 = Counter({'a': 3, 'b': 2, 'c': 1})
counter2 = Counter({'b': 4, 'c': 2, 'd': 1})
subtracted_counter = counter1 - counter2
print(subtracted_counter) # Output: Counter({'a': 3})
intersected_counter = counter1 & counter2
print(intersected_counter) # Output: Counter({'b': 2, 'c': 1})
Updating Counters with Arithmetic Operations
Counters might be up to date utilizing arithmetic operations corresponding to addition, subtraction, multiplication, and division. These operations replace the counts of components within the Counter primarily based on the corresponding operation. For instance:
counter = Counter({'a': 3, 'b': 2, 'c': 1})
counter += Counter({'b': 4, 'c': 2, 'd': 1})
print(counter) # Output: Counter({'a': 3, 'b': 6, 'c': 3, 'd': 1})
counter -= Counter({'b': 2, 'c': 1})
print(counter) # Output: Counter({'a': 3, 'b': 4, 'c': 2, 'd': 1})
Working with Nested Counters
Counters might be nested to symbolize hierarchical information constructions. This enables us to depend components at completely different ranges of granularity. For instance, we will have a Counter of Counters to symbolize the counts of components in numerous classes. For instance:
classes = Counter({
'fruit': Counter({'apple': 3, 'orange': 2}),
'vegetable': Counter({'carrot': 5, 'broccoli': 3}),
})
print(classes['fruit']['apple']) # Output: 3
print(classes['vegetable']['carrot']) # Output: 5
Dealing with Giant Datasets with Counter
Counters are environment friendly for dealing with giant datasets as a result of their optimized implementation. They use a hashtable to retailer the counts, which permits for constant-time entry and modification. This makes Counters appropriate for duties corresponding to counting phrase frequencies in giant texts or analyzing large information. For instance:
textual content = "Lorem ipsum dolor sit amet, consectetur adipiscing elit." * 1000000
counter = Counter(textual content.decrease().cut up())
print(counter.most_common(3))
Customizing Counter Habits
Python’s Counter gives a number of strategies and features to customise its habits. For instance, we will use the weather() methodology to retrieve an iterator over the weather within the Counter, or use the subtract() methodology to subtract counts from one other Counter. Moreover, we will use the most_common() operate to get the most typical components from any iterable. For instance:
counter = Counter({'a': 3, 'b': 2, 'c': 1})
components = counter.components()
print(listing(components)) # Output: ['a', 'a', 'a', 'b', 'b', 'c']
counter.subtract({'a': 2, 'b': 1})
print(counter) # Output: Counter({'a': 1, 'b': 1, 'c': 1})
my_list = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
most_common_elements = Counter(my_list).most_common(2)
print(most_common_elements) # Output: [(1, 3), (2, 3)]
Ideas for Optimizing Efficiency with Python’s Counter
Effectively Counting Giant Datasets
When counting giant datasets, utilizing the Counter’s replace() methodology is advisable as a substitute of making a brand new Counter object for every component. This avoids pointless reminiscence allocation and improves efficiency. For instance:
counter = Counter()
information = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
for component in information:
counter.replace([element])
print(counter)
Selecting the Proper Information Construction
Contemplate the necessities of your process and select the suitable information construction accordingly. Should you solely must depend components, a Counter is an appropriate alternative. Nonetheless, should you want extra functionalities corresponding to sorting or indexing, you could want to make use of different information constructions like dictionaries or lists.
Using Counter Strategies and Features
Python’s Counter gives numerous strategies and features that may assist optimize efficiency. For instance, the most_common() methodology can be utilized to retrieve the most typical components effectively, whereas the weather() methodology can be utilized to iterate over the weather with out creating a brand new listing.
Conclusion
Python’s Counter is a flexible information construction that gives highly effective functionalities for counting, combining, and manipulating information. By understanding the fundamentals of Counters, exploring widespread use circumstances, mastering superior strategies, optimizing efficiency, and following greatest practices, you’ll be able to leverage the complete potential of Python’s Counter in your tasks. Whether or not you’ll want to depend phrase frequencies, discover the most typical components, implement multisets, or monitor stock, Counters supply a handy and environment friendly resolution. So begin utilizing Python’s Counter right now and unlock the facility of counting in your code.