Welcome to the Python Abstraction and Encapsulation MCQs! Abstraction and encapsulation are elementary ideas in object-oriented programming (OOP) that promote code group, reusability, and safety. Abstraction entails hiding pointless particulars and exhibiting solely important options of an object, whereas encapsulation entails bundling the info and strategies that function on the info right into a single unit. These questions will take a look at your understanding of those ideas in Python, together with the right way to create summary lessons, implement encapsulation, and leverage these ideas for environment friendly code design. Every query is multiple-choice, with just one appropriate reply. Take your time to fastidiously learn every query and select the best choice. Let’s delve into the world of Python abstraction and encapsulation collectively!
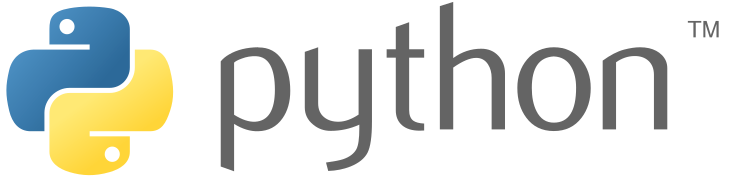
30+ MCQs on Python Abstraction and Encapsulation
Q1. What’s Abstraction in Python?
a) Abstraction is the method of hiding the implementation particulars and exhibiting solely the required options of an object.
b) Abstraction is the method of exposing all of the implementation particulars of an object.
c) Abstraction is the method of making a number of objects from a single class.
d) Abstraction is the method of defining a brand new class from an present class.
Reply: a
Rationalization: Abstraction in Python permits programmers to cover the implementation particulars and present solely the required options of an object to the surface world.
Q2. What’s Encapsulation in Python?
a) Encapsulation is the method of exposing all of the implementation particulars of an object.
b) Encapsulation is the method of mixing knowledge and features right into a single unit referred to as a category.
c) Encapsulation is the method of making a number of objects from a single class.
d) Encapsulation is the method of defining a brand new class from an present class.
Reply: b
Rationalization: Encapsulation in Python is the bundling of information and the strategies that function on that knowledge right into a single unit referred to as a category. It helps in hiding the implementation particulars of an object.
Q3. Which of the next is a bonus of Abstraction?
a) It will increase code reusability
b) It makes this system run quicker
c) It makes the code extra advanced
d) It reduces the necessity for code group
Reply: a
Rationalization: Abstraction will increase code reusability by permitting programmers to reuse the abstracted class or object with out worrying about its implementation particulars.
This fall. Which of the next is a bonus of Encapsulation?
a) It reduces code reusability
b) It makes this system run slower
c) It makes the code much less versatile
d) It prevents knowledge from being accessed by unauthorized code
Reply: d
Rationalization: In Python, encapsulation is used to cover the implementation particulars of an object and to guard the info from unauthorized entry. It improves safety and makes the code extra strong.
Q5. In Python, how do you create an summary class?
a) By utilizing the summary
key phrase
b) By utilizing the abstractmethod
decorator from the abc
module
c) By utilizing the summary
methodology from the abc
module
d) By inheriting from the summary
class
Reply: b
Rationalization: In Python, summary lessons are created utilizing the abstractmethod
decorator from the abc
(Summary Base Lessons) module.
Q6. Which of the next is true about summary strategies in Python?
a) Summary strategies have a physique and have to be applied within the derived class
b) Summary strategies haven’t any physique and have to be applied within the derived class
c) Summary strategies can’t be overridden within the derived class
d) Summary strategies are non-obligatory to implement within the derived class
Reply: b
Rationalization: Summary strategies in Python haven’t any physique and require implementation within the derived (youngster) class. They signify a technique within the dad or mum class that each one youngster lessons should implement.
Q7. What’s the objective of Encapsulation in Python?
a) To make this system run quicker
b) To cover the implementation particulars of an object
c) To create a number of objects from a single class
d) To outline a brand new class from an present class
Reply: b
Rationalization: In Python, encapsulation is used to cover the implementation particulars of an object and to guard the info from unauthorized entry.
Q8. Which key phrase is used to outline a protected variable in Python?
a) personal
b) shield
c) __private
d) _
Reply: d
Rationalization: In Python, protected variables are outlined utilizing a single underscore _
prefix.
Q9. What does it imply for a variable or methodology to be personal in Python?
a) It can’t be accessed from exterior the category
b) It might probably solely be accessed from exterior the category
c) It’s accessible from any class
d) It’s accessible solely throughout the identical module
Reply: a
Rationalization: Non-public variables or strategies in Python can’t be accessed from exterior the category. They’re solely accessible throughout the class itself.
Q10. What’s the good thing about utilizing Encapsulation in Python?
a) It will increase the complexity of the code
b) It makes code much less safe
c) It permits for higher management over class attributes and strategies
d) It makes code much less organized
Reply: c
Rationalization: Encapsulation in Python permits for higher management over class attributes and strategies, resulting in improved safety and maintainability of the code.
Q11. Which of the next is true about Abstraction in Python?
a) It exposes all of the implementation particulars of an object
b) It hides the implementation particulars and exhibits solely the required options of an object
c) It isn’t associated to lessons and objects
d) It makes this system run slower
Reply: b
Rationalization: Abstraction in Python hides the implementation particulars of an object and exhibits solely the required options to the surface world, bettering readability and decreasing complexity.
Q12. What’s the objective of the getter
methodology in Python Encapsulation?
a) To set the worth of a non-public variable
b) To get the worth of a non-public variable
c) To delete a non-public variable
d) To outline a non-public variable
Reply: b
Rationalization: A getter
methodology in Python Encapsulation is used to get the worth of a non-public variable, permitting managed entry to the variable from exterior the category.
Q13. Which of the next finest describes Encapsulation in Python?
a) Combining knowledge and features right into a single unit referred to as a category
b) Exposing all of the implementation particulars of an object
c) Creating a number of objects from a single class
d) Defining a brand new class from an present class
Reply: a
Rationalization: Encapsulation in Python entails combining knowledge and features right into a single unit referred to as a category, permitting for higher group and modularity within the code.
Q14. What’s the objective of a setter
methodology in Python Encapsulation?
a) To set the worth of a non-public variable
b) To get the worth of a non-public variable
c) To delete a non-public variable
d) To outline a non-public variable
Reply: a
Rationalization: A setter
methodology in Python Encapsulation is used to set the worth of a non-public variable, offering managed entry and validation for the variable.
Q15. Which of the next is true about Abstraction in Python?
a) It will increase code complexity
b) It reduces code reusability
c) It helps in code group and administration
d) It exposes all of the implementation particulars
Reply: c
Rationalization: Abstraction in Python helps in code group and administration by hiding the implementation particulars and exhibiting solely the required options of an object.
Q16. Which key phrase defines a non-public methodology in Python?
a) personal
b) shield
c) __private
d) __
Reply: d
Rationalization: In Python, personal strategies are outlined utilizing a double underscore __
prefix.
Q17. Contemplate the next Python code:
class Circle:
def __init__(self, radius):
self.__radius = radius
def get_radius(self):
return self.__radius
def set_radius(self, radius):
if radius > 0:
self.__radius = radius
# Create an occasion of the Circle class
c = Circle(5)
# Change the radius to 10
c.set_radius(10)
# Get the brand new radius
print(c.get_radius())
What would be the output of this code?
a) 5
b) 10
c) 15
d) Error: Can’t entry personal attribute __radius
Reply: b
Rationalization: The set_radius
methodology is used to vary the radius to 10, after which get_radius
methodology is used to get the up to date radius, which is 10.
Q18. Contemplate the next Python code:
class BankAccount:
def __init__(self, stability=0):
self.__balance = stability
def deposit(self, quantity):
if quantity > 0:
self.__balance += quantity
def withdraw(self, quantity):
if quantity > 0 and quantity <= self.__balance:
self.__balance -= quantity
return quantity
else:
return "Inadequate funds"
def get_balance(self):
return self.__balance
# Create an occasion of BankAccount
acc = BankAccount(100)
# Deposit $50
acc.deposit(50)
# Withdraw $70
print(acc.withdraw(70))
# Get the present stability
print(acc.get_balance())
What would be the output of this code?
a) 70, 80
b) 70, 50
c) 50, 80
d) Inadequate funds, 80
Reply: a
Rationalization: Initially, the stability is 100. After depositing 50, the stability turns into 150. After withdrawing 70, the output of withdraw(70)
is 70, and the present stability is 80.
Q19. Contemplate the next Python code:
class Particular person:
def __init__(self, title, age):
self.__name = title
self.__age = age
def get_name(self):
return self.__name
def set_name(self, title):
self.__name = title
def get_age(self):
return self.__age
def set_age(self, age):
self.__age = age
# Create an inventory of Particular person objects
folks = [Person("Alice", 30), Person("Bob", 25), Person("Charlie", 35)]
# Print the names of individuals over 30 years outdated
for individual in folks:
if individual.get_age() > 30:
print(individual.get_name())
What occurs when this code executes?
a) Alice, Bob, Charlie
b) Alice, Charlie
c) Bob, Charlie
d) Alice
Reply: c
Rationalization: The code iterates by the checklist of Particular person
objects and prints the names of these with an age over 30. Solely Bob and Charlie meet this situation, so their names are printed.
Q20. Contemplate the next Python code:
class Worker:
def __init__(self, title, wage):
self.__name = title
self.__salary = wage
def get_name(self):
return self.__name
def get_salary(self):
return self.__salary
def set_salary(self, wage):
if wage > 0:
self.__salary = wage
# Create an Worker object
emp = Worker("John", 50000)
# Set a brand new wage
emp.set_salary(60000)
# Attempt to straight entry the personal attribute __salary
print(emp.__salary)
What’s going to occur when this code is executed?
a) The brand new wage 60000 will likely be printed
b) The outdated wage 50000 will likely be printed
c) Error: AttributeError: ‘Worker’ object has no attribute ‘__salary’
d) Error: TypeError: ‘Worker’ object just isn’t subscriptable
Reply: c
Rationalization: Attempting to straight entry a non-public attribute __salary
exterior of the category will lead to an AttributeError.
Q21. Contemplate the next Python code:
class Rectangle:
def __init__(self, size, width):
self.__length = size
self.__width = width
def space(self):
return self.__length * self.__width
# Create a Rectangle object
rect = Rectangle(5, 10)
# Get the realm
print(rect.space())
# Attempt to straight entry the personal attribute __length
print(rect.__length)
What occurs when this code executes?
a) The realm 50 will likely be printed, adopted by an error
b) The realm 50 will likely be printed, adopted by the worth of __length
c) Error: AttributeError: ‘Rectangle’ object has no attribute ‘__length’
d) Error: TypeError: ‘Rectangle’ object just isn’t subscriptable
Reply: a
Rationalization: The code will print the realm, which is 50. Nevertheless, attempting to straight entry a non-public attribute __length
exterior of the category will lead to an AttributeError.
Q22. Contemplate the next Python code:
class E-book:
def __init__(self, title, writer):
self.__title = title
self.__author = writer
def get_title(self):
return self.__title
def get_author(self):
return self.__author
# Create a E-book object
guide = E-book("Python Programming", "John Doe")
# Attempt to change the title
guide.__title = "New Title"
# Print the title and writer
print(guide.get_title())
print(guide.get_author())
What will likely be printed when this code is executed?
a) Python Programming, John Doe
b) New Title, John Doe
c) Error: AttributeError: ‘E-book’ object has no attribute ‘__title’
d) Error: TypeError: ‘E-book’ object just isn’t subscriptable
Reply: a
Rationalization: The try to vary __title
straight with guide.__title = "New Title"
creates a brand new occasion variable, nevertheless it doesn’t change the personal __title
attribute outlined within the class. So when get_title()
is named, it returns the unique title “Python Programming”.
Q23. Contemplate the next Python code:
class Automotive:
def __init__(self, make, mannequin):
self.__make = make
self.__model = mannequin
def get_make(self):
return self.__make
def get_model(self):
return self.__model
# Create a Automotive object
automotive = Automotive("Toyota", "Camry")
# Print the make and mannequin
print(automotive.get_make())
print(automotive.get_model())
# Attempt to change the make
automotive.__make = "Honda"
# Print the make once more
print(automotive.get_make())
What will likely be printed when this code is executed?
a) Toyota, Camry, Honda
b) Toyota, Camry, Toyota
c) Error: AttributeError: ‘Automotive’ object has no attribute ‘__make’
d) Error: TypeError: ‘Automotive’ object just isn’t subscriptable
Reply: a
Rationalization: The try to vary __make
straight with automotive.__make = "Honda"
creates a brand new occasion variable, nevertheless it doesn’t change the personal __make
attribute outlined within the class. So when get_make()
is named, it returns the unique make “Toyota”.
Q24. Which of the next is NOT a good thing about utilizing Abstraction and Encapsulation?
a) Improved code maintainability
b) Enhanced safety
c) Elevated code complexity
d) Higher code group
Reply: c
Rationalization: Elevated code complexity just isn’t a good thing about Abstraction and Encapsulation. These ideas are designed to simplify code by hiding pointless particulars.
Q25. Which of the next is an instance of Abstraction in Python?
a) Utilizing a for
loop to iterate over an inventory
b) Defining a perform with parameters and a return worth
c) Instantiating an object from a category and calling its strategies
d) Declaring a non-public class attribute with a __
prefix
Reply: c
Rationalization: Instantiating an object from a category and calling its strategies is an instance of Abstraction, because it hides the interior implementation of the category.
Q26. In Python, what’s the significance of utilizing title mangling with double underscore (__) for attributes?
a) It makes attributes accessible from exterior the category
b) It hides attributes from throughout the class
c) It prevents attribute modification from exterior the category
d) It permits subclass strategies to entry dad or mum class attributes
Reply: b
Rationalization: Title mangling with double underscore (__
) in Python is used to make attributes personal, hiding them from exterior the category.
Q27. Which assertion finest describes the connection between Encapsulation and Data Hiding?
a) Encapsulation is identical as Data Hiding
b) Encapsulation is a broader idea that features Data Hiding
c) Data Hiding is a safer type of Encapsulation
d) Data Hiding is a design precept, whereas Encapsulation is a programming approach
Reply: b
Rationalization: Encapsulation is a broader idea that features Data Hiding, because it entails bundling knowledge and strategies collectively, and controlling entry to them.
Q28. Why do programmers suggest utilizing getter and setter strategies for personal attributes in Python?
a) To make the code extra advanced
b) To supply a constant strategy to entry and modify attributes
c) To keep away from utilizing personal attributes altogether
d) To straight entry personal attributes for effectivity
Reply: b
Rationalization: Getter and setter strategies present a managed strategy to entry and modify personal attributes, imposing encapsulation and guaranteeing knowledge integrity.
Q29. Contemplate the next Python code:
class Scholar:
def __init__(self, title, age):
self.__name = title
self.__age = age
def get_name(self):
return self.__name
def get_age(self):
return self.__age
# Create a Scholar object
scholar = Scholar("Alice", 20)
# Try and entry a non-public attribute straight
print(scholar.__name)
What error happens when executing this code?
a) AttributeError: ‘Scholar’ object has no attribute ‘__name’
b) TypeError: ‘Scholar’ object just isn’t subscriptable
c) SyntaxError: invalid syntax
d) No error, it would print “Alice”
Reply: a
Rationalization: Attempting to straight entry a non-public attribute __name
exterior of the category will lead to an AttributeError since personal attributes can’t be accessed straight from exterior the category.
Q30. Which of the next code snippets appropriately defines a Python class referred to as Particular person
with an __init__
methodology to initialize title
and age
attributes?
a)
class Particular person:
def __init__(self, title, age):
self.title = title
self.age = age
b)
class Particular person:
def __init__(self, title, age):
title = title
age = age
c)
class Particular person:
def __init__(self, title, age):
self.title = title
age = age
d)
class Particular person:
def __init__(self, title, age):
self.title = title
self.__age = age
Reply: a
Rationalization: Choice (a) appropriately defines the Particular person
class with self.title
and self.age
attributes initialized within the __init__
methodology.
Q31. What’s going to the next code snippet output?
class Circle:
def __init__(self, radius):
self.radius = radius
def get_area(self):
return 3.14 * self.radius ** 2
c = Circle(5)
print(c.get_area())
a) 78.5
b) 314
c) 25
d) Error: ‘Circle’ object has no attribute ‘get_area’
Reply: a
Rationalization: The get_area
methodology calculates the realm of the circle primarily based on the given radius (5), which is 78.5.
Q32. What does the next code snippet do?
class Rectangle:
def __init__(self, width, peak):
self.width = width
self.peak = peak
def get_area(self):
return self.width * self.peak
r = Rectangle(4, 5)
print(r.get_area())
a) Calculates the perimeter of a rectangle with width 4 and peak 5
b) Calculates the realm of a rectangle with width 4 and peak 5
c) Prints the string illustration of the Rectangle object
d) Raises an error as a result of there is no such thing as a __init__
methodology
Reply: b
Rationalization: The get_area
methodology calculates the realm of the rectangle primarily based on the given width (4) and peak (5), which is 20.
Congratulations on finishing the Python Abstraction and Encapsulation MCQs! Abstraction and encapsulation are important ideas in OOP that assist in designing maintainable, scalable, and safe code. By mastering these ideas, you achieve the flexibility to create clear and concise class buildings, cover implementation particulars, and shield knowledge integrity. Hold working towards and exploring Python’s abstraction and encapsulation functionalities to develop into proficient in designing strong and environment friendly purposes. When you have any questions or need to delve deeper into any matter, don’t hesitate to proceed your studying journey. Pleased coding!
You can too enroll in out free Python Course As we speak!
Learn our extra articles associated to MCQs in Python: