Introduction
Metaprogramming is a captivating facet of software program growth, permitting builders to put in writing packages that manipulate code itself, altering or producing code dynamically. This highly effective method opens up a world of potentialities for automation, code era, and runtime modifications. In Python, metaprogramming with metaclasses isn’t just a function however an integral a part of the language’s philosophy, enabling versatile and dynamic creation of courses, features, and even total modules on the fly. On this article, we’ll focus on the fundamentals of metaprogramming with metaclasses, in Python.
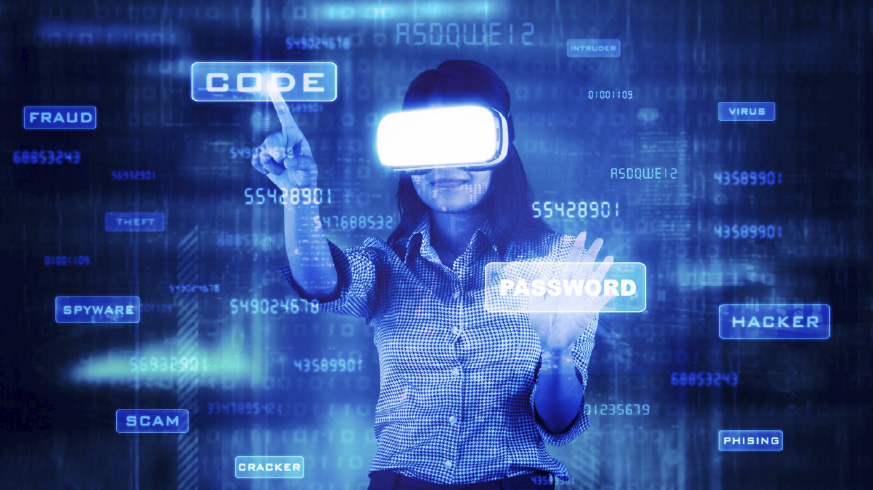
Metaprogramming is about writing code that may produce, modify, or introspect different code. It’s a higher-order programming method the place the operations are carried out on packages themselves. It allows builders to step again and manipulate the elemental constructing blocks of their code, equivalent to features, courses, and even modules, programmatically.
This idea may appear summary at first, however it’s broadly utilized in software program growth for varied functions, together with code era, code simplification, and the automation of repetitive duties. By leveraging metaprogramming, builders can write extra generic and versatile code, lowering boilerplate and making their packages simpler to keep up and prolong.
Additionally Learn: 12 AI Instruments That Can Generate Code To Assist Programmers
Idea of Code that Manipulates Code
To really grasp metaprogramming, it’s important to know that in languages like Python, all the pieces is an object, together with class definitions and features. Which means that courses and features might be manipulated identical to every other object within the language. You possibly can create, modify, or delete them at runtime, enabling dynamic conduct primarily based on this system’s state or exterior inputs.
For example, by way of metaprogramming, a Python script might robotically generate a sequence of features primarily based on sure patterns or configurations outlined at runtime, considerably lowering guide coding efforts. Equally, it might examine and modify the properties of objects or courses, altering their conduct with out altering the unique code instantly.
Python’s design philosophy embraces metaprogramming, offering built-in options that assist and encourage its use. Options like decorators, metaclasses, and the reflection API are all examples of metaprogramming capabilities built-in into the language. These options enable builders to implement highly effective patterns and methods, equivalent to:
- Improve or modify the conduct of features or strategies with out altering their code.
- Customise the creation of courses to implement sure patterns or robotically add performance, enabling superior metaprogramming methods equivalent to Metaprogramming with Metaclasses in Python.
- Study the properties of objects at runtime, enabling dynamic invocation of strategies or entry to attributes.
By these mechanisms, Python builders can write code that’s not nearly performing duties however about governing how these duties are carried out and the way the code itself is structured. This results in extremely adaptable and concise packages that may deal with advanced necessities with elegant options.
Checkout: 5 Wonderful Google Colab Hacks You Ought to Attempt At the moment
Fundamentals of Python Courses and Objects
Python, a powerhouse within the programming world, operates on a easy but profound idea: all the pieces is an object. This philosophy varieties the bedrock of Python’s construction, making understanding courses and objects important for any Python programmer. This text goals to demystify these ideas, delving into the fundamentals of Python courses and objects, the intriguing world of metaclasses, and the way they play a pivotal function in Python’s dynamic nature. Moreover, we’ll discover the fascinating realm of Metaprogramming with Metaclasses in Python, unveiling their capabilities and utilization situations.
Fast Recap of Python Courses and Objects
In Python, a category is a blueprint for creating objects. Objects are situations of courses and encapsulate knowledge and features associated to that knowledge. These features, often known as strategies, outline the behaviors of the item. Courses present a way of bundling knowledge and performance collectively, making a clear, intuitive method to construction software program.
class Canine:
def __init__(self, identify):
self.identify = identify
def converse(self):
return f"{self.identify} says Woof!
On this easy instance, Canine is a category representing a canine, with a reputation attribute and a technique converse that simulates the canine’s bark. Creating an occasion of Canine is simple:
my_dog = Canine("Rex")
print(my_dog.converse()) # Output: Rex says Woof!
Sort Hierarchy in Python
Python’s sort system is remarkably versatile, accommodating all the pieces from primitive knowledge varieties like integers and strings to advanced knowledge constructions. On the prime of this sort hierarchy is the item class, making it the bottom class for all Python courses. This hierarchical construction implies that each Python class is a descendant of this common object class, inheriting its traits.
Courses are Objects Too
An intriguing facet of Python is that courses themselves are objects. They’re situations of one thing referred to as a metaclass. A metaclass in Python is what creates class objects. The default metaclass is sort. This idea may appear recursive, however it’s essential for Python’s dynamic nature, permitting for the runtime creation of courses and even alteration of sophistication conduct.
Need to be taught python for FREE? Enroll in our Introduction to Python Program as we speak!
A metaclass is finest understood because the “class of a category.” It defines how a category behaves. A category defines how an occasion of the category behaves. Consequently, metaclasses enable us to manage the creation of courses, providing a excessive degree of customization in object-oriented programming.
How Metaclasses are Totally different from Courses?
The important thing distinction between a category and a metaclass is their degree of abstraction. Whereas a category is a blueprint for creating objects, a metaclass is a blueprint for creating courses. Metaclasses function at a better degree, manipulating the category itself, not simply situations of the category.
The Default Metaclass in Python: sort
The kind perform is the built-in metaclass Python makes use of by default. It’s versatile, able to creating new courses on the fly. sort can be utilized each as a perform to return the kind of an object and as a base metaclass to create new courses.
Understanding the Sort Perform’s Function in Class Creation
The kind perform performs a pivotal function at school creation. It could possibly dynamically create new courses, taking the category identify, a tuple of base courses, and a dictionary containing attributes and strategies as arguments.
When a category definition is executed in Python, the kind metaclass is known as to create the category object. As soon as the category is created, situations of the category are created by calling the category object, which in flip invokes the __call__ methodology to initialize the brand new object.
The brand new and init Strategies in Metaclasses
Metaclasses can customise class creation by way of the __new__ and __init__ strategies. __new__ is accountable for creating the brand new class object, whereas __init__ initializes the newly created class object. This course of permits for the interception and customization of sophistication creation.
class Meta(sort):
def __new__(cls, identify, bases, dct):
# Customized class creation logic right here
return tremendous().__new__(cls, identify, bases, dct)
Customizing Class Creation with Metaclasses
Metaclasses enable for superior customization of sophistication creation. They will robotically modify class attributes, implement sure patterns, or inject new strategies and properties.
The decision Methodology: Controlling Occasion Creation
The __call__ methodology in metaclasses can management how situations of courses are created, permitting for pre-initialization checks, implementing singleton patterns, or dynamically modifying the occasion.
Metaclasses in Python are a profound but typically misunderstood function. They supply a mechanism for modifying class creation, enabling builders to implement patterns and behaviors that may be cumbersome or unimaginable to realize with customary courses. This text will information you thru Metaprogramming with Metaclasses in Python, demonstrating the way to create customized metaclasses, illustrate this idea with easy examples, and discover sensible use instances the place metaclasses shine.
Step-by-Step Information to Defining a Metaclass
Defining a metaclass in Python entails subclassing from the kind metaclass. Right here’s a simplified step-by-step information to creating your individual metaclass:
- Perceive the sort Metaclass: Acknowledge that sort is the built-in metaclass Python makes use of by default for creating all courses.
- Outline the Metaclass: Create a brand new class, sometimes named with a Meta suffix, and make it inherit from sort. This class is your customized metaclass.
- Implement Customized Conduct: Override the __new__ and/or __init__ strategies to introduce customized class creation conduct.
- Use the Metaclass in a Class: Specify your customized metaclass utilizing the metaclass key phrase within the class definition.
Instance
# Step 2: Outline the Metaclass
class CustomMeta(sort):
# Step 3: Implement Customized Conduct
def __new__(cls, identify, bases, dct):
# Add customized conduct right here. For instance, robotically add a category attribute.
dct['custom_attribute'] = 'Worth added by metaclass'
return tremendous().__new__(cls, identify, bases, dct)
# Step 4: Use the Metaclass in a Class
class MyClass(metaclass=CustomMeta):
cross
# Demonstration
print(MyClass.custom_attribute) # Output: Worth added by metaclass
Attribute Validator Metaclass
This metaclass checks if sure attributes are current within the class definition.
class ValidatorMeta(sort):
def __new__(cls, identify, bases, dct):
if 'required_attribute' not in dct:
elevate TypeError(f"{identify} should have 'required_attribute'")
return tremendous().__new__(cls, identify, bases, dct)
class TestClass(metaclass=ValidatorMeta):
required_attribute = True
Singleton Metaclass
This ensures a category solely has one occasion.
class SingletonMeta(sort):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = tremendous().__call__(*args, **kwargs)
return cls._instances[cls]
class SingletonClass(metaclass=SingletonMeta):
cross
Singleton Sample
The singleton sample ensures {that a} class has just one occasion and gives a world level of entry to it. The SingletonMeta metaclass instance above is a direct software of this sample, controlling occasion creation to make sure solely a single occasion exists.
Class Property Validation
Metaclasses can be utilized to validate class properties at creation time, guaranteeing that sure circumstances are met. For instance, you possibly can implement that each one subclasses of a base class implement particular strategies or attributes, offering compile-time checks relatively than runtime errors.
Automated Registration of Subclasses
A metaclass can robotically register all subclasses of a given class, helpful for plugin programs or frameworks the place all extensions must be found and made obtainable with out express registration:
class PluginRegistryMeta(sort):
registry = {}
def __new__(cls, identify, bases, dct):
new_class = tremendous().__new__(cls, identify, bases, dct)
if identify not in ['BasePlugin']:
cls.registry[name] = new_class
return new_class
class BasePlugin(metaclass=PluginRegistryMeta):
cross
# Subclasses of BasePlugin at the moment are robotically registered.
class MyPlugin(BasePlugin):
cross
print(PluginRegistryMeta.registry) # Output contains MyPlugin
class PluginRegistryMeta(sort):
registry = {}
def __new__(cls, identify, bases, dct):
new_class = tremendous().__new__(cls, identify, bases, dct)
if identify not in ['BasePlugin']:
cls.registry[name] = new_class
return new_class
class BasePlugin(metaclass=PluginRegistryMeta):
cross
# Subclasses of BasePlugin at the moment are robotically registered.
class MyPlugin(BasePlugin):
cross
print(PluginRegistryMeta.registry) # Output contains MyPlugin
Conclusion
Metaclasses are a strong function in Python, permitting for classy manipulation of sophistication creation. By understanding the way to create and use customized metaclasses, builders can implement superior patterns and behaviors, equivalent to singletons, validation, and automated registration. Whereas metaclasses can introduce complexity, their even handed use can result in cleaner, extra maintainable, and extra intuitive code.
Need to grasp python coding? Enroll in our free course to Introduction to Python.