Python is each enjoyable and simple to make use of. With a variety of libraries obtainable, Python makes our lives simpler by simplifying the creation of video games and purposes. On this article, we’ll create a traditional recreation that many people have probably performed sooner or later in our lives – the snake recreation. For those who haven’t skilled this recreation earlier than, now’s your probability to discover and craft your very personal model with out the necessity to set up heavy libraries in your system. All you could get began on making this nostalgic snake recreation is a fundamental understanding of Python and an internet coding editor like repl.it.
The snake recreation is a timeless arcade traditional the place the participant controls a snake that grows in size because it consumes meals. On this implementation, let’s break down the code to know how the sport is structured and the way the Turtle library is used for graphics and consumer interplay.
Utilizing Turtle Graphics for Easy Sport Growth
The Turtle graphics library in Python offers a enjoyable and interactive strategy to create shapes, draw on the display, and reply to consumer enter. It’s typically used for instructional functions, instructing programming ideas by visible suggestions. This code makes use of Turtle to create the sport components such because the snake, meals, and rating show.
On-line coding editor: Repl.it
An internet coding platform known as Repl.it permits you to develop, run, and collaborate on code instantly inside your net browser. It helps many alternative programming languages and has built-in compilers and interpreters along with options like code sharing, model management, and teamwork. Builders extensively use it for studying, quick prototyping, and code sharing as a result of it’s easy to make use of and requires no setup.
Algorithm
Allow us to begin constructing our first recreation with python. For doing so we have to comply with sure steps beneath:
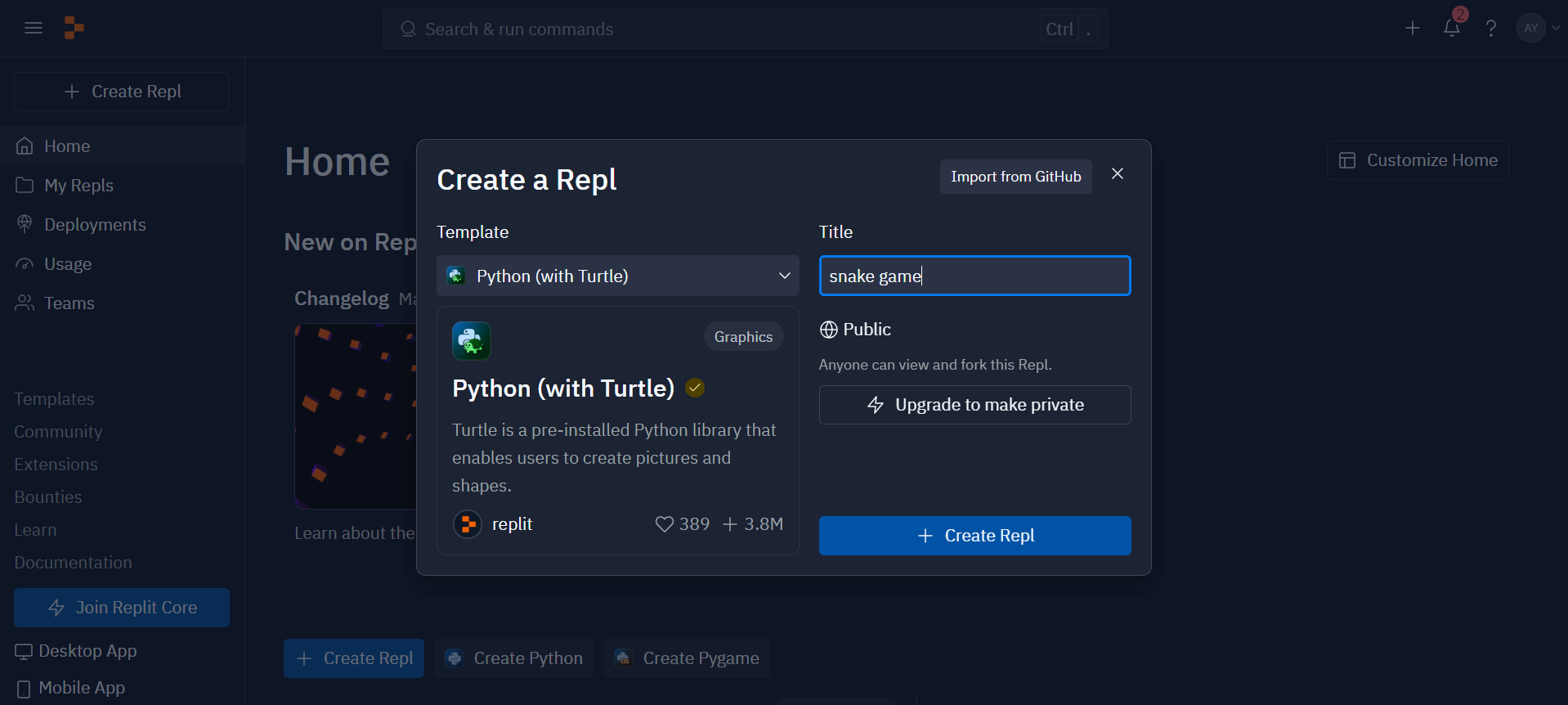
Step1: Putting in the mandatory libraries
This marks the preliminary step in creating the sport often known as Snake. Turtle, random, and time are amongst of those.
- Turtle: We want this library to create the graphics in our recreation. We’re in a position to manipulate the actions of the meals and snake on the display and draw them.
- Random: To create random areas for the meals on the display, we make the most of the random library. This ensures that each time the meals is eaten, it seems in a unique place.
- Time: The snake strikes with a slight delay between every motion because of the time library. This makes the sport simpler to function and extra pleasurable for gamers.
import turtle
import time
import random
Step2: Establishing the sport surroundings.
This contains establishing the display’s dimensions, including a blue background, and including a small delay to make sure fluid gameplay. We additionally arrange variables like high_score to retain the best rating attained, rating to observe the participant’s rating, and segments to trace the snake’s physique.
# Initialize the display
sc = turtle.Display()
sc.bgcolor("blue")
sc.setup(top=1000, width=1000)
delay = 0.1
# Initialize variables
segments = []
rating = 0
high_score = 0
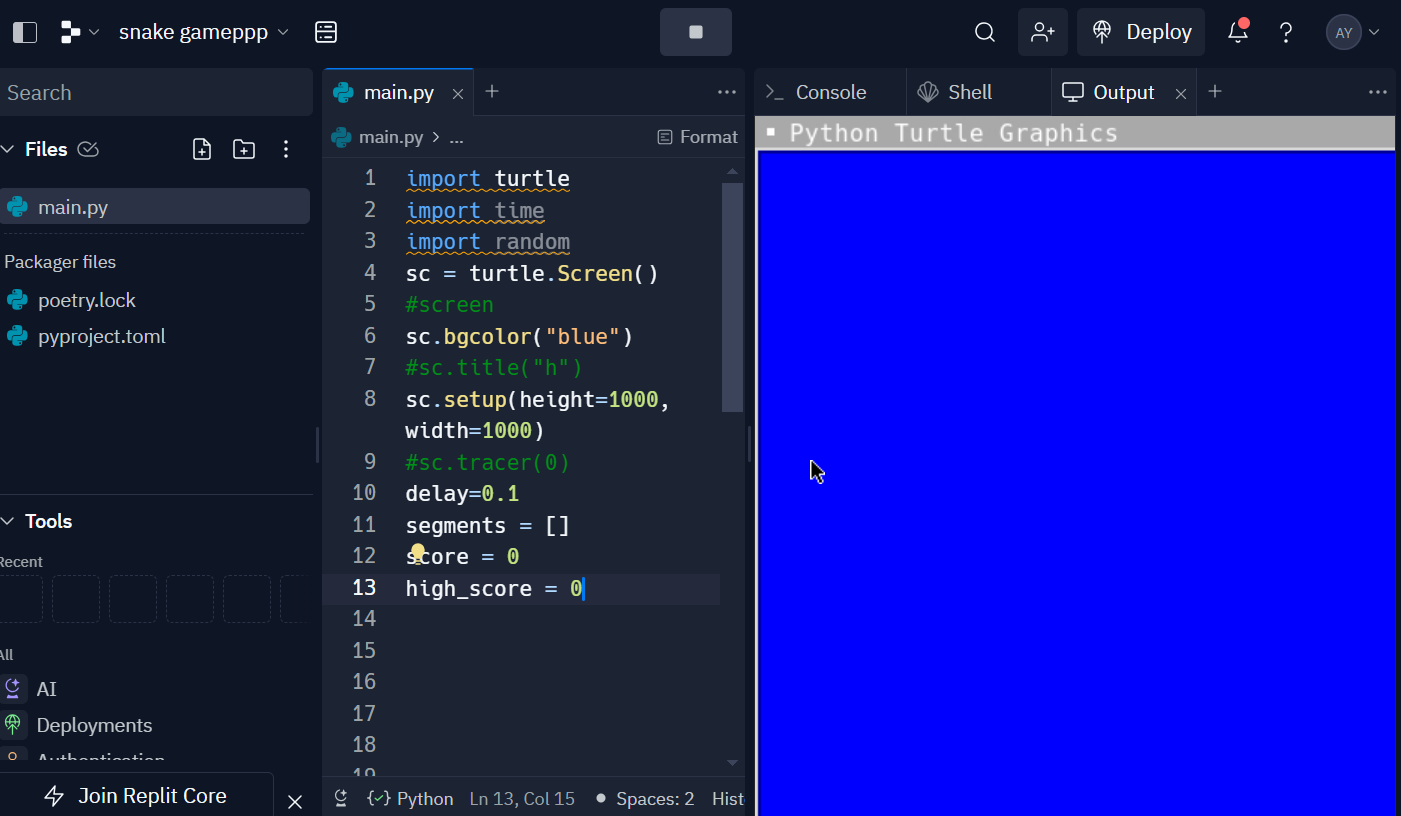
Step3: Creating the snake
A turtle object with a sq. type symbolizes the snake. We find the pen on the heart of the display (goto(0, 100)), set its shade to black, then elevate it to keep away from drawing strains. Initially set to “cease”, the snake’s route stays stationary till the participant begins to maneuver it.
# Create the snake
snake = turtle.Turtle()
snake.form("sq.")
snake.shade("black")
snake.penup()
snake.goto(0, 100)
snake.route = "cease"
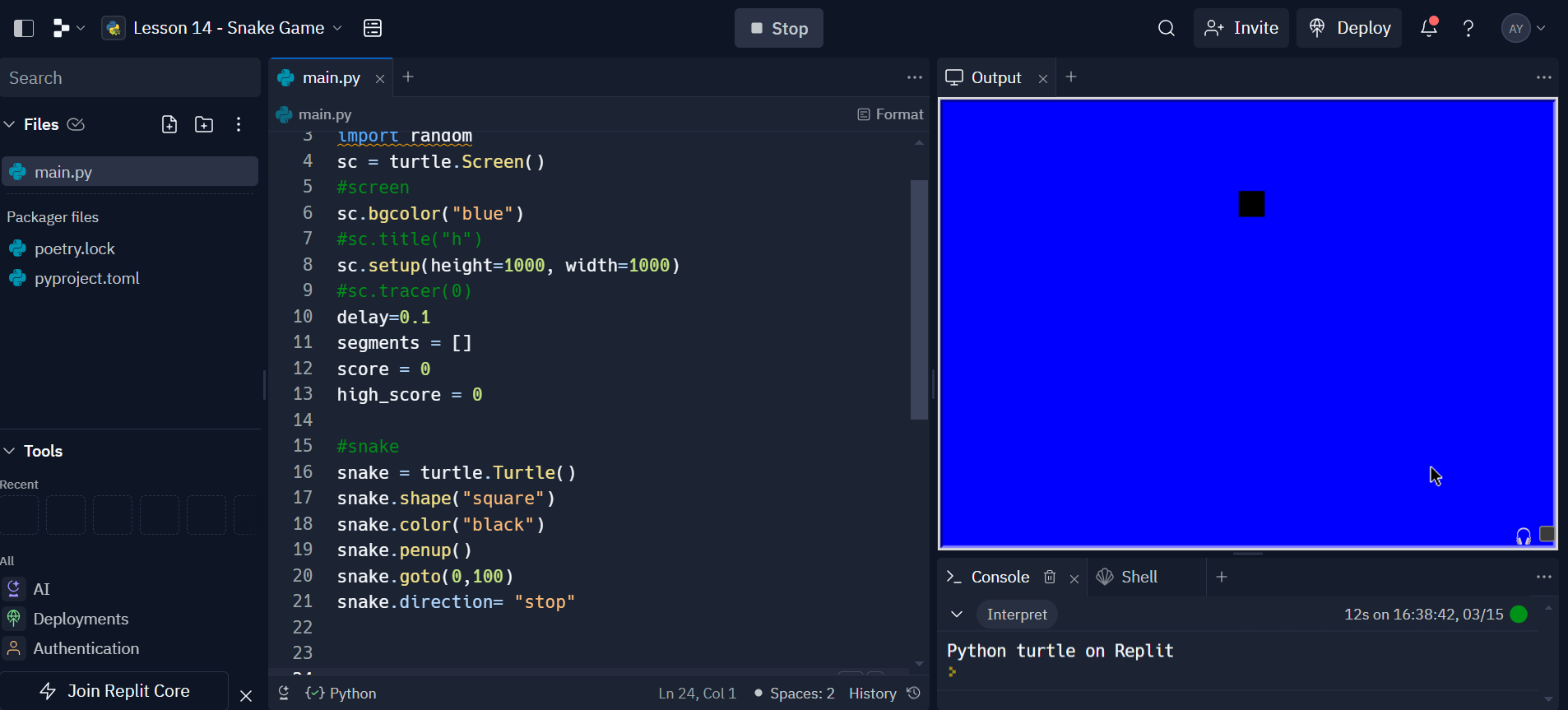
Step4: Motion Capabilities
We outline the snake’s motion features (transfer()) in accordance with its route at that second. These features management the snake’s capability to maneuver up, down, left, and proper. They transfer the pinnacle of the snake 20 items within the acceptable route when requested.
# Capabilities to maneuver the snake
def transfer():
if snake.route == "up":
y = snake.ycor()
snake.sety(y + 20)
if snake.route == "down":
y = snake.ycor()
snake.sety(y - 20)
if snake.route == "left":
x = snake.xcor()
snake.setx(x - 20)
if snake.route == "proper":
x = snake.xcor()
snake.setx(x + 20)
Step5: Controlling the Snake
Utilizing sc.pay attention() and sc.onkey(), we configure key listeners to manage the snake. The associated routines (go_up(), go_down(), go_left(), go_right()) alter the snake’s route in response to key presses on the w, s, a, or d keyboard.
# Capabilities to hyperlink with the keys
def go_up():
snake.route = "up"
def go_down():
snake.route = "down"
def go_left():
snake.route = "left"
def go_right():
snake.route = "proper"
# Hear for key inputs
sc.pay attention()
sc.onkey(go_up, "w")
sc.onkey(go_down, "s")
sc.onkey(go_left, "a")
sc.onkey(go_right, "d")
Step6: Creating the Meals
The meals is represented by a round turtle object with a purple shade. Initially positioned at coordinates (100, 100), it serves because the goal for the snake to eat. When the snake collides with the meals, it “eats” the meals, and a brand new one seems at a random location.
# Create the meals
meals = turtle.Turtle()
meals.form("circle")
meals.shade("purple")
meals.penup()
meals.goto(100, 100)
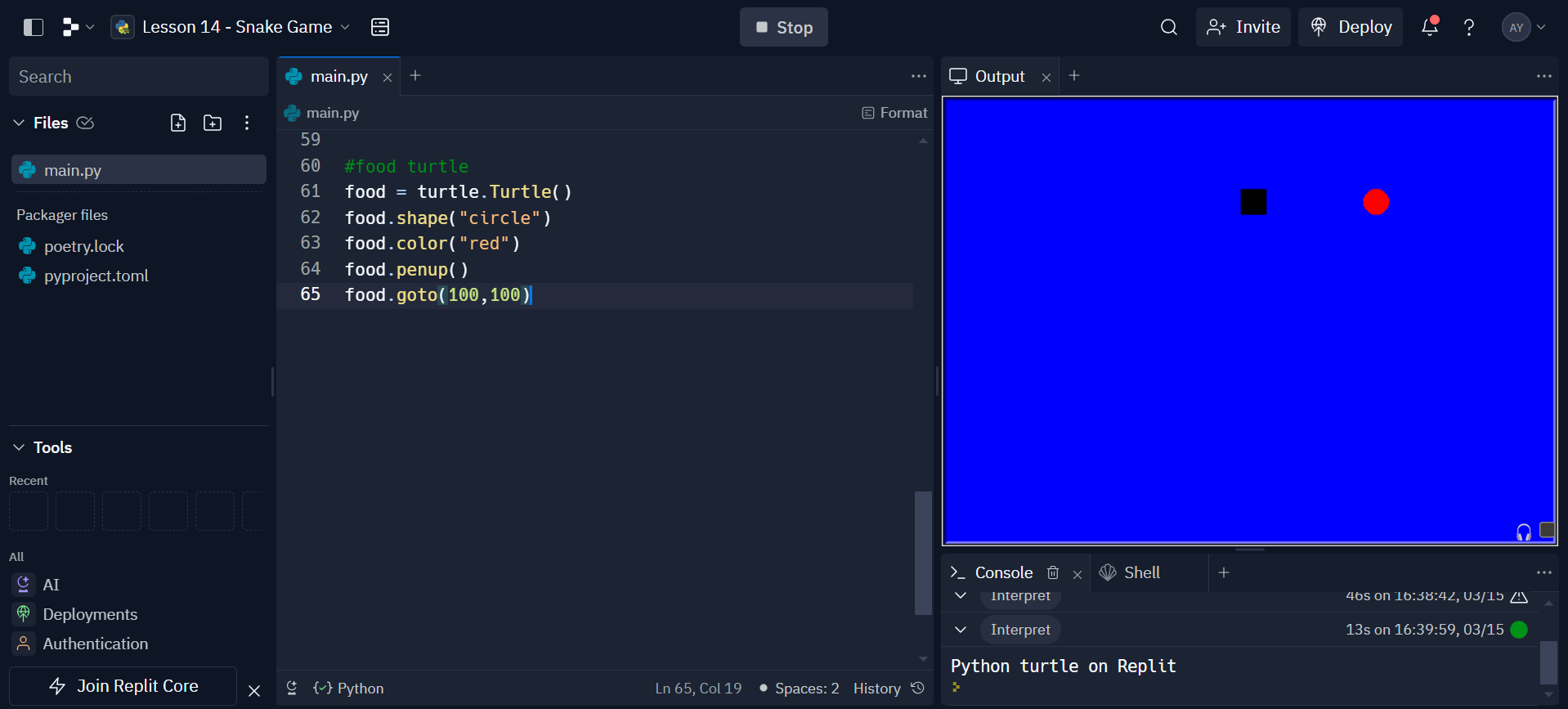
Step7: Displaying Rating
A turtle object (pen) shows the participant’s rating and the best rating achieved. This data updates every time the snake eats the meals.
# Create the rating show
pen = turtle.Turtle()
pen.penup()
pen.goto(0, 100)
pen.hideturtle()
pen.write("Rating: 0 Excessive Rating: 0", align="heart", font=("Arial", 30, "regular")
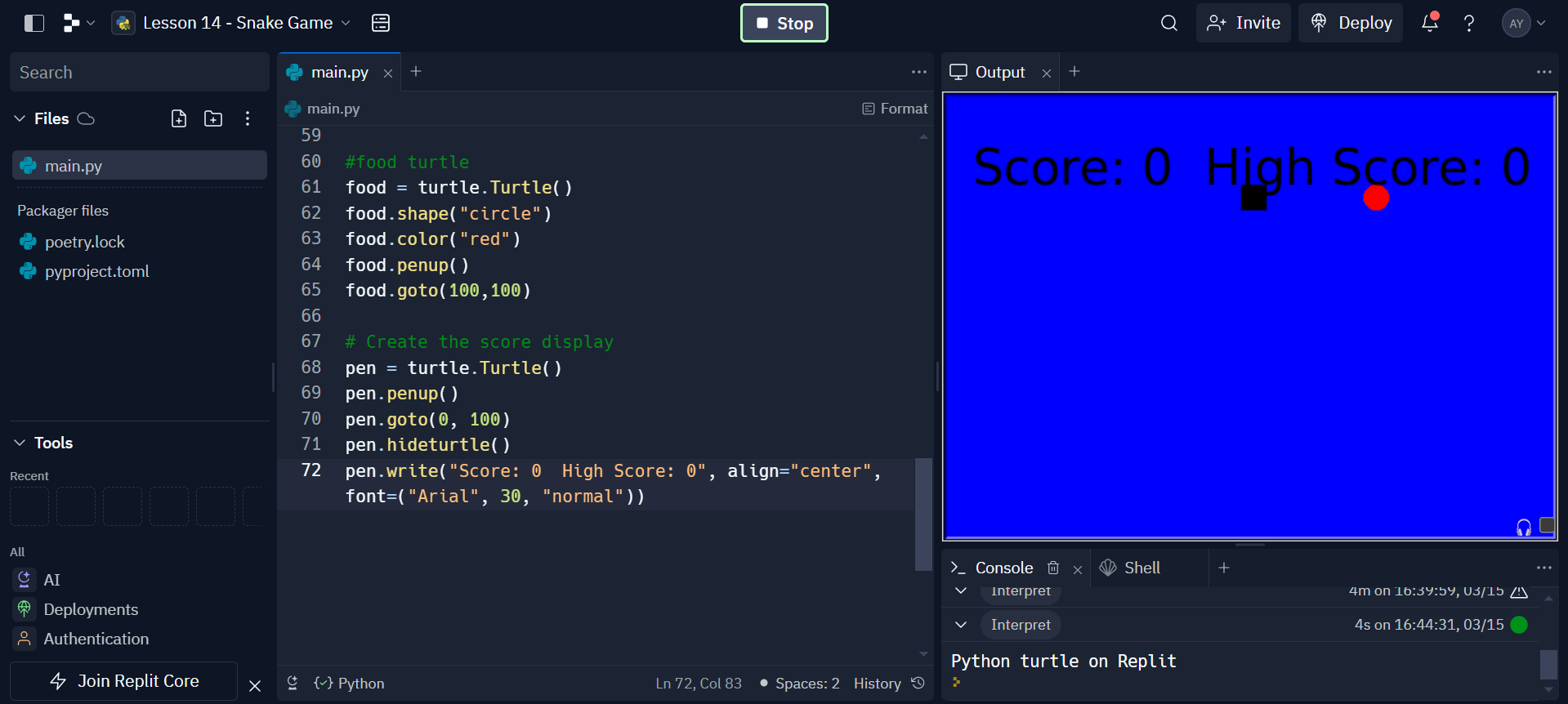
Step8: Most important Sport Loop
The core of the Snake recreation is the first recreation loop. It manages consumer enter, updates the display, strikes the snake, appears for collisions, and regulates how the sport performs out. Let’s look at the precise features of every part of the primary loop in additional element:
whereas True:
sc.replace() # Replace the display
transfer() # Transfer the snake
time.sleep(delay) # Introduce a slight delay for easy gameplay
- sc.replace() : updates the display to mirror any modifications made within the recreation. With out this, the display wouldn’t refresh, and gamers wouldn’t see the snake transfer or the rating replace.
transfer()
: This perform controls the snake’s motion based mostly on its present route. It strikes the snake’s head by 20 items within the route specified by the participant’s enter. The snake constantly strikes within the route it was final directed till the participant modifications its route.- time.sleep(delay): introduces a slight delay between every motion of the snake. The
delay
variable is about to0.1
at the start of the code. The aim of this delay is to manage the velocity of the sport. With out it, the snake would transfer too rapidly for the participant to react, making the sport tough to play.
Consuming Meals and Rising the Snake
if snake.distance(meals) < 20:
x = random.randint(-200, 200)
y = random.randint(-200, 200)
meals.penup()
meals.goto(x, y)
meals.pendown()
# Improve the size of the snake
new_segment = turtle.Turtle()
new_segment.form("sq.")
new_segment.shade("gray")
new_segment.penup()
segments.append(new_segment)
rating += 1
# Replace rating and excessive rating
if rating > high_score:
high_score = rating
pen.clear()
pen.write("Rating: {} Excessive Rating: {}".format(rating, high_score), align="heart", font=("Arial", 30, "regular"))
Consuming Meals
- When the snake’s head (
snake
) comes inside a distance of 20 items from the meals (meals
), it means the snake has “eaten” the meals. - The meals’s place is then reset to a brand new random location on the display utilizing
random.randint()
. - This motion generates the phantasm of the meals “reappearing” in several spots every time it’s eaten.
Rising the snake physique
- When the snake eats the meals, the code provides a brand new phase to the snake’s physique to make it develop.
- A brand new turtle object (
new_segment
) is created, which turns into a part of thesegments
listing. - This listing retains monitor of all of the segments that make up the snake’s physique.
- The participant’s rating is incremented (
rating += 1
) every time the snake eats meals.
Rating Updation
- After consuming meals and updating the rating, the rating show is up to date utilizing the
pen
object. - The
pen.clear()
perform clears the earlier rating show. - Then, the up to date rating and excessive rating are written to the display utilizing
pen.write()
.
Transferring the Snake’s Physique
for i in vary(len(segments) - 1, 0, -1):
x = segments[i - 1].xcor()
y = segments[i - 1].ycor()
segments[i].goto(x, y)
# Transfer the primary phase to comply with the pinnacle
if len(segments) > 0:
x = snake.xcor()
y = snake.ycor()
segments[0].goto(x, y)
- This part of code is liable for making the snake’s physique comply with its head.
- It iterates by the
segments
listing, ranging from the final phase (len(segments) - 1
) all the way down to the second phase (0
), shifting every phase to the place of the phase in entrance of it. - This creates the impact of the snake’s physique following the pinnacle because it strikes.
Updating the First Phase (Head)
- After shifting all of the physique segments, the place of the primary phase (the pinnacle) is up to date to match the present place of the snake (
snake
). - This ensures that the snake’s head continues to guide the physique because it strikes.
Finish Notice
This code offers a fundamental construction for a Snake recreation utilizing the Turtle graphics library. The sport entails controlling a snake to eat meals and develop longer whereas avoiding collisions with partitions and its personal tail. It’s an amazing newbie challenge for studying about recreation improvement ideas, fundamental Python programming, and dealing with consumer enter and graphics. Be happy to customise and develop upon this code so as to add options like collision detection, rising issue, or including ranges to make your Snake recreation much more thrilling!
If you wish to be taught extra about python then enroll in our free python course.
Additionally learn our extra articles associated to python right here:
Podcast: Play in new window | Obtain