Introduction
Embark on an exhilarating journey into the area of Convolutional Neural Networks (CNNs) and Skorch, a revolutionary fusion of PyTorch’s deep studying prowess and the simplicity of scikit-learn. Discover how CNNs emulate human visible processing to crack the problem of handwritten digit recognition whereas Skorch seamlessly integrates PyTorch into machine studying pipelines. Be part of us as we resolve the mysteries of superior deep studying strategies and discover the facility of CNNs for real-world purposes.
Studying Outcomes
- Achieve a deep understanding of Convolutional Neural Networks and their software in handwritten digit recognition.
- Learn the way Skorch bridges PyTorch’s deep studying capabilities with scikit-learn’s user-friendly interface.
- Uncover the structure of CNNs, together with convolutional layers, pooling layers, and totally related layers.
- Discover sensible strategies for coaching and evaluating CNN fashions utilizing Skorch and PyTorch.
- Grasp important abilities in knowledge preprocessing, mannequin definition, hyperparameter tuning, and mannequin persistence for CNN-based duties.
- Purchase insights into superior deep studying ideas akin to hyperparameter optimization, cross-validation, knowledge augmentation, and ensemble studying.
This text was revealed as part of the Knowledge Science Blogathon.
Overview of Convolutional Neural Networks (CNNs)
Image your self sifting by means of a stack of scribbled numbers. Precisely figuring out and classifying every digit is your job; whereas this may increasingly appear straightforward for people, it might be actually tough for machines. That is the elemental subject within the discipline of synthetic intelligence, that’s, handwritten digit recognition.
So as to deal with this subject utilizing machines, researchers have utilized Convolutional Neural Networks (CNNs), a sturdy class of deep studying fashions that draw inspiration from the advanced human visible system. CNNs resemble how layers of neurons in our brains analyze visible knowledge, figuring out objects and patterns at varied scales.
Convolutional layers, the brains of CNNs, search enter knowledge for distinctive traits like edges, corners, and textures. Stacking these layers permits CNNs to study summary representations, capturing hierarchical patterns for purposes like digital quantity identification.
CNNs use convolutions, pooling layers, down sampling, and backpropagation to scale back spatial dimension and enhance computing effectivity. They’ll acknowledge handwritten numbers with precision, typically outperforming standard algorithms. CNNs open the door to a future the place robots can decode and perceive handwritten numbers utilizing deep studying, mimicking human imaginative and prescient’s complexities.
What’s Skorch and Its Advantages ?
With its intensive library and framework ecosystem, Python has emerged as the popular language for configuring deep studying fashions. TensorFlow, PyTorch, and Keras are a couple of well-known frameworks that give programmers a set of chic instruments and APIs for successfully creating and coaching CNN fashions.
Each framework has its personal distinctive advantages and options that meet the wants and tastes of varied builders.
PyTorch’s success is attributed to its “define-by-run” semantics, which dynamically creates the computational graph throughout operations, enabling extra environment friendly debugging, mannequin customization, and quicker prototyping.
Skorch connects PyTorch and scikit-learn, permitting builders to make use of PyTorch’s deep studying capabilities whereas utilizing the user-friendly scikit-learn API. This enables builders to combine deep studying fashions into their present machine studying pipelines.
Skorch is a wrapper that integrates with scikit-learn, permitting builders to make use of PyTorch’s neural community modules for coaching, validating, and making predictions. It helps options like grid search, cross-validation, and mannequin persistence, permitting builders to maximise their present information and workflows. Skorch is simple to make use of and adaptable, permitting builders to make use of PyTorch’s deep studying capabilities with out intensive coaching. This mix affords alternatives to create superior CNN fashions and implement them in sensible eventualities.
Easy methods to Work with Skorch?
Allow us to now undergo some steps on find out how to set up Skorch and construct a CNN Mannequin:
Step1: Putting in Skorch
We are going to use the pip command to put in the Skorch library. It’s required solely as soon as.
The fundamental command to put in a package deal utilizing pip is:
pip set up skorch
Alternatively, use the next command inside Jupyter Pocket book/Colab:
!pip set up skorch
Step2: Constructing a CNN mannequin
Be happy to make use of the supply code obtainable right here.
The very first step in coding is to import the mandatory libraries. We would require NumPy, Scikit-learn for dataset dealing with and preprocessing, PyTorch for constructing and coaching neural networks, torch imaginative and prescient
for performing picture transformations as we’re coping with picture knowledge, and Skorch, in fact, for integration of Pytorch with Scikit-learn.
print('Importing Libraries... ',finish='')
import numpy as np
from sklearn.datasets import fetch_openml
from sklearn.model_selection import train_test_split
from skorch import NeuralNetClassifier
from skorch.callbacks import EarlyStopping
from skorch.dataset import Dataset
import torch
from torch import nn
import torch.nn.purposeful as F
import matplotlib.pyplot as plt
import random
print('Executed')
Step3: Understanding the Knowledge
The dataset we selected is known as the USPS digit dataset. It’s a assortment of 9,298 grayscale samples. These samples are routinely scanned from envelopes by the U.S. Postal Service. Every pattern is a 16×16 pixel picture.
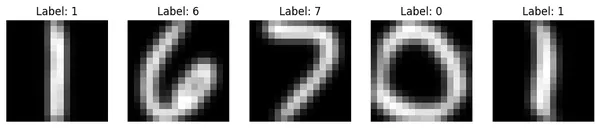
This dataset is freely obtainable at OpenML for experimentation. We are going to use Scikit-learn’s fetch_openml methodology to load the dataset and print the dataset statistics.
# Loading the info
print('Loading knowledge... ',)
X, y = fetch_openml('usps', return_X_y=True)
print('Executed')
# Get dataset statistics
print('Dataset statistics... ')
print(X.form,y.form)
Subsequent, we’ll carry out normal knowledge preprocessing adopted by standardization. Subsequent, we’ll cut up the dataset within the ratio of 70:30 for coaching and testing, respectively.
# Preprocessing
X = X / 16.0 # Scale the enter to [0, 1] vary
X = X.values.reshape(-1, 1, 16, 16).astype(np.float32) # Reshape for CNN enter
y = y.astype('int')-1
# Cut up train-test knowledge in 70:30
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=11)
Defining CNN Structure Utilizing PyTorch
Our CNN mannequin consists of three convolution blocks and two totally related layers. The convolutional layers are stacked to extract the options hierarchically, whereas the totally related layers, typically known as dense layers, are used to carry out the classification job. For the reason that convolution operation generates excessive dimensional knowledge, pooling is carried out to downsize it. Max pooling is among the most used operations, which we now have used. A kernel of dimension 3×3 is used with stride=1. Padding preserves the data on the edges; therefore, padding of dimension one is used. Every layer applies the ReLU activation operate apart from the output layer.
To maintain the mannequin easy, we aren’t utilizing batch normalization. Nonetheless, one might want to use it. To stop overfitting, we use dropout and early stopping.
# Outline CNN mannequin
class DigitClassifier(nn.Module):
def __init__(self):
tremendous(DigitClassifier, self).__init__()
self.conv1 = nn.Conv2d(1, 32, kernel_size=3, padding=1)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3, padding=1)
self.conv3 = nn.Conv2d(64, 128, kernel_size=3, padding=1)
self.fc1 = nn.Linear(128 * 4 * 4, 256)
self.dropout = nn.Dropout(0.2)
self.fc2 = nn.Linear(256, 10)
def ahead(self, x):
x = F.relu(self.conv1(x))
x = F.max_pool2d(x, 2)
x = F.relu(self.conv2(x))
x = F.max_pool2d(x, 2)
x = F.relu(self.conv3(x))
x = x.view(-1, 128 * 4 * 4)
x = F.relu(self.fc1(x))
x = self.dropout(x)
x = self.fc2(x)
return x
Utilizing Skorch to Encapsulate CNN Mannequin
Now comes the central half: find out how to wrap the PyTorch mannequin in Skorch for Sckit-learn type coaching.
For this goal, allow us to outline the hyperparameters as:
# Hyperparameters
max_epochs = 25
lr = 0.001
batch_size = 32
endurance = 5
machine="cuda" if torch.cuda.is_available() else 'cpu'
Subsequent, this code creates a wrapper round a neural community mannequin known as DigitClassifier utilizing Skorch. The wrapped mannequin is configured with settings akin to the utmost variety of coaching epochs, studying fee, batch dimension for coaching and validation knowledge, loss operate, optimizer, early stopping callback, and the machine to run the computations, that’s, CPU or GPU.
# Wrap the mannequin in Skorch NeuralNetClassifier
digit_classifier = NeuralNetClassifier(
module = DigitClassifier,
max_epochs = max_epochs,
lr = lr,
iterator_train__batch_size = batch_size,
iterator_train__shuffle = True,
iterator_valid__batch_size = batch_size,
iterator_valid__shuffle = False,
criterion = nn.CrossEntropyLoss,
optimizer = torch.optim.Adam,
callbacks = [EarlyStopping(patience=patience)],
machine = machine
)
Code Evaluation
Allow us to dig into the code with a radical evaluation:
- Skorch, a wrapper for PyTorch that manages neural community fashions, comprises the `NeuralNetClassifier` class as one in every of its parts. It permits for utilizing PyTorch fashions in a user-friendly interface just like scikit-learn, making the coaching and analysis of neural networks simpler.
- The `module` parameter signifies the neural community mannequin that shall be employed. On this explicit occasion, the PyTorch module “DigitClassifier” encapsulates the definition of the CNN’s structure and performance.
- The `max_epochs` parameter units the higher restrict on the variety of epochs for coaching the neural community.
- The `lr` parameter controls the educational fee, which determines the step dimension throughout optimization. The step dimension is significant in fine-tuning the mannequin’s parameters and decreasing the loss operate.
- The parameters `iterator_train__batch_size` and `iterator_valid__batch_size` are liable for setting the batch dimension for the coaching and validation knowledge, respectively. The batch dimension determines the variety of samples processed earlier than updating the mannequin’s parameters.
- The parameters `iterator_train__shuffle` and `iterator_valid__shuffle` decide how the coaching and validation datasets are shuffled earlier than every epoch. Reorganizing the info helps defend the mannequin from memorizing the order of the samples.
- The parameter
optimizer = torch.optim.Adam
determines the optimizer that can replace the mannequin’s parameters with the calculated gradients. - The `callbacks` parameter consists of utilizing callbacks throughout coaching. Within the instance, EarlyStopping is used to cease coaching early if the validation loss stops bettering inside a set variety of epochs (on this instance, endurance=5).
- The ‘machine’ parameter specifies the machine, akin to CPU or GPU, on which the computations shall be executed.
# Practice the mannequin
print('Utilizing...', machine)
print("Coaching began...")
digit_classifier.match(X_train, y_train)
print("Coaching accomplished!")
# Consider the mannequin
# Consider on take a look at knowledge
y_pred = digit_classifier.predict(X_test)
accuracy = digit_classifier.rating(X_test, y_test)
print(f'Check accuracy: {accuracy:.4f}')
Subsequent, practice the mannequin utilizing the Scikit-learn type match operate. Our mannequin achieves greater than 96% accuracy on take a look at knowledge.
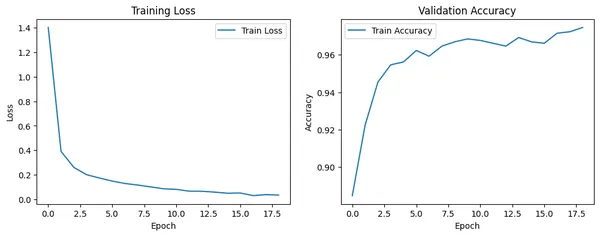
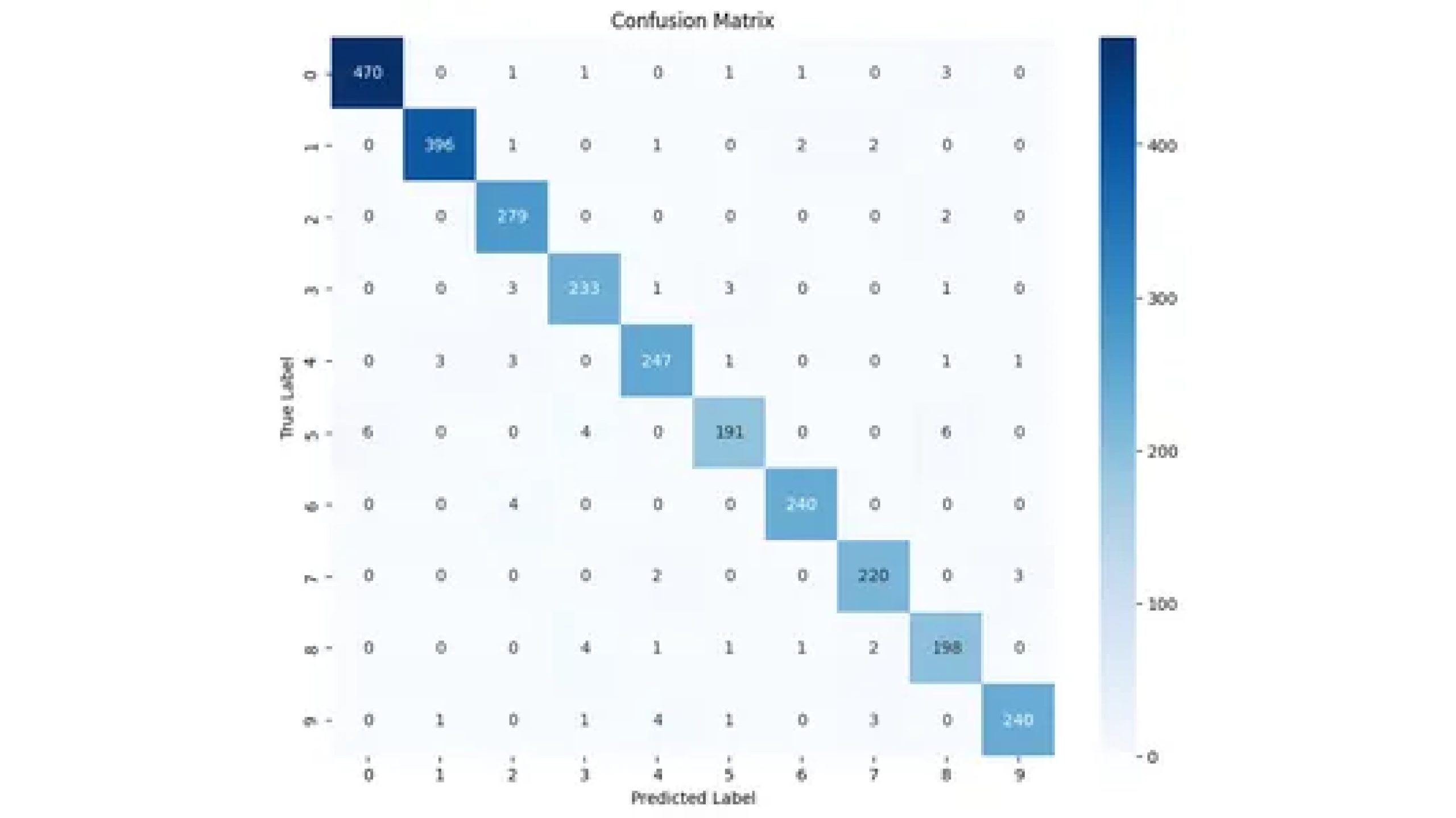
Further Experiments
The above code consists of a easy CNN mannequin. Nonetheless, it’s possible you’ll take into account incorporating the next facets to make sure a extra complete strategy.
Hyperparameters
Hyperparameters regulate how a machine-learning mannequin trains. Correctly tuning them can have a major influence on the efficiency of the mannequin. Make use of varied strategies to optimize hyperparameters, together with grid search or random search. These strategies may help fine-tune studying fee, batch dimension, community structure, and different tunable parameters and return an optimum mixture of hyperparameters.
Cross-Validation
Cross-validation is a beneficial approach for enhancing the reliability of mannequin efficiency analysis. It includes dividing the dataset into a number of subsets and coaching the mannequin on varied combos of those subsets. Carry out k-fold cross-validation to guage the mannequin’s efficiency extra successfully.
Mannequin Persistence
Mannequin persistence entails the method of saving the educated mannequin to disk for future reuse, eliminating the necessity for retraining. By using instruments akin to joblib or torch.save, conducting this job turns into comparatively simple.
Logging and Monitoring
Protecting observe of essential data throughout the coaching course of, akin to loss and accuracy metrics, is essential. There are instruments obtainable that may help in visualizing coaching metrics, akin to TensorBoard or Weights & Biases (wandb).
Knowledge Augmentation
Deep studying fashions rely closely on knowledge. The supply of coaching knowledge straight influences efficiency. Knowledge augmentation includes producing new coaching samples by making use of transformations
to present ones, akin to rotations, translations and flips.
Ensemble Studying
Ensemble studying is a way that leverages the facility of a number of fashions to boost total efficiency. One technique is to coach a number of fashions utilizing varied initializations or subsets of the info after which common their predictions. Discover ensemble strategies akin to bagging or boosting
to boost efficiency by coaching a number of fashions and merging their predictions.
Conclusion
W explored into Convolutional Neural Networks and Skorch reveals the highly effective synergy between superior deep studying strategies and environment friendly Python frameworks. By leveraging CNNs for handwritten digit recognition and Skorch for seamless integration with scikit-learn, we’ve demonstrated the potential to bridge cutting-edge know-how with user-friendly interfaces. This journey underscores the transformative influence of mixing PyTorch’s strong capabilities with scikit-learn’s simplicity, empowering builders to implement subtle fashions with ease. As we navigate by means of the realms of deep studying and machine studying, the collaboration between CNNs and Skorch heralds a future the place advanced duties turn out to be accessible and options turn out to be attainable.
Key Takeaways
- Realized Skorch facilitates seamless integration of PyTorch fashions into Scikit-learn workflows, optimizing productiveness in machine studying duties.
- With Skorch, customers can harness PyTorch’s deep studying capabilities throughout the acquainted and environment friendly atmosphere of Scikit-learn.
- Skorch bridges the hole between PyTorch’s flexibility and Scikit-learn’s ease of use, providing a robust instrument for coaching advanced fashions.
- By leveraging Skorch, builders can practice and deploy PyTorch fashions utilizing Scikit-learn’s strong ecosystem and intuitive API.
- Skorch permits the coaching of PyTorch fashions with Scikit-learn’s grid search, cross-validation, and mannequin persistence functionalities, enhancing mannequin efficiency and reliability.
References
Continuously Requested Questions
A. Skorch is a Python library that seamlessly integrates PyTorch with Scikit-learn, permitting customers to coach PyTorch fashions utilizing Scikit-learn’s acquainted interface and instruments.
A. Skorch supplies a wrapper for PyTorch fashions, enabling customers to make the most of Scikit-learn’s strategies akin to match
, predict
, and rating
for coaching, analysis, and prediction duties.
A. Skorch simplifies the method of constructing and coaching PyTorch fashions by offering a higher-level interface just like Scikit-learn. This makes it simpler for customers aware of Scikit-learn to transition to PyTorch.
A. Sure, Skorch seamlessly integrates with present Scikit-learn workflows, permitting customers to include PyTorch fashions into their machine studying pipelines with out vital modifications.
A. Sure, Skorch helps hyperparameter tuning and cross-validation utilizing Scikit-learn’s instruments akin to GridSearchCV
and RandomizedSearchCV
, enabling customers to optimize their PyTorch fashions effectively.
The media proven on this article just isn’t owned by Analytics Vidhya and is used on the Creator’s discretion.