Introduction
Embark on an thrilling journey into the world of easy machine studying with “Query2Model”! This progressive weblog introduces a user-friendly interface the place complicated duties are simplified into plain language queries. Discover the fusion of pure language processing and superior AI fashions, remodeling intricate duties into easy conversations. Be a part of us as we delve into the HuggingChat chatbot, develop end-to-end mannequin coaching pipelines, leverage AI-powered chatbots for streamlined coding, and unravel the longer term implications of this groundbreaking know-how.
Studying Goals
- Immerse your self on the earth of HuggingChat, a game-changing AI chatbot redefining consumer interplay.
- Navigate the intricacies of mannequin coaching pipelines effortlessly utilizing intuitive pure language queries.
- Discover the horizon of AI chatbot know-how, uncovering its future implications and potential developments.
- Uncover progressive immediate engineering methods for seamless code technology and execution.
- Embrace the democratization of machine studying, empowering customers with accessible interfaces and automation.
This text was printed as part of the Information Science Blogathon.
What’s HuggingChat?
Hugging Chat is an open-source AI-powered chatbot that has been designed to revolutionize the way in which we work together with know-how. With its superior pure language processing capabilities, Hugging Chat affords a seamless and intuitive conversational expertise that feels extremely human-like. Considered one of its key strengths lies in its means to grasp and generate contextually related responses, guaranteeing that conversations move naturally and intelligently. Hugging Chat’s underlying know-how relies on giant language fashions, which have been skilled on huge quantities of textual content information, enabling it to understand a variety of subjects and supply informative and fascinating responses.
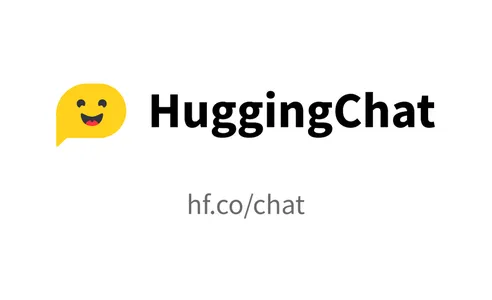
It might help customers in producing code snippets based mostly on their prompts, making it a useful instrument for builders and programmers. Whether or not it’s offering code examples, explaining syntax, or providing options to varied challenges, Hugging Chat’s code technology characteristic enhances its versatility and utility. Moreover, Hugging Chat prioritizes consumer privateness and information safety, guaranteeing confidential and safe conversations. It adheres to moral AI practices, refraining from storing consumer info or conversations, thus offering customers with peace of thoughts and management over their private information.
Unofficial HuggingChat Python API is on the market right here.
What’s Pipeline?
A pipeline refers to a sequence of information processing parts organized in a particular order. Every element within the pipeline performs a specific job on the info, and the output of 1 element turns into the enter of the following. Pipelines are generally used to streamline the machine studying workflow, permitting for environment friendly information preprocessing, characteristic engineering, mannequin coaching, and analysis. By organizing these duties right into a pipeline, it turns into simpler to handle, reproduce, and deploy machine studying fashions.
The pipeline is as follows:
- Textual content Question: Consumer queries the system with all the necessities specified
- Request: Question is restructured and the request is shipped to HuggingChat API(unofficial)
- HuggingChatAPI: Processes the question and generates related code
- Response: Generated code is obtained by consumer as response
- Execution: Resultant Python code is executed to get desired output
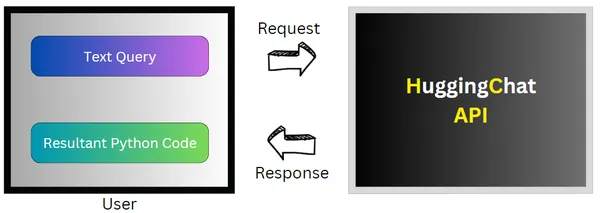
Step-by Step Implementation of Query2Model
Allow us to now look into the step-by-step implementation of Query2Model:
Step1. Import Libraries
Allow us to begin by importing the next libraries:
- sklearn: versatile machine studying library in Python, providing a complete suite of instruments for information preprocessing, mannequin coaching, analysis, and deployment.
- pandas: highly effective information manipulation and evaluation library in Python, designed to simplify the dealing with of knowledge effectively.
- hugchat: unofficial HuggingChat Python API, extensible for chatbots and many others.
!pip set up hugchat
import sklearn
import pandas as pd
from hugchat import hugchat
from hugchat.login import Login
Step2. Defining Query2Model Class
Formatting immediate is used to construction the output in desired format. It consists of a number of pointers reminiscent of printing outcomes if wanted, together with indentations, guaranteeing error-free code, and many others., to make sure the output from the chatbot accommodates solely executable code with out errors when handed to the exec() perform.
#formatting_prompt is to make sure that the response accommodates solely the required code
formatting_prompt = """Error-free code
Retailer the variable names in variables for future reference.
Print the consequence if required
Code ought to be effectively indented with areas, and many others., mustn't comprise importing libraries, feedback.
No loops.
Output ought to be executable with out errors when it's handed to exec() perform"""
The Query2Model class is a instrument for executing consumer queries inside a particular atmosphere. It requires the consumer’s electronic mail and password for authentication, units a cookie storage listing, and initializes a Login object. After profitable authentication, it retrieves and saves cookies, initializing a ChatBot object for interplay. The execute_query() methodology executes consumer queries, returning the consequence as a string.
class Query2Model:
def __init__(self, electronic mail, password):
self.electronic mail = electronic mail
self.password = password
self.cookie_path_dir = "./cookies/"
self.signal = Login(EMAIL, PASSWD)
self.cookies = signal.login(cookie_dir_path=cookie_path_dir, save_cookies=True)
self.chatbot = hugchat.ChatBot(cookies=cookies.get_dict())
# perform to execute the consumer's question
def execute_query(self, question):
query_result = self.chatbot.chat(question+formatting_prompt)
exec(str(query_result))
return str(query_result)
Consumer wants to offer the login credentials of HuggingFace account for authentication
consumer = Query2Model(electronic mail="electronic mail", password="password")
Step3. Information Preparation and Preprocessing
Question consists of path to the dataset(right here the dataset is current in present working listing), the variable to retailer it upon studying, and to show the primary 5 rows.
question= r"""Learn the csv file at path: iris.csv into df variable and show first 5 rows"""
output_code= consumer.execute_query( question )
print(output_code, sep="n")
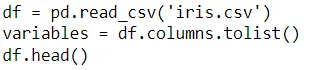
Separating the enter options(X) and label(y) into separate dataframes. Options consists of sepal size& width, petal size& width which signify the traits of iris flower. Label denotes which species the flower belongs to.
question= r"""Retailer 'SepalLengthCm', 'SepalWidthCm', 'PetalLengthCm', 'PetalWidthCm' in X
and 'Species' in y"""
output_code= consumer.execute_query( question )
print(output_code, sep="n")

Dividing 80% of knowledge for coaching and 20% of knowledge for testing with a random state of 111
question= r"""Divide X, y for coaching and testing with 80-20% with random_state=111"""
output_code= consumer.execute_query( question )
print(output_code, sep="n")

Making use of normal scaler method to normalize the info. It transforms the info by eradicating the imply and scaling it to unit variance, guaranteeing that every characteristic has a imply of 0 and a regular deviation of 1.
question= r"""Apply normal scaler"""
output_code= consumer.execute_query( question )
print(output_code, sep="n")
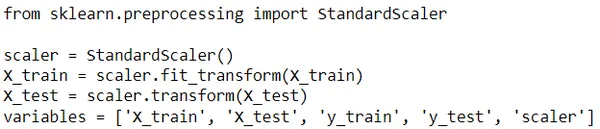
Step4. Mannequin Coaching and Analysis
Question accommodates directions to coach a random forest classifier, show it’s accuracy, and at last to avoid wasting the skilled mannequin for futuristic duties. As any hyperparameters will not be specified within the question, it considers default ones.
Random Forest: Random forest algorithm operates by setting up a number of determination timber throughout coaching and outputs the mode of the lessons or imply prediction of the person timber for regression duties.
question= r"""Practice a random forest classifier, print the accuracy, and save in .pkl"""
output_code= consumer.execute_query( question )
print()
print(output_code, sep="n")
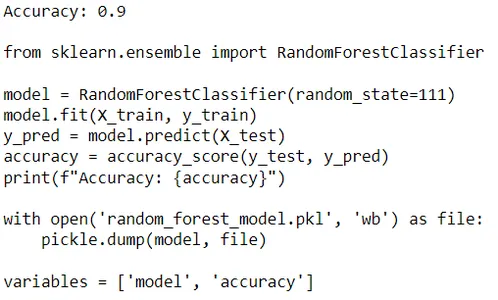
After efficiently coaching the mannequin, we carry out querying to examine the output based mostly on supplied enter options.
question= r"""Load the mannequin, and predict ouput for SepalLength= 5.1, SepalWidth= 3.5, PetalLength= 1.4, and PetalWidth= 0.2"""
output_code= consumer.execute_query( question )
print()
print(output_code, sep="n")
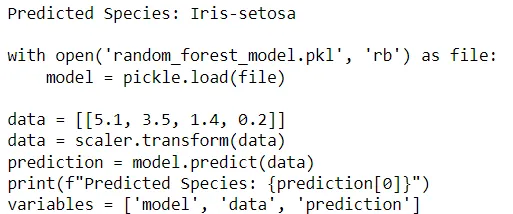
Future Implications
- Democratization of Programming: “Query2Model” might democratize programming by decreasing the barrier to entry for newbies, enabling people with restricted coding expertise to harness the ability of machine studying and automation.
- Elevated Productiveness: By automating the code technology course of, “Query2Model” has the potential to considerably improve productiveness, permitting builders to focus extra on problem-solving and innovation moderately than routine coding duties.
- Development of Pure Language Processing: The widespread adoption of such instruments could drive additional developments in pure language processing methods, fostering a deeper integration between human language and machine understanding in numerous domains past programming which result in the futuristic growth of Giant Motion Fashions(LAMs).
Conclusion
“Query2Model” represents an progressive answer for automating the method of producing and executing code based mostly on consumer queries. By leveraging pure language enter, the pipeline streamlines the interplay between customers and the system, permitting for seamless communication of necessities. By integration with the HuggingChat API, the system effectively processes queries and generates related code, offering customers with well timed and correct responses. With its means to execute Python code, “Query2Model” empowers customers to acquire desired outputs effortlessly, enhancing productiveness and comfort within the realm of code technology and execution. It’s extremely helpful to newbies in addition to working professionals.
Key Takeaways
- HuggingChat, an AI-powered chatbot, revolutionizes consumer interplay by simplifying complicated duties into pure language queries, enhancing accessibility and effectivity.
- Query2Model facilitates seamless mannequin coaching pipelines, enabling customers to navigate machine studying workflows effortlessly by way of intuitive pure language queries.
- Builders can customise chatbots like HuggingChat for code technology duties, probably lowering growth time and enhancing productiveness.
- Immediate engineering methods leverage the outputs of huge language fashions (LLMs), reminiscent of GPT, to generate fascinating code snippets effectively and precisely.
Ceaselessly Requested Questions
A. HuggingChat streamlines machine studying duties by permitting customers to work together with the system by way of pure language queries, eliminating the necessity for complicated programming syntax and instructions.
A. Sure, customers can tailor HuggingChat’s performance to swimsuit numerous code technology duties, making it adaptable and versatile for various programming wants.
A. Query2Model empowers customers by offering a user-friendly interface for constructing and coaching machine studying fashions, making complicated duties accessible to people with various ranges of experience.
A. AI-powered chatbots have the potential to democratize programming by decreasing the barrier to entr. It enhance developer productiveness by automating repetitive duties, and drive developments in pure language processing methods.
The media proven on this article will not be owned by Analytics Vidhya and is used on the Writer’s discretion.