Introduction
This information focuses on mastering Python and NumPy, a robust library for numerical computing. It affords 30 ideas and tips to boost coding abilities, overlaying foundational matrix operations and superior statistical evaluation strategies. Sensible examples accompany every tip, permitting customers to navigate advanced knowledge manipulations and scientific computations. The information goals to unlock the complete potential of Python and NumPy and uncover Numpy ideas and tips for Python.
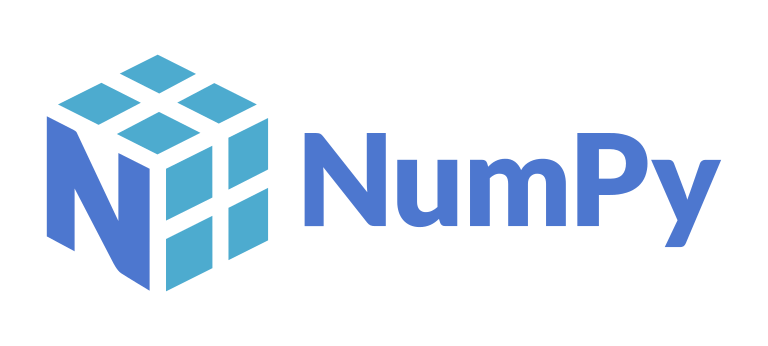
You too can enroll in our free python course right now!
Numpy Suggestions and Tips for Python
Listed below are 30 helpful NumPy tips overlaying numerous functionalities:
1. Create a Matrix of Zeros
A helpful utility within the NumPy library for Python numerical computing is the numpy.zeros operate. It produces an array that has simply zeros in it. This operate is useful when you must initialize an array with zeros of a sure knowledge kind and kind.
Generate a matrix of zeros with a specified form:
import numpy as np
zeros_matrix = np.zeros((3, 3))
This line creates a 3×3 matrix full of zeros utilizing the np.zeros() operate.
- np.zeros() is a NumPy operate that generates an array full of zeros.
(3, 3)
contained in the operate name specifies the form of the array as a tuple (rows, columns). So, on this case, it creates a 3×3 matrix.
2. Create a Matrix of Ones
Builders often create a ones matrix utilizing NumPy’s np.ones() technique, which generates an array containing components that each one have the worth 1. This matrix finds frequent use in knowledge processing and mathematical computations because it helps initialize matrices with a preset form.
Generate a matrix of ones with a specified form:
ones_matrix = np.ones((3, 3))
This line of code initializes a 3×3 matrix full of components having a worth of 1 utilizing NumPy’s np.ones() operate. The ensuing matrix is assigned to the variable ones_matrix, which can be utilized for additional computations or processing.
3. Id Matrix
We all know a sq. matrix as an id matrix, or 𝐼𝑛In, the place each diagonal member is 1 and each non-diagonal aspect is 0.
Generate an id matrix:
identity_matrix = np.eye(3)
This line of code creates a 3×3 id matrix utilizing NumPy’s np.eye() operate. We assign the ensuing matrix to the variable identity_matrix.
4. Generate a Vary of Numbers
A NumPy operate referred to as np.arange() creates an array with entries uniformly spaced inside a specified interval.
Create an array of numbers inside a sure vary which might be uniformly spaced:
numbers = np.arange(0, 10, 2)
This code creates a NumPy array referred to as numbers with values starting from 0 to eight (inclusive) for every of its two iterations.
5. Random Array Era
The np.random.rand() operate generates random numbers from a uniform distribution over the interval [0, 1). One random float is produced when the function is invoked without any parameters.
Generate an array of random numbers:
random_array = np.random.rand(3, 3)
Using np.random.rand(), this line creates a 3×3 NumPy array full of random values drawn from a uniform distribution between 0 and 1. The variable random_array is given the resultant array.
6. Reshape an Array
NumPy’s reshape() method lets you modify an array’s shape without altering its contents. As long as the total number of elements is constant, it allows you to transform an array between different shapes.
Reshape an array to a different shape:
reshaped_array = np.arange(9).reshape(3, 3)
This line of code creates a 3×3 NumPy array named reshaped_array
.
Using np.arange(9), it first creates a 1D array with values ranging from 0 to 8. We then use the reshape() function to transform this 1D array into a 3×3 2D array.
7. Transpose a Matrix
To transpose an array or matrix in NumPy, use the transpose() method. A view of the array with the axes reversed is returned.
Transpose a matrix:
transposed_matrix = np.transpose(matrix)
This line uses NumPy’s np.transpose() function to transpose a matrix stored in the variable matrix. The transposed matrix is then assigned to the variable transposed_matrix.
The NumPy method np.diag() takes a square matrix as input and returns its diagonal members as an array. Alternatively, it takes a 1D array as input and creates a diagonal matrix using those elements.
Get diagonal elements of a matrix:
diagonal_elements = np.diag(matrix)
This line of code extracts the diagonal elements from a matrix using NumPy’s np.diag() function and assigns them to the variable diagonal_elements.
9. Concatenate Arrays
NumPy’s np.concatenate() function takes arrays and joins them along a given axis. After receiving two or more arrays as input, it concatenates them using the given axis and outputs the finished array.
Concatenate arrays horizontally or vertically:
concatenated_array = np.concatenate((array1, array2), axis=0)
This line gives the result to concatenated_array after concatenating two arrays, array1 and array2, along the rows (axis=0).
10. Stack Arrays
NumPy’s np.stack() function moves arrays along a new axis for stacking. It creates a new axis by stacking an array sequence along the designated axis.
Stack arrays along different axes:
stacked_array = np.stack((array1, array2), axis=1)
This line allocates the result to stacked_array and stacks arrays 1 and 2 horizontally (along columns).
11. Indexing and Slicing
Access elements using indexing and slicing:
element = array[0, 0]
sub_array = array[:, 1:3]
These strains take one aspect out of the array at (0, 0) and assign it to aspect. Moreover, they extract and assign to sub_array a sub-array that accommodates each row and column from index 1 via index 3, excluding index 3.
12. Broadcasting
Carry out operations between arrays of various shapes:
end result = array1 + 5
This code creates a new array referred to as end result by including 5 to every entry in array 1.
13. Vectorization
Every aspect in an array has its sine calculated by NumPy’s np.sin() operate. Every aspect within the new array is the sine of its corresponding aspect within the enter array, and it has the identical form as the unique array.
This is called vectorization as a result of it permits mathematical operations to be utilized to complete arrays (or vectors) without delay, fairly than iterating over particular person components.
Make the most of vectorized operations for quicker computation:
end result = np.sin(array)
Utilizing NumPy’s np.sin() technique, every aspect within the array is sine-calculated on this line, and the result’s assigned to the variable end result.
14. Discover Distinctive Components
NumPy’s np.distinctive() technique takes an array, eliminates duplicates, and returns the distinctive components sorted so as.
Discover distinctive components in an array:
unique_elements = np.distinctive(array)
This code searches the array array for distinctive components, assigning them to the unique_elements variable. After eliminating redundant components, it yields a sorted array with simply the distinctive values.
15. Filtering
Filter components primarily based on situations:
filtered_array = array[array > 0]
This piece of code creates a brand new array referred to as filtered_array by filtering the objects of the array array by selecting solely these which might be better than 0. To retrieve components that meet the factors array > 0, it employs boolean indexing.
16. Factor-wise Features
Every aspect in an array is squared by NumPy’s np.sq.() technique, which returns a brand new array containing the squared values.
Apply features element-wise:
squared_array = np.sq.(array)
By calculating the sq. of every aspect within the array array, this line of code creates a brand new array referred to as squared_array that holds the squared values.
17. Matrix Operations
NumPy’s np.dot() technique calculates the dot product of two arrays, which can be matrices or vectors. It computes the scalar product for vectors and matrix multiplication for matrices.
Carry out matrix operations like dot product:
dot_product = np.dot(matrix1, matrix2)
This line creates a brand new array referred to as dot_product by computing the dot product of matrix 1 and matrix 2.
18. Statistics
When calculating the arithmetic imply (common) of an array’s components, NumPy’s np.imply() technique returns a single end result that represents the common of the array’s components.
Calculate statistics like imply, median, and so on.:
mean_value = np.imply(array)
This line determines the imply (common) worth of every member within the array ‘array’, after which shops the end result within the variable ‘mean_value’.
19. Save and Load
A NumPy operate referred to as np.save() is used to retailer arrays in a binary format to disk. The array to be saved and the filename (together with the.npy suffix) are the 2 inputs it requires. This operate successfully saves the array knowledge to disk whereas sustaining the info sorts and type of the array.
A NumPy operate referred to as np.load() is used to load arrays from recordsdata which have beforehand been saved utilizing np.save(). It returns the array contained in that file after receiving the filename as an enter. You may work together with the info as if it had been loaded straight from reminiscence by utilizing this operate to recreate the array in reminiscence.
Collectively, these features present a handy technique to save and cargo NumPy arrays, facilitating knowledge persistence and reuse.
Save and cargo arrays to/from recordsdata:
np.save('array.npy', array)
loaded_array = np.load('array.npy')
This code makes use of NumPy’s np.save() operate to save lots of the array array to a file referred to as “array.npy.” It then masses the saved array again into reminiscence and assigns it to the variable loaded_array utilizing np.load().
20. Interpolation
Utilizing recognized knowledge factors, the NumPy np.interp() operate makes use of linear interpolation to estimate values inside a variety. It returns an array of interpolated values relying on the question factors and knowledge factors equipped.
Interpolate values in an array:
interpolated_values = np.interp(x_values, xp, fp)
Utilizing the xp and fp arrays, this line of code interpolates the x_values linearly. Primarily based on the linear interpolation between the values in xp and fp, it returns an array of interpolated values matching to x_values.
21. Linear Algebra
The NumPy operate np.linalg.eig() calculates a sq. matrix’s eigenvalues and eigenvectors. The enter matrix’s eigenvalues and matching eigenvectors are the 2 arrays which might be returned.
Carry out linear algebraic operations:
eigenvalues, eigenvectors = np.linalg.eig(matrix)
This piece of code makes use of NumPy’s np.linalg.eig() operate to calculate the sq. matrix matrix’s eigenvalues and eigenvectors. It provides again two arrays: eigenvalues, which include the matrix’s eigenvalues, and eigenvectors, which include the matching eigenvectors.
22. Cumulative Sum
The NumPy np.cumsum() operate calculates the cumulative sum of the weather in an array alongside a given axis. Every entry within the returned array represents the cumulative whole of all of the entries as much as that index alongside the given axis.
Calculate cumulative sum alongside a specified axis:
cumulative_sum = np.cumsum(array, axis=0)
This line creates a brand new array referred to as cumulative_sum by calculating the cumulative sum alongside the rows (axis=0) of the array array.
23. Kind Array Components
The NumPy np.type() operate arranges an array’s objects alongside a given axis in ascending order. The previous array is left intact and is changed with a brand new array that has the sorted components in it.
Kind components of an array:
sorted_array = np.type(array)
This line assigns the end result to the variable sorted_array and types the array array’s members in ascending order.
24. Factor-wise Comparability
In NumPy, when evaluating two arrays for equality, the np.array_equal() technique returns True if their shapes and components match, and False in any other case.
Carry out element-wise comparability between arrays:
comparison_result = np.array_equal(array1, array2)
This line checks if two arrays, array1 and array2, are equal. If they’re, it returns True; if not, it returns False. The variable comparison_result is allotted the end result.
25. Repeating Components
NumPy’s np.tile() operate multiplies an array’s dimension by copying its contents alongside given dimensions. It requires a tuple indicating the variety of repeats alongside every dimension along with the array to be tiled.
Repeat components of an array:
repeated_array = np.tile(array, (2, 2))
By tiling or repeating the contents of array twice alongside each dimensions, this line creates a brand new array referred to as repeated_array, which is bigger than the unique array.
26. Set Operations
While you union two arrays utilizing NumPy’s np.union1d() operate, it returns a brand new array with distinctive components from each enter arrays.
Carry out set operations like union, intersection, and so on.:
union = np.union1d(array1, array2)
This line provides the end result to the variable union after calculating the union of the weather in arrays 1 and a pair of. Distinctive components from both array1 or array2 are current within the ensuing array.
27. Histogram
The NumPy operate np.histogram() calculates an array’s histogram, which exhibits the frequency distribution of values inside designated bins. The road returns two arrays: the bin edges and the histogram values, or frequencies.
Compute histogram of array values:
hist, bins = np.histogram(array, bins=10)
On this line, we compute the histogram of the array ‘array’ with 10 bins, leading to two arrays: ‘bins’, which accommodates the bin edges, and ‘hist’, which accommodates the frequencies of values in every bin.
28. Discover Max and Min
NumPy routines referred to as np.max() and np.min() are used to discover the largest and least values in an array, respectively. With out taking into account any NaN values, they return the array’s highest and lowest values.
Discover most and minimal values in an array:
max_value = np.max(array)
min_value = np.min(array)
These strains decide the array array’s most and minimal values, respectively, then assign them to the variables max_value and min_value.
29. Compute Dot Product
NumPy’s np.dot() operate calculates the dot product of two arrays. It computes the dot product, also called the scalar product, of vectors in 1-D arrays. It carries out matrix multiplication for 2-D arrays.
Compute dot product of vectors:
dot_product = np.dot(vector1, vector2)
The dot product of vectors 1 and a pair of is calculated on this line, and the result’s a scalar worth that’s assigned to the variable dot_product.
30. Broadcast to Appropriate Shapes
The NumPy operate np.broadcast_to() broadcasts an array’s contents to a given form and creates a new array with that kind. To fill the new form, it repeats the components in the enter array as wanted.
Mechanically broadcast arrays to suitable shapes:
broadcasted_array = np.broadcast_to(array, (3, 3))
By broadcasting the contents of array to a bigger kind (3, 3), this line produces a brand new array referred to as broadcasted_array. This means that the bigger kind might be crammed by repeating the objects of the array.
Conclusion
This information supplies 30 Numpy ideas and tips for Python, enhancing coding abilities in numerical computing. It covers creating matrices, performing array manipulations, and conducting superior statistical evaluation. This information equips customers to deal with numerous challenges in knowledge science, machine studying, and scientific analysis. The important thing to success lies in steady studying and exploration. Embrace the facility of Python and NumPy, and let your coding journey flourish.