Introduction
Unlocking your coding potential requires investigating the syntax and have set of Python. Chances are you’ll optimize your code for efficiency and class by studying primary ideas like listing comprehensions, producing expressions, and unpacking. Discover an enormous array of fastidiously chosen 30+ Python ideas and tips which might be certain to enhance your programming expertise and allow you to write down clear, environment friendly, and Pythonic code that appeals to each novice and skilled builders.
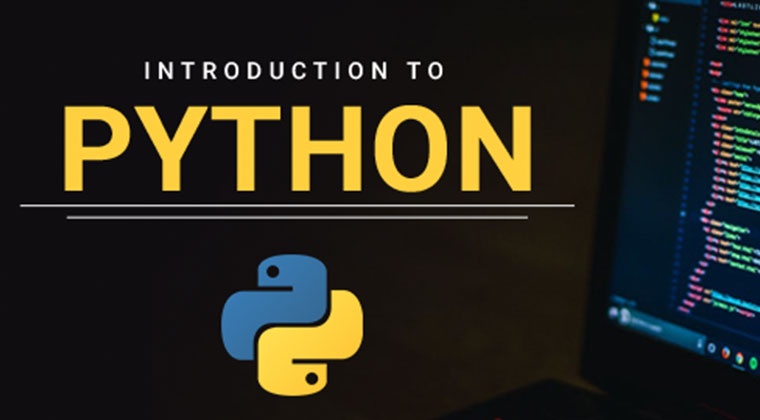
You can too enroll in our free python course as we speak!
30+ Python Ideas and Tips
1. Listing Comprehensions
Listing comprehensions in Python present a streamlined strategy to creating lists from pre-existing iterables. A number of for or if clauses, an expression, after which one other expression make up their composition. They provide an alternative choice to conventional looping algorithms for list-building that’s each extra concise and understandable.
Use listing comprehensions for concise creation of lists.
squares = [x**2 for x in range(10)]
print(squares)
# Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
2. Dictionary Comprehensions
Python dictionary comprehensions allow you to construct dictionaries in a transparent and expressive manner. They generate dictionaries as a substitute of lists, though they use a syntax just like that of listing comprehensions. Inside curly braces {}, you specify key-value pairs the place a colon (:) separates the important thing and worth.
squares_dict = {x: x**2 for x in vary(10)}
print(squares_dict)
# Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
3. Generator Expressions
Generator expressions of python work equally to listing comprehensions. However they yield a generator object versus an inventory. They supply a memory-efficient technique of making information immediately, which may be very useful for large datasets. Generator expressions exchange sq. brackets [] with parentheses () in Python.
squares_gen = (x**2 for x in vary(10))
for num in squares_gen:
print(num)
# Output: 0 1 4 9 16 25 36 49 64 81
4. Unpacking
Unpacking in python lets you take particular person components out of iterables, such lists or tuples, and put them into variables. When you’ve got information units and want to entry their particular person elements independently, you steadily use it.
a, b, c = [1, 2, 3]
print(a, b, c)
# Output: 1 2 3
5. A number of Assignments in One Line
In Python, you can provide values to quite a few variables in a single assertion by utilizing a number of assignments in a single line. Whenever you want to assign quite a few values without delay, utilizing totally different or the identical information sorts, that is fairly useful.
a, b = 1, 2
print(a, b)
# Output: 1 2
6. Enumerate
An enumerate object, which contains pairs of indices and values from an iterable. The enumerate() operate in Python returns it. It’s particularly useful when you must iterate over an iterable whereas sustaining observe of every factor’s index or place.
for index, worth in enumerate(squares):
print(index, worth)
# Output: (0, 0) (1, 1) (2, 4) (3, 9) (4, 16) (5, 25) (6, 36) (7, 49) (8, 64) (9, 81)
7. Zip
Zip operate of python is a built-in operate. It takes as enter two or extra iterables and outputs an iterator that builds tuples within the output tuple with the i-th factor from every of the enter iterables.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
for a, b in zip(list1, list2):
print(a, b)
# Output: 1 a 2 b 3 c
8. zip(*iterables) Unpacking Trick
Python’s zip(*iterables) unpacking operate permits the conversion of knowledge from rows to columns or vice versa. It takes many iterables as enter and outputs an iterator that takes every enter iterable that makes up the i-th tuple and makes use of it to create tuples with the i-th factor.
matrix = [[1, 2], [3, 4], [5, 6]]
transposed = zip(*matrix)
print(listing(transposed))
# Output: [(1, 3, 5), (2, 4, 6)]
9. collections.Counter
The built-in Python class collections.Counter is utilized to rely the occurrences of components in a group, normally in iterables comparable to lists or strings. It gives a handy solution to carry out frequency counting in a Pythonic and environment friendly method.
from collections import Counter
counts = Counter([1, 2, 1, 3, 1, 2, 4])
print(counts)
# Output: Counter({1: 3, 2: 2, 3: 1, 4: 1})
10. collections.defaultdict
collections.defaultdict is a Python subclass that gives a default worth for every key in a dictionary, eliminating the necessity to verify if a key exists, particularly helpful for nested dictionaries or lacking keys.
from collections import defaultdict
d = defaultdict(listing)
d['key'].append('worth')
print(d['key'])
# Output: ['value']
11. collections.namedtuple
collections.namedtuple is a manufacturing unit operate for creating tuple subclasses with named fields. Named tuples are a handy technique for outlining easy lessons with out explicitly writing a full definition. Named tuples are immutable, however you possibly can entry their fields utilizing dot notation, making them extra readable and self-documenting.
from collections import namedtuple
Level = namedtuple('Level', ['x', 'y'])
p = Level(1, y=2)
print(p)
# Output: Level(x=1, y=2)
12. any() and all()
any()
: This operate returns True if a minimum of one factor within the iterable evaluates to True. If the iterable is empty, it returns False. It’s helpful whenever you wish to verify if any of the weather within the iterable fulfill a situation.all()
: This operate returns True if all components within the iterable consider to True. If the iterable is empty, it returns True. It’s helpful whenever you wish to verify if all components within the iterable fulfill a situation.
print(any([True, False, True]))
# Output: True
print(all([True, False, True]))
# Output: False
13. sorted()
The built-in Python operate sorted() is used to return a brand new sorted listing after sorting iterables comparable to lists, tuples, and strings. We are able to additionally move non-compulsory arguments to customise the sorting habits.
sorted_list = sorted([3, 1, 4, 1, 5, 9, 2])
print(sorted_list)
# Output: [1, 1, 2, 3, 4, 5, 9]
14. filter()
A built-in Python operate known as filter() can be utilized to use a given operate to filter components from an iterable. When the operate returns True, it returns an iterator that yields the weather of the iterable.
filtered_list = listing(filter(lambda x: x > 5, [3, 8, 2, 7, 1]))
print(filtered_list)
# Output: [8, 7]
15. map()
You’ll be able to get hold of an iterator yielding the outcomes of every merchandise in an iterable by utilizing the built-in Python operate map().
doubled_list = listing(map(lambda x: x * 2, [1, 2, 3, 4]))
print(doubled_list)
# Output: [2, 4, 6, 8]
16. functools.scale back()
You should use the built-in Python operate functools.scale back() to cut back an iterable to a single worth by making use of a operate with two arguments cumulatively on its members from left to proper.
from functools import scale back
product = scale back((lambda x, y: x * y), [1, 2, 3, 4])
print(product)
# Output: 24
17. *args and **kwargs
*args
: It’s used to move a variable variety of positional arguments to a operate, permitting it to just accept any variety of arguments and acquire them right into a tuple when used as a parameter in a operate definition.**kwargs
: It’s a notation used to move a variable variety of key phrase arguments to a operate, permitting it to just accept any variety of arguments and acquire them right into a dictionary when used as a parameter in a operate definition.
def my_func(*args, **kwargs):
print(args, kwargs)
my_func(1, 2, a=3, b=4)
# Output: (1, 2) {'a': 3, 'b': 4}
18. .__doc__
The docstring (documentation string) of a category, operate, module, or technique will be accessed utilizing the distinctive attribute.doc. It affords a method of describing the performance and documentation of Python programming.
def my_func():
"""It is a docstring."""
move
print(my_func.__doc__)
# Output: It is a docstring.
19. dir()
A sturdy built-in Python operate known as dir() retrieves the names of the attributes of an object or an inventory of names within the present native scope. It’s steadily employed for debugging, investigation, and introspection.
print(dir([]))
# Output: ['__add__', '__class__', '__contains__', ...]
20. getattr()
A built-in Python operate known as getattr() returns the worth of an object’s designated attribute. As well as, it affords a default worth within the occasion that the attribute is absent.
class MyClass:
attribute = 42
print(getattr(MyClass, 'attribute'))
# Output: 42
21. setattr()
To set an object’s named attribute worth, use the built-in Python operate setattr().
It lets you dynamically set the worth of an attribute on an object, even when the attribute hasn’t been declared but.
class MyClass:
move
obj = MyClass()
setattr(obj, 'attribute', 42)
print(obj.attribute)
# Output: 42
22. hasattr()
The hasattr() built-in Python operate can be utilized to find out whether or not an object has a selected attribute or not. It returns True if the item has the attribute and False in any other case.
class MyClass:
attribute = 42
print(hasattr(MyClass, 'attribute'))
# Output: True
23. __str__
and __repr__
In Python, builders use two particular strategies known as str and repr to outline string representations of objects. These strategies are instantly known as whenever you use features like print() or str(), or when an object is inspected within the interpreter.
- __str__(self): The str() operate calls this technique to return a string illustration of the item for end-users. It ought to return a human-readable illustration of the item.
- __repr__(self): The repr() operate returns an unambiguous string illustration of an object, usually used for debugging and logging. The returned string needs to be legitimate Python code that may recreate the item if handed to eval().
class MyClass:
def __str__(self):
return 'MyClass'
def __repr__(self):
return 'MyClass()'
obj = MyClass()
print(str(obj), repr(obj))
# Output: MyClass MyClass()
24. *
and **
Operators
In Python, the * (asterisk) and ** (double asterisk) operators are used for unpacking iterables and dictionaries, respectively, in operate calls, listing literals, tuple literals, and dictionary literals. They’re highly effective instruments for working with variable-length argument lists and dictionary unpacking.
def my_func(a, b, c):
print(a, b, c)
my_list = [1, 2, 3]
my_dict = {'a': 1, 'b': 2, 'c': 3}
my_func(*my_list)
my_func(**my_dict)
# Output: 1 2 3
# 1 2 3
25. move Assertion
The move assertion in Python is a null operation that does nothing when executed, serving as a placeholder for syntactically required statements with none motion wanted, usually used for unimplemented code or empty code blocks.
def my_func():
move
my_func()
26. with Assertion
The with assertion in Python streamlines exception dealing with and useful resource administration by making certain correct initialization and cleanup, making it helpful for managing information, community connections, and different sources to forestall errors.
with open('file.txt', 'r') as f:
information = f.learn()
27. __init__.py
The init.py file is a particular Python file that’s used to outline a bundle. It may be empty or comprise initialization code for the bundle.
# In a listing known as 'my_package'
# __init__.py will be empty or comprise initialization code
28. @staticmethod
In Python, builders outline static strategies inside a category utilizing the @staticmethod decorator.
These strategies, known as static strategies, belong to the category and will be straight known as on the category itself, with out requiring entry to occasion variables or strategies.
class MyClass:
@staticmethod
def my_method():
print('Static technique known as')
MyClass.my_method()
# Output: Static technique known as
29. @classmethod
In Python, builders outline class strategies inside a category utilizing the @classmethod decorator. These strategies, often known as class strategies, are related to the category itself fairly than particular person class situations. They are often known as on each the category and its situations, and so they have entry to the category itself by the cls parameter.
class MyClass:
@classmethod
def my_method(cls):
print('Class technique known as')
MyClass.my_method()
# Output: Class technique known as
30. is and isn’t
Python comparability operators is and is not are used to confirm the id of an object. Slightly of figuring out if two objects have the similar worth, they decide if they correspond to the similar reminiscence handle.
- is: If two variables belong to the similar reminiscence merchandise, this operator returns True; in any other case, it returns False.
- is not: If two variables do not correspond to the similar object in reminiscence, this operator returns True; if they do, it returns False.
x = None
print(x is None)
# Output: True
31. del Assertion
In Python, builders use the del assertion to delete objects. It may take away components from lists, slices from lists or arrays, delete whole variables, and carry out different actions.
my_list = [1, 2, 3]
del my_list[1]
print(my_list)
# Output: [1, 3]
32. lambda Features
Lambda features in Python are small, nameless features outlined utilizing the lambda
key phrase. They’re usually used for brief, one-time operations the place defining a named operate is pointless. Lambda features can take any variety of arguments, however can solely have a single expression.
sq. = lambda x: x**2
print(sq.(5))
# Output: 25
33. attempt, besides, else, lastly
In Python, the attempt
, besides
, else
, and lastly
blocks are used for dealing with exceptions and executing cleanup code in case of exceptions or no matter whether or not exceptions happen.
Right here’s an evidence of every block in energetic voice:
attempt
block: In Python, builders use the attempt block to catch exceptions throughout execution, enclosing the code which may elevate them and making an attempt to execute it.besides
block: In Python, builders make the most of the besides block to deal with exceptions inside the attempt block, executing the code contained in the besides block if it matches the exception sort.else
block: Theelse
block is executed if the code contained in theattempt
block executes efficiently with out elevating any exceptions. Builders usually use this block to execute code provided that no exceptions happen.lastly
block: The lastly block is an important block in programming that executes cleanup operations, comparable to closing information or releasing sources, no matter exceptions.
attempt:
x = 1 / 0
besides ZeroDivisionError:
print('Error: division by zero')
else:
print('No exceptions raised')
lastly:
print('This at all times runs')
# Output: Error: division by zero
# This at all times runs
Conclusion
The huge array of strategies and capabilities that the Python programming language affords is excess of simply the helpful hints and options. Chances are you’ll enhance as a Python developer and study extra concerning the language’s potentialities by making use of these tips to your code. Each novice and seasoned builders can profit from these pointers, which is able to assist you to advance your Python data and programming expertise. These 30+ python ideas and tips will information you and act as a catalyst in your coding journey.