Introduction
Unlock the potential of Python’s knowledge manipulation capabilities with the environment friendly and concise strategy of dictionary comprehension. This text delves into the syntax, functions, and examples of dictionary comprehension in Python. From superior methods to potential pitfalls, discover how dictionary comprehension stacks up towards different knowledge manipulation strategies.
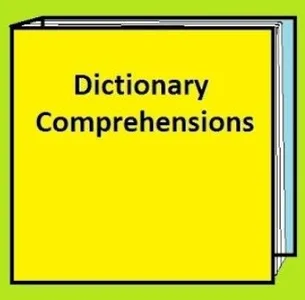
Simplifying with Dictionary Comprehension
Streamlining the dictionary creation course of, dictionary comprehension harmonizes components, expressions, and non-obligatory situations. The end result ? A contemporary dictionary emerges with out the burden of specific loops and conditional statements.
Learn extra about Python Dictionaries- A Tremendous Information for a dictionaries in Python for Absolute Learners
Syntax and Utilization of Dictionary Comprehension
Grasp the syntax:
{key_expression: value_expression for factor in iterable if situation}
The key_expression signifies the important thing for every factor within the iterable, and the value_expression signifies the corresponding worth. The variable ‘factor’ adopts the worth of every merchandise within the iterable, and the situation, if specified, filters which components are included within the dictionary.
Examples of Dictionary Comprehension in Python
Making a Dictionary from Lists
Suppose we’ve two lists, one containing names and the opposite containing ages. We will use dictionary comprehension to create a dictionary the place the names are the keys and the ages are the values.
names = ['Himanshu', 'Tarun', 'Aayush']
ages = [25, 30, 35]
person_dict = {identify: age for identify, age in zip(names, ages)}
print(person_dict)
Output:
{‘Himanshu’: 25, ‘Tarun’: 30, ‘Aayush’: 35}
Filtering and Remodeling Dictionary Components
We will make use of dictionary comprehension to filter and rework components primarily based on particular situations. As an example, contemplate a state of affairs the place we’ve a dictionary containing college students and their scores. We will use dictionary comprehension to generate a brand new dictionary that features solely the scholars who achieved scores above a specific threshold.
scores = {'Himanshu': 80, 'Tarun': 90, 'Nishant': 70, 'Aayush': 85}
passing_scores = {identify: rating for identify, rating in scores.gadgets() if rating >= 80}
print(passing_scores)
Output:
{‘Himanshu’: 80, ‘Tarun’: 90, ‘Aayush’: 85}
Nested Dictionary Comprehension
Nested dictionaries may be created utilizing dictionary comprehension, permitting for a number of ranges of nesting. As an example, suppose we’ve an inventory of cities and their populations. On this case, we will use nested dictionary comprehension to generate a dictionary with cities as keys and corresponding values as dictionaries containing data comparable to inhabitants and nation.
cities = ['New York', 'London', 'Paris']
populations = [8623000, 8908081, 2140526]
international locations = ['USA', 'UK', 'France']
city_dict = {metropolis: {'inhabitants': inhabitants, 'nation': nation}
for metropolis, inhabitants, nation in zip(cities, populations, international locations)}
print(city_dict)
Output:
{
‘New York’: {‘inhabitants’: 8623000, ‘nation’: ‘USA’},
‘London’: {‘inhabitants’: 8908081, ‘nation’: ‘UK’},
‘Paris’: {‘inhabitants’: 2140526, ‘nation’: ‘France’}
}
Conditional Dictionary Comprehension
Conditional statements may be integrated into dictionary comprehension to deal with particular instances. As an example, contemplate a state of affairs the place there’s a dictionary containing temperatures in Celsius. We will make the most of dictionary comprehension to provide a brand new dictionary by changing temperatures to Fahrenheit, however solely for temperatures which might be above freezing.
temperatures = {'New York': -2, 'London': 3, 'Paris': 1}
fahrenheit_temperatures = {metropolis: temp * 9/5 + 32 for metropolis,
temp in temperatures.gadgets() if temp > 0}
print(fahrenheit_temperatures)
Output:
{‘London’: 37.4, ‘Paris’: 33.8}
Dictionary Comprehension with Capabilities
Utilizing features in dictionary comprehension permits for the execution of advanced operations on components. For instance, suppose there’s a checklist of numbers. On this case, we will make use of dictionary comprehension to instantly create a dictionary the place the numbers function keys and their corresponding squares as values.
numbers = [1, 2, 3, 4, 5]
def sq.(num):
return num ** 2
squared_numbers = {num: sq.(num) for num in numbers}
print(squared_numbers)
Output:
{1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
Suggestions and Methods for Dictionary Comprehension
Dictionary comprehension is a robust instrument in Python for creating dictionaries with magnificence and conciseness. Whereas it simplifies the method, mastering some ideas and methods can additional elevate your knowledge manipulation abilities. Let’s delve into these superior strategies:
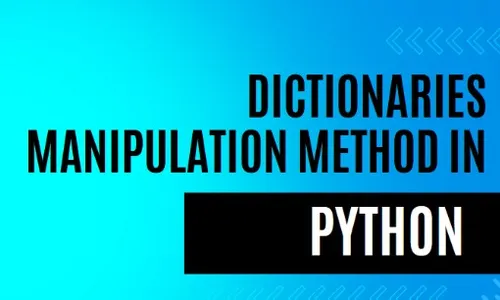
- Dealing with Duplicate Keys: Deal with duplicate keys by using set comprehension to remove duplicates earlier than establishing the dictionary. This ensures every key retains its distinctive worth.
- Combining with Set Comprehension: Mix dictionary comprehension with set comprehension for dictionaries with distinctive keys. Splendid for situations the place knowledge could comprise duplicate keys, this system enhances readability and avoids key conflicts.
- Combining A number of Dictionaries: Merge dictionaries or create new ones by combining a number of dictionaries by way of dictionary comprehension. This system gives flexibility when coping with numerous datasets.
- Optimizing Efficiency: Optimize the efficiency of dictionary comprehension, particularly with giant datasets, by utilizing generator expressions as a substitute of lists. This strategy enhances reminiscence effectivity and general execution velocity.
Comparability with Different Knowledge Manipulation Methods
Dictionary Comprehension vs. For Loops
Dictionary comprehension gives a extra concise and readable technique for creating dictionaries in comparison with conventional for loops. It removes the need for specific loop initialization, iteration, and handbook appending to the dictionary.
Utilizing For Loops:
knowledge = [('a', 1), ('b', 2), ('c', 3)]
end result = {}
for key, worth in knowledge:
end result[key] = worth
print(end result)
Utilizing Dictionary Comprehension:
# Dictionary Comprehension for a similar activity
knowledge = [('a', 1), ('b', 2), ('c', 3)]
end result = {key: worth for key, worth in knowledge}
print(end result)
Dictionary Comprehension vs. Map and Filter Capabilities
Dictionary comprehension may be seen as a mixture of map and filter features. It permits us to rework and filter components concurrently, leading to a brand new dictionary.
Utilizing Map and Filter:
# Utilizing map and filter to create a dictionary
knowledge = [('a', 1), ('b', 2), ('c', 3)]
end result = dict(map(lambda x: (x[0], x[1]*2), filter(lambda x: x[1] % 2 == 0, knowledge)))
print(end result)
Utilizing Dictionary Comprehension:
# Dictionary Comprehension for a similar activity
knowledge = [('a', 1), ('b', 2), ('c', 3)]
end result = {key: worth*2 for key, worth in knowledge if worth % 2 == 0}
print(end result)
Dictionary Comprehension vs. Generator Expressions
Generator expressions, like checklist comprehensions, produce iterators slightly than lists. When utilized in dictionary comprehension, they improve reminiscence effectivity, notably when managing substantial datasets.
Utilizing Generator Expressions:
# Generator Expression to create an iterator
knowledge = [('a', 1), ('b', 2), ('c', 3)]
result_generator = ((key, worth*2) for key, worth in knowledge if worth % 2 == 0)
# Convert the generator to a dictionary
end result = dict(result_generator)
print(end result)
Utilizing Dictionary Comprehension:
# Dictionary Comprehension with the identical situation
knowledge = [('a', 1), ('b', 2), ('c', 3)]
end result = {key: worth*2 for key, worth in knowledge if worth % 2 == 0}
print(end result)
Frequent Pitfalls and Errors to Keep away from
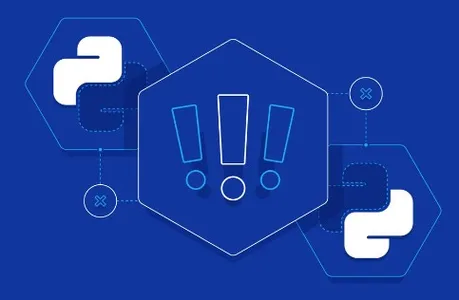
Overcomplicating Dictionary Comprehension
Keep away from unnecessarily complicating expressions or situations inside dictionary comprehension. Prioritize simplicity and readability to make sure clear code and ease of upkeep.
Incorrect Syntax and Variable Utilization
Utilizing incorrect syntax or variable names in dictionary comprehension is a typical mistake. It’s essential to confirm the syntax and guarantee consistency between the variable names within the comprehension and people within the iterable.
Not Dealing with Edge Circumstances and Error Dealing with
Dealing with edge instances and implementing error dealing with is important when using dictionary comprehension. This includes addressing situations comparable to an empty iterable or potential errors arising from the situations specified within the comprehension.
By steering clear of those widespread pitfalls, you fortify your dictionary comprehension abilities, guaranteeing that your code stays not simply concise but in addition resilient to varied knowledge situations. Embrace these tips to raise your proficiency in Python’s knowledge manipulation panorama.
Conclusion
Python dictionary comprehension empowers knowledge manipulation by simplifying the creation of dictionaries. Eradicate the necessity for specific loops and situations, and improve your knowledge manipulation abilities. Dive into this complete information and leverage the effectivity and magnificence of dictionary comprehension in your Python initiatives.
You can too study checklist comprehension from right here.
Incessantly Requested Questions
A. Python Dictionary Comprehension is a concise and environment friendly method to create dictionaries by combining an expression and an non-obligatory situation. It streamlines the method, eliminating the necessity for specific loops. The advantages embrace code magnificence, readability, and effectivity.
A. The syntax follows this sample: {key_expression: value_expression for factor in iterable if situation}. Key_expression represents the important thing, value_expression represents the related worth, ‘factor’ is a variable for every iterable merchandise, and ‘situation’ is an non-obligatory filter.
Actually! As an example, given two lists ‘names’ and ‘ages’, {identify: age for identify, age in zip(names, ages)} creates a dictionary the place names are keys and ages are values.
By incorporating an non-obligatory situation, Dictionary Comprehension can filter and rework components primarily based on particular standards. As an example, {identify: rating for identify, rating in scores.gadgets() if rating >= 80} filters college students who scored above 80.
Completely! Nested Dictionary Comprehension allows creating dictionaries with a number of ranges of nesting. It’s helpful for advanced constructions like {metropolis: {‘inhabitants’: inhabitants, ‘nation’: nation} for metropolis, inhabitants, nation in zip(cities, populations, international locations)}.