Introduction
In pure language processing (NLP), it is very important perceive and successfully course of sequential information. Lengthy Brief-Time period Reminiscence (LSTM) fashions have emerged as a strong device for tackling this problem. They provide the aptitude to seize each short-term nuances and long-term dependencies inside sequences. Earlier than delving into the intricacies of LSTM language translation fashions, it’s essential to understand the basic idea of LSTMs and their function inside Recurrent Neural Networks (RNNs). This text offers a complete information to understanding, implementing, and evaluating LSTM fashions for language translation duties, with a deal with translating English sentences into Hindi. Via a step-by-step method, we’ll discover the structure, preprocessing methods, mannequin constructing, coaching, and analysis of LSTM fashions.
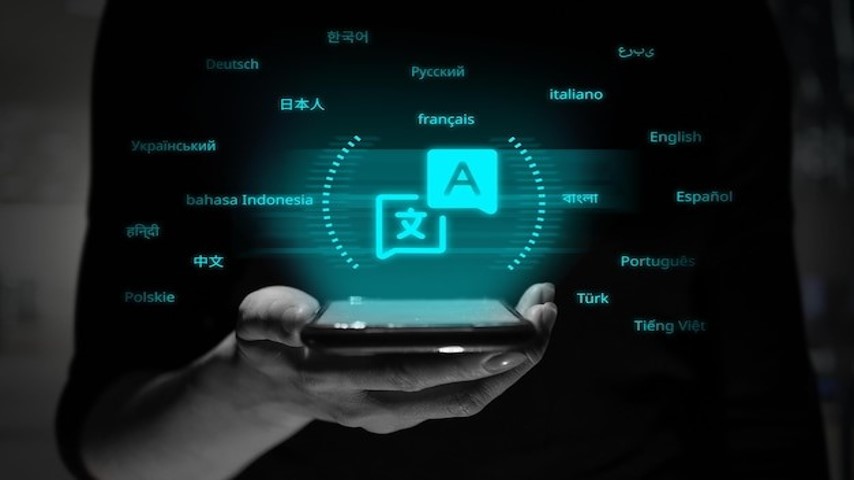
Studying Goal
- Perceive the basics of LSTM structure.
- Learn to preprocess sequential information for LSTM fashions.
- Implement LSTM fashions for sequence prediction duties.
- Consider and interpret LSTM mannequin efficiency.
What’s RNN?
Recurrent Neural Networks (RNNs) serve a vital goal within the space of neural networks as a result of their distinctive means to deal with sequential information successfully. Not like different sorts of neural networks, RNNs are particularly designed to seize dependencies inside sequential information factors.
Contemplate the instance of textual content information, the place every information level Xi represents a sequence of phrases or sentences. In pure language, the order of phrases issues considerably, in addition to the semantic relationships between them. Nevertheless, typical neural networks typically overlook this facet, treating the enter as an unordered set of options. Consequently, they wrestle to understand the inherent construction and which means inside the textual content.
RNNs handle this limitation by sustaining relationships between phrases throughout all the sequence. They obtain this by introducing a time axis, primarily making a looped construction the place every phrase within the enter sequence is processed sequentially, incorporating info from each the present phrase and the context supplied by earlier phrases.
This construction permits RNNs to seize short-term dependencies inside the information. Nevertheless, they nonetheless face challenges in preserving long-term dependencies successfully. Within the context of the time axis illustration, RNNs encounter issue in sustaining sturdy connections between the primary and final phrases of the sequence. That is primarily as a result of tendency for earlier inputs to have much less affect on later predictions, resulting in the potential lack of context and which means over longer sequences.
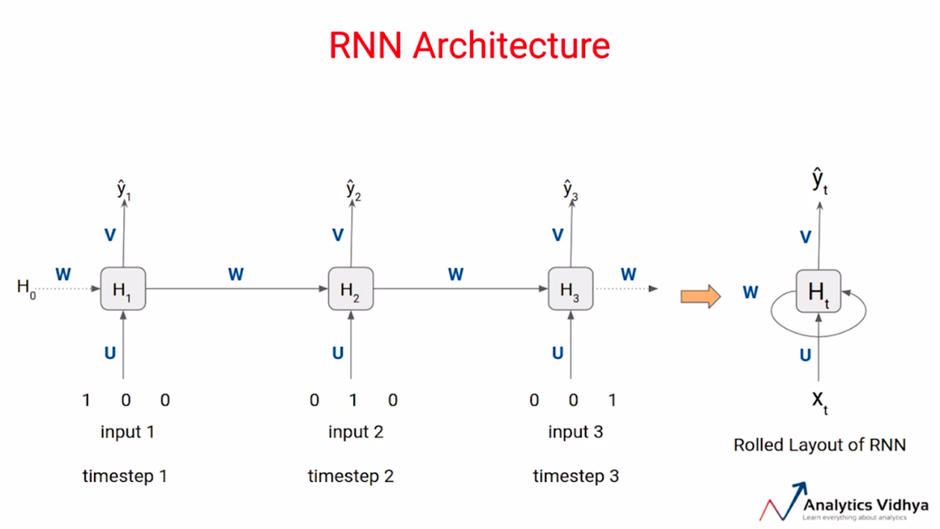
What’s LSTM?
Earlier than delving into LSTM language translation fashions, it’s important to understand the idea of LSTMs.
LSTM stands for Lengthy Brief-Time period Reminiscence, which is a specialised kind of RNN. Because the title suggests, LSTMs are designed to successfully seize each long-term and short-term dependencies inside sequential information. In the event you’re fascinated about studying extra about RNNs and LSTMs, you may discover the assets obtainable right here and right here. However let me offer you a concise overview of them.
LSTMs gained recognition for his or her means to deal with the restrictions of conventional RNNs, significantly in sustaining each long-term and short-term dependencies inside sequential information. This achievement is facilitated by the distinctive construction of LSTMs.
The LSTM construction could initially seem intricate, however I’ll simplify it for higher understanding. The time axis of a knowledge level, labeled as xt0 to xtn, corresponds to particular person blocks representing cell states, denoted as h_t, which output the corresponding cell state. The yellow sq. bins symbolize activation features, whereas the spherical pink bins signify pointwise operations. Let’s delve into the core idea.
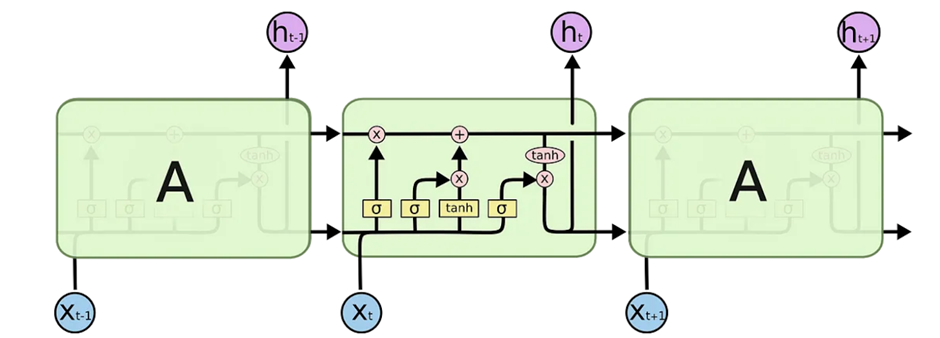
The elemental thought behind LSTMs is to handle long-term and short-term dependencies successfully. That is completed by selectively discarding unimportant parts x_t whereas retaining crucial ones by identification mapping. LSTMs will be distilled into three major gates, every serving a definite goal.
1. Neglect Gate
The Neglect Gate determines the relevance of data from the earlier state to be retained or discarded for the following state. It merges info from the earlier hidden state h_t-1 and the present enter x_t, passing it by a sigmoid perform to supply values between 0 and 1. Values nearer to 0 signify info to overlook, whereas these nearer to 1 point out info to maintain, achieved by applicable weight backpropagation throughout coaching.
2. Enter Gate
The Enter Gate manages information updates to the cell state. It merges and processes the earlier hidden state h_t-1 and the present enter x_t by a sigmoid perform, producing values between 0 and 1. These values, indicating significance, are pointwise multiplied with the output of the tanh perform, which squashes values between -1 and 1 to control the community. The ensuing product determines the related info to be added to the cell state.
3. Cell State
The Cell State combines the numerous info retained from the Neglect Gate (representing the necessary info from the earlier state) and the Enter Gate (representing the necessary info from the present state) by pointwise addition. This replace yields a brand new cell state c_t that the neural community deems related.
4. Output Gate
Lastly, the Output Gate determines the data related to the following hidden state. It merges the earlier hidden state and the present enter right into a sigmoid perform to find out which info to retain. Concurrently, the modified cell state is handed by a tanh perform. The outputs are then multiplied to resolve the data to hold ahead to the following hidden state.
It’s necessary to notice that the hidden state retains info from earlier enter states, making it helpful for predictions, and is handed because the output for the present state h_t.
Drawback Assertion
Our goal is to make the most of an LSTM sequence-to-sequence mannequin to translate English sentences into their corresponding Hindi counterparts.
For this, I’m taking a dataset from hugging face
Step 1: Loading the Knowledge from Hugging Face
!pip set up datasets
from datasets import load_datasetdf=load_dataset("Aarif1430/english-to-hindi")
df['train'][0]

import pandas as pd
da = pd.DataFrame(df['train']) # Assuming you wish to load the practice cut up
da.rename(columns={'english_sentence': 'english', 'hindi_sentence': 'hindi'}, inplace=True)
da.head()
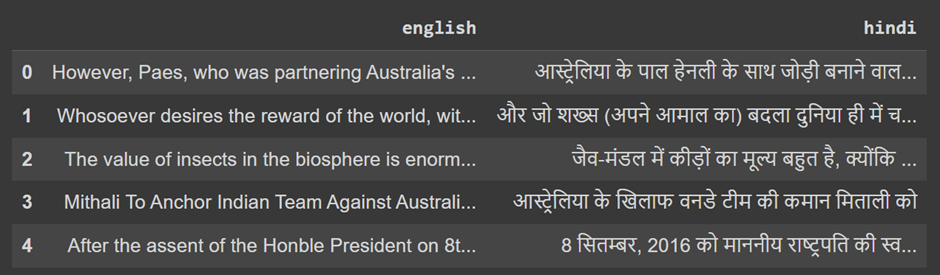
On this code, we set up the dataset library if not already put in. Then, use the load_dataset perform to load the English-Hindi dataset from Hugging Face. We convert the dataset into pandas DataFrame for additional processing and show the primary few rows to confirm the information loading.
Step 2: Importing Vital Libraries
import numpy as np
import string
from numpy import array, argmax, random, take
import pandas as pd
from keras.fashions import Sequential
from keras.layers import Dense, LSTM, Embedding, RepeatVector
from keras.preprocessing.textual content import Tokenizer
from keras.callbacks import ModelCheckpoint
from keras.preprocessing.sequence import pad_sequences
from keras.fashions import load_model
from keras import optimizers
from tensorflow.keras.fashions import Sequential
from tensorflow.keras.layers import Embedding, LSTM
import matplotlib.pyplot as plt
import tensorflow as tf
import warnings
warnings.filterwarnings("ignore")
Right here, we’ve got imported all the mandatory libraries and modules required for information preprocessing, mannequin constructing, and analysis.
Step 3: Knowledge Preprocessing
#Eradicating punctuations and changing textual content to lowercase for each languages
da['english'] = da['english'].str.change('[{}]'.format(string.punctuation), '').str.decrease()
da['hindi'] = da['hindi'].str.change('[{}]'.format(string.punctuation), '').str.decrease()# Discover indices of empty rows in each languages
eng_empty_indices = da[da['english'].str.strip().astype(bool) == False].index
hin_empty_indices = da[da['hindi'].str.strip().astype(bool) == False].index
# Mix indices from each languages to take away empty rows
remove_indices = checklist(set(eng_empty_indices) | set(hin_empty_indices))
# Eradicating empty rows
da.drop(remove_indices, inplace=True)
# Reset indices
da.reset_index(drop=True, inplace=True)
Right here , we preprocess the information by eradicating punctuation and changing textual content to lowercase for each English and Hindi sentences. Moreover, we deal with empty rows by discovering and eradicating them from the dataset.
Step 4: Tokenization and Sequence Padding
# Importing essential libraries
from tensorflow.keras.preprocessing.textual content import Tokenizer
from tensorflow.keras.preprocessing.sequence import pad_sequences# Initialize Tokenizer for English subtitles
tokenizer_eng = Tokenizer()
tokenizer_eng.fit_on_texts(da['english'])
# Convert textual content to sequences of integers for English subtitles
sequences_eng = tokenizer_eng.texts_to_sequences(da['english'])
# Initialize Tokenizer for Hindi subtitles
tokenizer_hin = Tokenizer()
tokenizer_hin.fit_on_texts(da['hindi'])
# Convert textual content to sequences of integers for Hindi subtitles
sequences_hin = tokenizer_hin.texts_to_sequences(da['hindi'])
# Pad sequences to make sure uniform size
max_length = 100 # Outline the utmost sequence size
sequences_eng = pad_sequences(sequences_eng, maxlen=max_length, padding='put up')
sequences_hin = pad_sequences(sequences_hin, maxlen=max_length, padding='put up')
# Confirm the vocabulary sizes
vocab_size_eng = len(tokenizer_eng.word_index) + 1
vocab_size_hin = len(tokenizer_hin.word_index) + 1
print("Vocabulary dimension for English subtitles:", vocab_size_eng)
print("Vocabulary dimension for Hindi subtitles:", vocab_size_hin)

Right here, we import the mandatory libraries for tokenization and sequence padding. Then, we tokenize the textual content information for each English and Hindi sentences and convert them into sequences of integers. We pad the sequences to make sure uniform size, and at last, we print the vocabulary sizes for each languages.
Figuring out Sequence Lengths
eng_length = sequences_eng.form[1] # Size of English sequences
hin_length = sequences_hin.form[1] # Size of Hindi sequences
print(eng_length, hin_length)
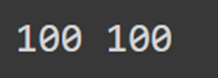
On this, we’re figuring out the lengths of the sequences for each English and Hindi sentences. The size of a sequence refers back to the variety of tokens or phrases within the sequence.
Step 5: Splitting Knowledge into Coaching and Validation Units
from sklearn.model_selection import train_test_split# Cut up the coaching information into coaching and validation units
X_train, X_val, y_train, y_val = train_test_split(sequences_eng[:50000], sequences_hin[:50000], test_size=0.2, random_state=42)
# Confirm the shapes of the datasets
print("Form of X_train:", X_train.form)
print("Form of y_train:", y_train.form)
print("Form of X_val:", X_val.form)
print("Form of y_val:", y_val.form)
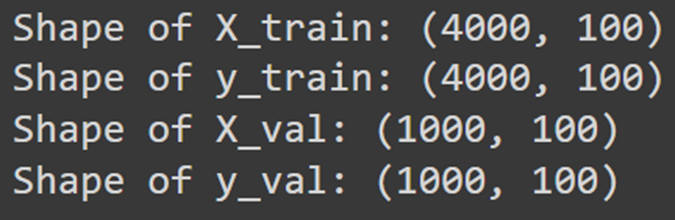
On this step, we’re splitting the preprocessed information into coaching and validation units.
Step 6: Constructing The LSTM Mannequin
from keras.fashions import Sequential
from keras.layers import Dense, LSTM, Embedding, RepeatVectormannequin = Sequential()
mannequin.add(Embedding(input_dim=vocab_size_eng, output_dim=128,input_shape=(eng_length,), mask_zero=True))
mannequin.add(LSTM(models=512))
mannequin.add(RepeatVector(n=hin_length))
mannequin.add(LSTM(models=512, return_sequences=True))
mannequin.add(Dense(models=vocab_size_hin, activation='softmax'))
This step includes constructing the LSTM sequence-to-sequence mannequin for English to Hindi translation. Let’s break down the layers added to the mannequin:
The primary layer is an embedding layer (Embedding) which maps every phrase index to a dense vector illustration. It takes as enter the vocabulary dimension for English (vocab_size_eng), the output dimensionality (output_dim=128), and the enter form specified by the utmost sequence size for English (input_shape=(eng_length,)). Moreover, mask_zero=True is about to disregard padded zeros.
Subsequent, we add an LSTM layer (LSTM) with 512 models, which processes the embedded sequences.
The RepeatVector layer repeats the output of the LSTM layer for hin_length occasions, getting ready it to be fed into the next LSTM layer.
Then, we add one other LSTM layer with 512 models, set to return sequences (return_sequences=True), which is essential for sequence-to-sequence fashions.
Lastly, we add a dense layer (Dense) with a softmax activation perform to foretell the chance distribution over the Hindi vocabulary for every time step.
Printing the Mannequin Abstract
mannequin.abstract()
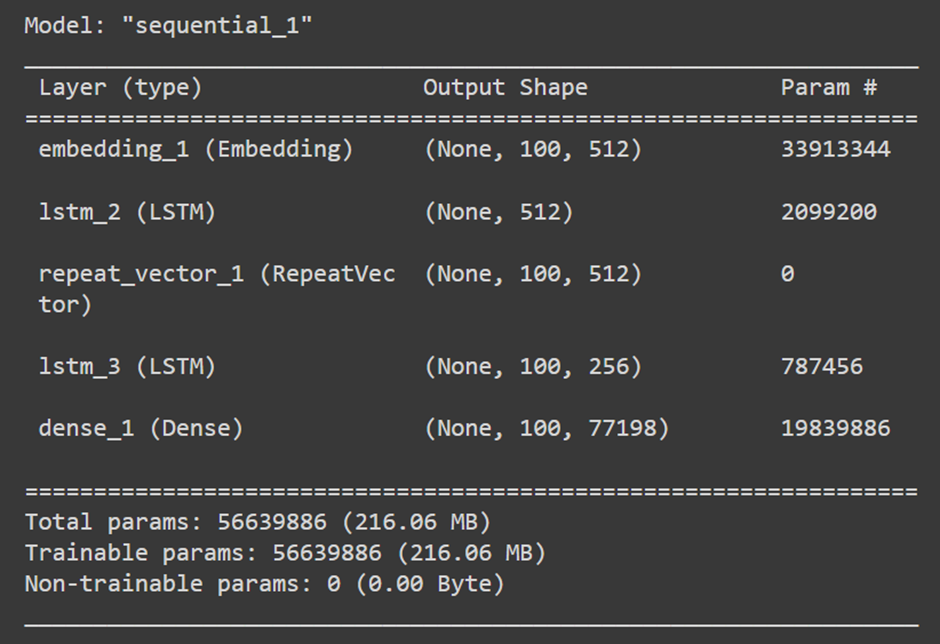
Step 7: Compiling and Coaching the Mannequin
from tensorflow.keras.optimizers import RMSprop# Outline optimizer
rms = RMSprop(learning_rate=0.001)
# Compile the mannequin
mannequin.compile(optimizer=rms, loss="sparse_categorical_crossentropy", metrics=['accuracy'])
# Prepare the mannequin
historical past = mannequin.match(X_train, y_train, validation_data=(X_val, y_val), epochs=10, batch_size=32)
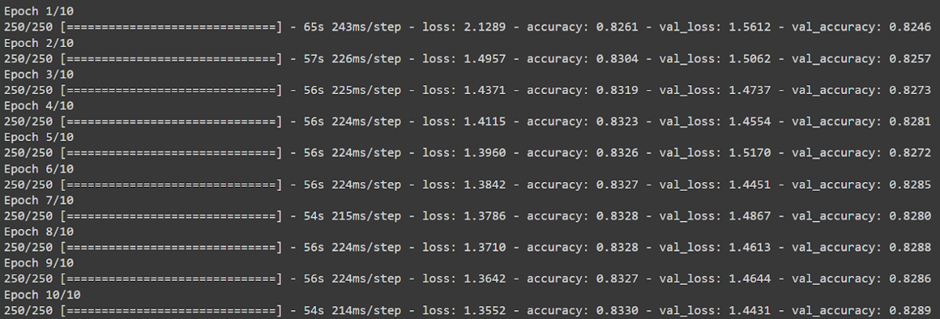
This step compiles the LSTM mannequin with rms optimizer, sparse_categorical_crossentropy loss perform, and accuracy metrics. Then, it trains the mannequin on the supplied information for 10 epochs, utilizing a batch dimension of 32. The coaching course of yields a historical past object capturing coaching metrics over epochs.
Step 8: Plotting Coaching and Validation Loss
import matplotlib.pyplot as plt# Get the coaching historical past
loss = historical past.historical past['loss']
val_loss = historical past.historical past['val_loss']
epochs = vary(1, len(loss) + 1)
# Plot loss and validation loss with customized colours
plt.plot(epochs, loss, 'r', label="Coaching Loss") # Pink coloration for coaching loss
plt.plot(epochs, val_loss, 'g', label="Validation Loss") # Inexperienced coloration for validation loss
plt.title('Coaching and Validation Loss')
plt.xlabel('Epochs')
plt.ylabel('Loss')
plt.legend()
plt.present()
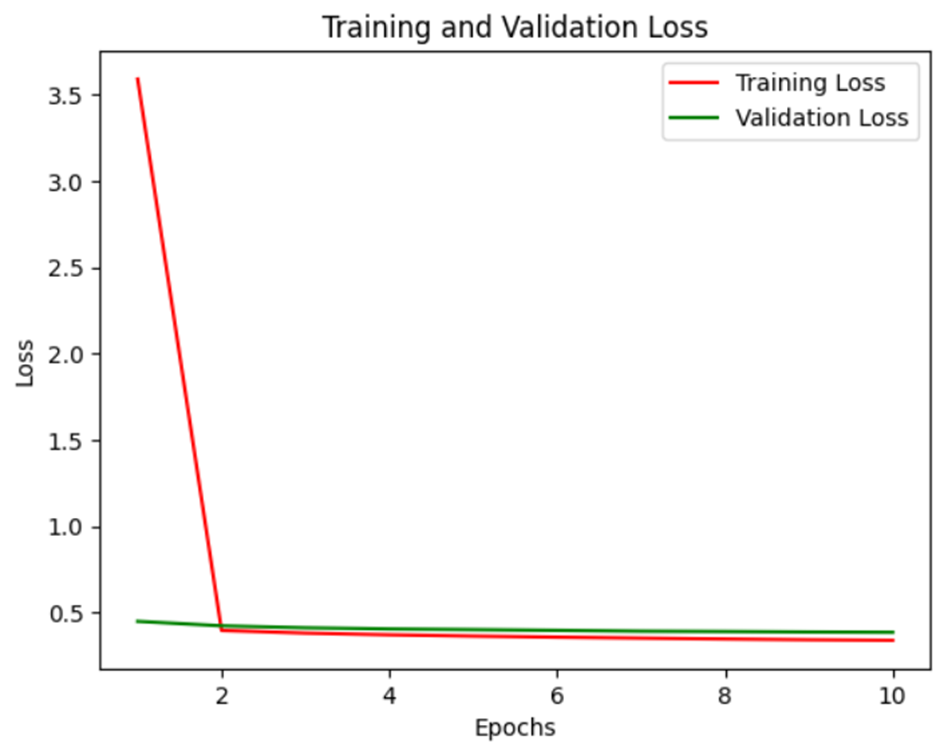
This step includes plotting the coaching and validation loss over epochs to visualise the mannequin’s studying progress and potential overfitting.
Conclusion
This information navigates the creation of an LSTM sequence-to-sequence mannequin for English-to-Hindi language translation. It begins with an outline of RNNs and LSTMs, emphasizing their means to deal with sequential information successfully. The target is to translate English sentences into Hindi utilizing this mannequin.
Steps embrace information loading from Hugging Face, preprocessing to take away punctuation and deal with empty rows, and tokenization with sequence padding for uniform size. The LSTM mannequin is meticulously constructed with embedding, LSTM, RepeatVector, and dense layers. Coaching includes compiling the mannequin with an optimizer, loss perform, and metrics, adopted by becoming it to the dataset over epochs.
Visualizing coaching and validation loss provides insights into the mannequin’s studying progress. Finally, this information empowers customers with the abilities to assemble LSTM fashions for language translation duties, offering a basis for additional exploration in NLP.
Continuously Requested Questions
A. An LSTM (Lengthy Brief-Time period Reminiscence) sequence-to-sequence mannequin is a kind of neural community structure designed to translate sequences of information from one language to a different. It makes use of LSTM models to seize each short-term and long-term dependencies inside sequential information successfully.
A. The LSTM mannequin processes enter sequences, sometimes in English, and generates corresponding output sequences, normally in one other language like Hindi. It does so by studying to encode the enter sequence right into a fixed-size vector illustration after which decoding this illustration into the output sequence.
A. Preprocessing steps embrace eradicating punctuation, dealing with empty rows, tokenizing the textual content into sequences of integers, and padding the sequences to make sure uniform size.
A. Frequent analysis metrics embrace coaching and validation loss, which measure the discrepancy between predicted and precise sequences throughout coaching. Moreover, metrics like BLEU rating can be utilized to guage the mannequin’s efficiency.
A. Efficiency will be improved by experimenting with totally different mannequin architectures, adjusting hyperparameters reminiscent of studying fee and batch dimension, growing the scale of the coaching dataset, and using methods like consideration mechanisms to deal with related components of the enter sequence throughout translation.
A. Sure, the LSTM mannequin will be tailored to translate between pairs of languages apart from English and Hindi. By coaching the mannequin on datasets containing sequences in several languages, it could possibly study to carry out translation duties for these language pairs as nicely.