Introduction
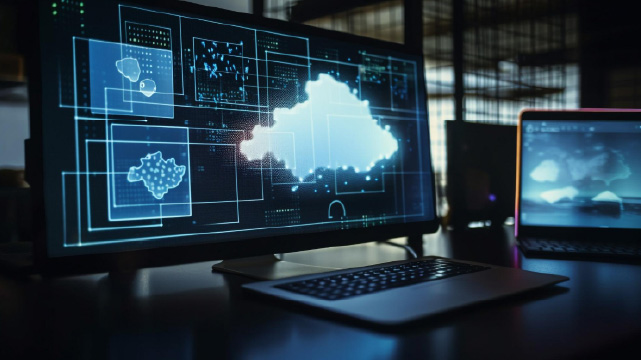
Pandas is a strong knowledge manipulation library in Python that gives varied functionalities to work with structured knowledge. One widespread activity in knowledge evaluation is so as to add a brand new column to an current DataFrame in Pandas. This text will discover completely different strategies to perform this activity and supply examples for instance their utilization.
Why Add a New Column to a DataFrame?
Including a brand new column to a DataFrame permits us to incorporate extra info or carry out calculations based mostly on current knowledge. It enhances the flexibleness and performance of the DataFrame, enabling us to research and manipulate the information extra successfully.
Strategies for Including a New Column
A number of strategies can be found in Pandas so as to add a brand new column to an current DataFrame. Let’s discover every of them:
Utilizing the Bracket Notation
The bracket notation is an easy and intuitive manner so as to add a brand new column to a DataFrame. We are able to assign values to the brand new column by specifying the identify inside sq. brackets and assigning it to an inventory or array of values.
Code:
import pandas as pd
df = pd.DataFrame({'Title': ['John', 'Alice', 'Bob'],
'Age': [25, 30, 35]})
df['Gender'] = ['Male', 'Female', 'Male']
Utilizing the `assign()` Technique
The `assign()` methodology permits us so as to add a brand new column to a DataFrame by specifying the column identify and its corresponding values. It returns a brand new DataFrame with the added column, leaving the unique DataFrame unchanged.
Code:
import pandas as pd
df = pd.DataFrame({'Title': ['John', 'Alice', 'Bob'],
'Age': [25, 30, 35]})
df_new = df.assign(Gender=['Male', 'Female', 'Male'])
Utilizing the `insert()` Technique
The `insert()` methodology allows us so as to add a brand new column at a particular place throughout the DataFrame. We should present the index of the specified place, column identify, and values.
Code:
import pandas as pd
df = pd.DataFrame({'Title': ['John', 'Alice', 'Bob'],
'Age': [25, 30, 35]})
df.insert(1, 'Gender', ['Male', 'Female', 'Male'])
Utilizing the `concat()` Operate
The `concat()` operate permits us to concatenate two or extra DataFrames alongside a specific axis. We are able to use this operate so as to add a brand new column from one other DataFrame to an current DataFrame.
Code:
import pandas as pd
df1 = pd.DataFrame({'Title': ['John', 'Alice', 'Bob'],
'Age': [25, 30, 35]})
df2 = pd.DataFrame({'Gender': ['Male', 'Female', 'Male']})
df = pd.concat([df1, df2], axis=1)
Examples of Including a New Column
Let’s discover some examples for instance the way to add a brand new column to a DataFrame.
Including a Column with Fixed Values
Utilizing the above talked about strategies, we will add a brand new column with fixed values to a DataFrame. That is helpful once we need to embrace extra info that’s the identical for all rows.
Code:
import pandas as pd
df = pd.DataFrame({'Title': ['John', 'Alice', 'Bob'],
'Age': [25, 30, 35]})
df['Nationality'] = 'USA'
Including a Column with Calculated Values
We are able to add a brand new column with calculated values based mostly on current columns. This enables us to carry out computations and derive insights from the information.
Code:
import pandas as pd
df = pd.DataFrame({'Title': ['John', 'Alice', 'Bob'],
'Age': [25, 30, 35]})
df['Birth Year'] = 2024 - df['Age']
Including a Column with Conditional Logic
We are able to add a brand new column based mostly on conditional logic utilized to current columns. This allows us to categorize or flag sure rows based mostly on particular circumstances.
Code:
import pandas as pd
df = pd.DataFrame({'Title': ['John', 'Alice', 'Bob'],
'Age': [25, 17, 35]})
df['Is Adult'] = df['Age'] >= 18
Including a Column with Knowledge from One other DataFrame
We are able to add a brand new column to a DataFrame by extracting knowledge from one other DataFrame. That is helpful once we need to mix info from completely different sources.
Code:
import pandas as pd
df1 = pd.DataFrame({'Title': ['John', 'Alice', 'Bob'],
'Age': [25, 30, 35]})
df2 = pd.DataFrame({'Gender': ['Male', 'Female', 'Male']})
df1['Gender'] = df2['Gender']
Greatest Practices for Including Columns
When including columns to a DataFrame in Pandas, it’s important to observe sure greatest practices to make sure consistency and effectivity. Listed here are some suggestions:
- Naming Conventions for New Columns: Select descriptive and significant names for brand new columns that precisely signify the data they comprise. This improves the readability and understandability of the DataFrame.
- Dealing with Lacking or Null Values: Contemplate how lacking or null values needs to be dealt with when including a brand new column. Determine whether or not to assign default values, drop rows with lacking values, or use applicable knowledge imputation strategies.
- Contemplating Efficiency and Reminiscence Utilization: Be aware of the efficiency and reminiscence implications when including columns to massive DataFrames. Keep away from pointless computations or operations considerably impacting processing time and reminiscence consumption.
Conclusion
Including a brand new column to an current DataFrame in Pandas is a elementary operation in knowledge evaluation. We explored varied strategies, together with bracket notation, dot notation, `assign()` methodology, `insert()` methodology, and `concat()` operate. We additionally offered examples to exhibit their utilization in numerous situations. By following greatest practices and contemplating efficiency issues, we will successfully improve the performance and insights derived from the DataFrame.